802. Find Eventual Safe States LeetCode Solution
In this guide, you will get 802. Find Eventual Safe States LeetCode Solution with the best time and space complexity. The solution to Find Eventual Safe States problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Find Eventual Safe States solution in C++
- Find Eventual Safe States solution in Java
- Find Eventual Safe States solution in Python
- Additional Resources
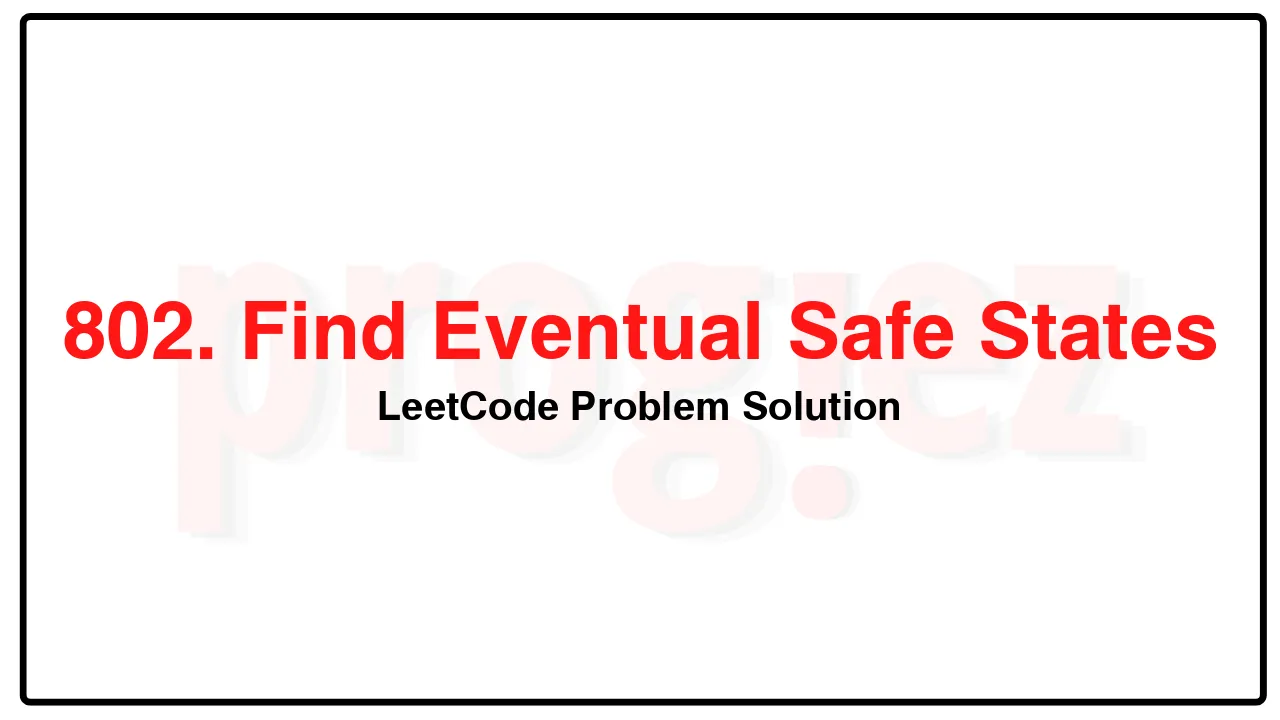
Problem Statement of Find Eventual Safe States
There is a directed graph of n nodes with each node labeled from 0 to n – 1. The graph is represented by a 0-indexed 2D integer array graph where graph[i] is an integer array of nodes adjacent to node i, meaning there is an edge from node i to each node in graph[i].
A node is a terminal node if there are no outgoing edges. A node is a safe node if every possible path starting from that node leads to a terminal node (or another safe node).
Return an array containing all the safe nodes of the graph. The answer should be sorted in ascending order.
Example 1:
Input: graph = [[1,2],[2,3],[5],[0],[5],[],[]]
Output: [2,4,5,6]
Explanation: The given graph is shown above.
Nodes 5 and 6 are terminal nodes as there are no outgoing edges from either of them.
Every path starting at nodes 2, 4, 5, and 6 all lead to either node 5 or 6.
Example 2:
Input: graph = [[1,2,3,4],[1,2],[3,4],[0,4],[]]
Output: [4]
Explanation:
Only node 4 is a terminal node, and every path starting at node 4 leads to node 4.
Constraints:
n == graph.length
1 <= n <= 104
0 <= graph[i].length <= n
0 <= graph[i][j] <= n – 1
graph[i] is sorted in a strictly increasing order.
The graph may contain self-loops.
The number of edges in the graph will be in the range [1, 4 * 104].
Complexity Analysis
- Time Complexity: O(|V| + |E|)
- Space Complexity: O(|V| + |E|)
802. Find Eventual Safe States LeetCode Solution in C++
enum class State { kInit, kVisiting, kVisited };
class Solution {
public:
vector<int> eventualSafeNodes(vector<vector<int>>& graph) {
vector<int> ans;
vector<State> states(graph.size());
for (int i = 0; i < graph.size(); ++i)
if (!hasCycle(graph, i, states))
ans.push_back(i);
return ans;
}
private:
bool hasCycle(const vector<vector<int>>& graph, int u,
vector<State>& states) {
if (states[u] == State::kVisiting)
return true;
if (states[u] == State::kVisited)
return false;
states[u] = State::kVisiting;
for (const int v : graph[u])
if (hasCycle(graph, v, states))
return true;
states[u] = State::kVisited;
return false;
}
};
/* code provided by PROGIEZ */
802. Find Eventual Safe States LeetCode Solution in Java
enum State { kInit, kVisiting, kVisited }
class Solution {
public List<Integer> eventualSafeNodes(int[][] graph) {
List<Integer> ans = new ArrayList<>();
State[] states = new State[graph.length];
for (int i = 0; i < graph.length; ++i)
if (!hasCycle(graph, i, states))
ans.add(i);
return ans;
}
private boolean hasCycle(int[][] graph, int u, State[] states) {
if (states[u] == State.kVisiting)
return true;
if (states[u] == State.kVisited)
return false;
states[u] = State.kVisiting;
for (final int v : graph[u])
if (hasCycle(graph, v, states))
return true;
states[u] = State.kVisited;
return false;
}
}
// code provided by PROGIEZ
802. Find Eventual Safe States LeetCode Solution in Python
from enum import Enum
class State(Enum):
kInit = 0
kVisiting = 1
kVisited = 2
class Solution:
def eventualSafeNodes(self, graph: list[list[int]]) -> list[int]:
states = [State.kInit] * len(graph)
def hasCycle(u: int) -> bool:
if states[u] == State.kVisiting:
return True
if states[u] == State.kVisited:
return False
states[u] = State.kVisiting
if any(hasCycle(v) for v in graph[u]):
return True
states[u] = State.kVisited
return False
return [i for i in range(len(graph)) if not hasCycle(i)]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.