188. Best Time to Buy and Sell Stock IV LeetCode Solution
In this guide, you will get 188. Best Time to Buy and Sell Stock IV LeetCode Solution with the best time and space complexity. The solution to Best Time to Buy and Sell Stock IV problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Best Time to Buy and Sell Stock IV solution in C++
- Best Time to Buy and Sell Stock IV solution in Java
- Best Time to Buy and Sell Stock IV solution in Python
- Additional Resources
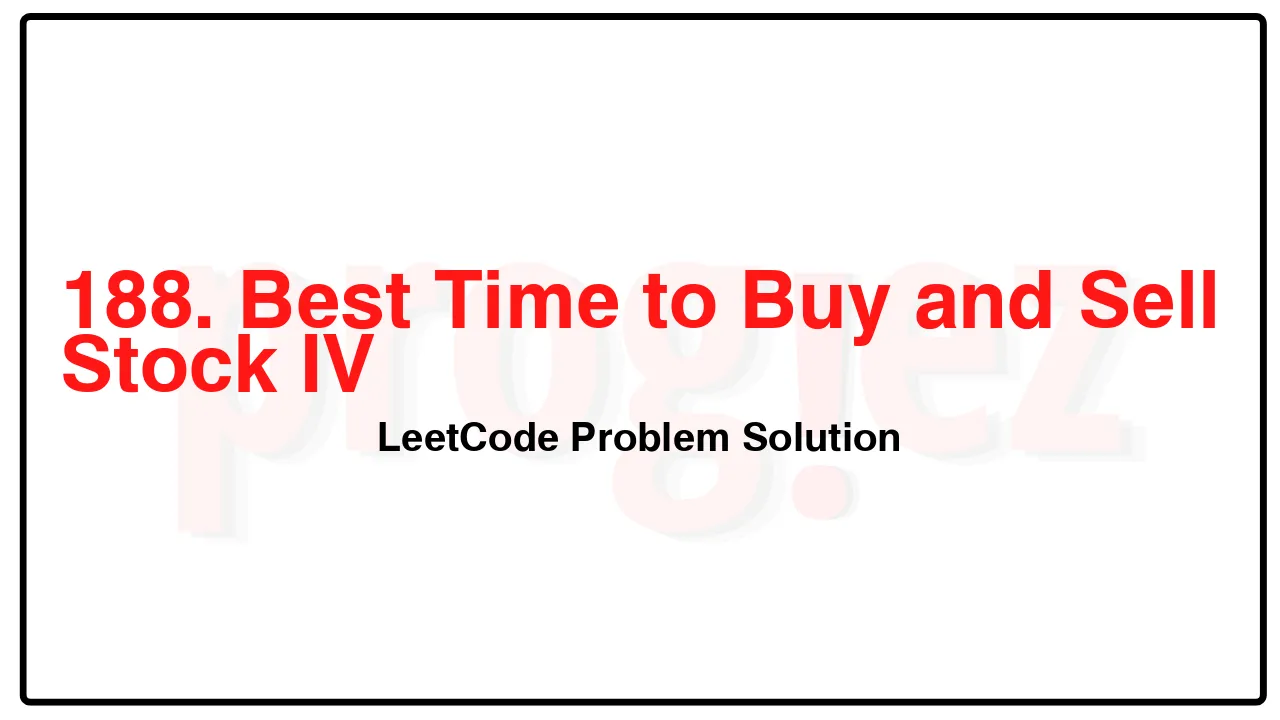
Problem Statement of Best Time to Buy and Sell Stock IV
You are given an integer array prices where prices[i] is the price of a given stock on the ith day, and an integer k.
Find the maximum profit you can achieve. You may complete at most k transactions: i.e. you may buy at most k times and sell at most k times.
Note: You may not engage in multiple transactions simultaneously (i.e., you must sell the stock before you buy again).
Example 1:
Input: k = 2, prices = [2,4,1]
Output: 2
Explanation: Buy on day 1 (price = 2) and sell on day 2 (price = 4), profit = 4-2 = 2.
Example 2:
Input: k = 2, prices = [3,2,6,5,0,3]
Output: 7
Explanation: Buy on day 2 (price = 2) and sell on day 3 (price = 6), profit = 6-2 = 4. Then buy on day 5 (price = 0) and sell on day 6 (price = 3), profit = 3-0 = 3.
Constraints:
1 <= k <= 100
1 <= prices.length <= 1000
0 <= prices[i] <= 1000
Complexity Analysis
- Time Complexity: O(nk)
- Space Complexity: O(k)
188. Best Time to Buy and Sell Stock IV LeetCode Solution in C++
class Solution {
public:
int maxProfit(int k, vector<int>& prices) {
if (k >= prices.size() / 2) {
int sell = 0;
int hold = INT_MIN;
for (const int price : prices) {
sell = max(sell, hold + price);
hold = max(hold, sell - price);
}
return sell;
}
vector<int> sell(k + 1);
vector<int> hold(k + 1, INT_MIN);
for (const int price : prices)
for (int i = k; i > 0; --i) {
sell[i] = max(sell[i], hold[i] + price);
hold[i] = max(hold[i], sell[i - 1] - price);
}
return sell[k];
}
};
/* code provided by PROGIEZ */
188. Best Time to Buy and Sell Stock IV LeetCode Solution in Java
class Solution {
public int maxProfit(int k, int[] prices) {
if (k >= prices.length / 2) {
int sell = 0;
int hold = Integer.MIN_VALUE;
for (final int price : prices) {
sell = Math.max(sell, hold + price);
hold = Math.max(hold, sell - price);
}
return sell;
}
int[] sell = new int[k + 1];
int[] hold = new int[k + 1];
Arrays.fill(hold, Integer.MIN_VALUE);
for (final int price : prices)
for (int i = k; i > 0; --i) {
sell[i] = Math.max(sell[i], hold[i] + price);
hold[i] = Math.max(hold[i], sell[i - 1] - price);
}
return sell[k];
}
}
// code provided by PROGIEZ
188. Best Time to Buy and Sell Stock IV LeetCode Solution in Python
class Solution:
def maxProfit(self, k: int, prices: list[int]) -> int:
if k >= len(prices) // 2:
sell = 0
hold = -math.inf
for price in prices:
sell = max(sell, hold + price)
hold = max(hold, sell - price)
return sell
sell = [0] * (k + 1)
hold = [-math.inf] * (k + 1)
for price in prices:
for i in range(k, 0, -1):
sell[i] = max(sell[i], hold[i] + price)
hold[i] = max(hold[i], sell[i - 1] - price)
return sell[k]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.