1200. Minimum Absolute Difference LeetCode Solution
In this guide, you will get 1200. Minimum Absolute Difference LeetCode Solution with the best time and space complexity. The solution to Minimum Absolute Difference problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Absolute Difference solution in C++
- Minimum Absolute Difference solution in Java
- Minimum Absolute Difference solution in Python
- Additional Resources
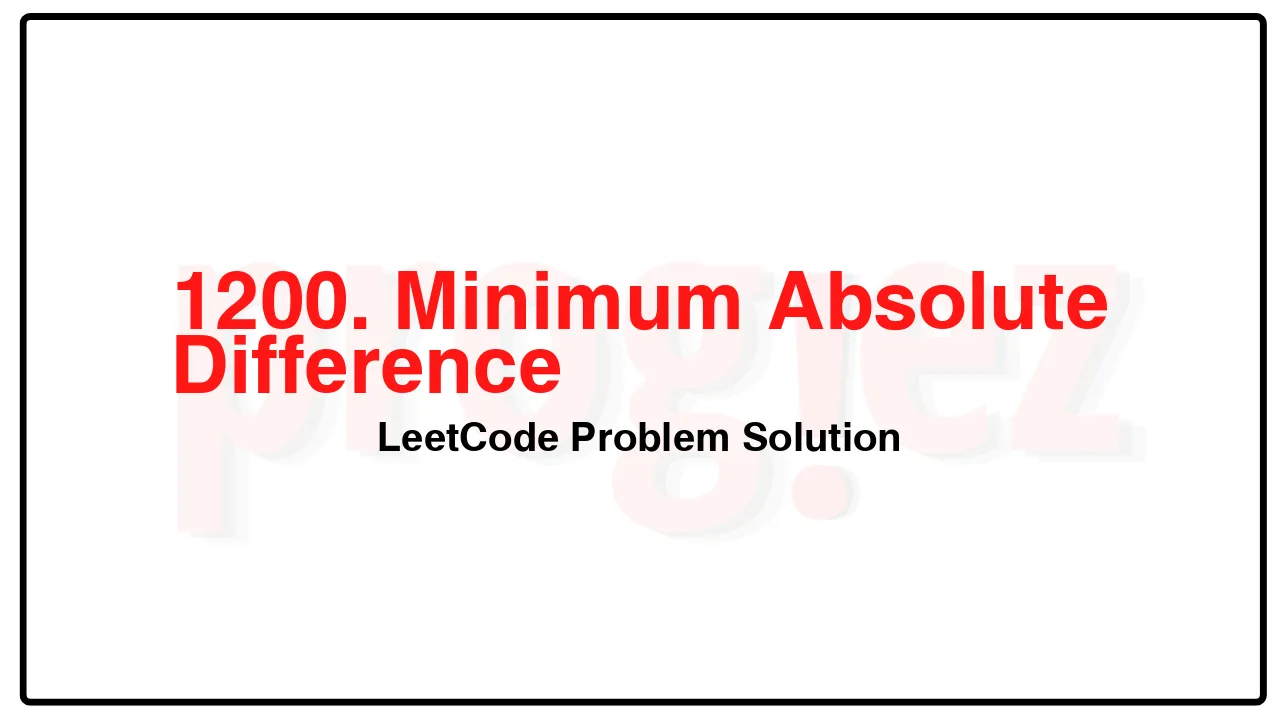
Problem Statement of Minimum Absolute Difference
Given an array of distinct integers arr, find all pairs of elements with the minimum absolute difference of any two elements.
Return a list of pairs in ascending order(with respect to pairs), each pair [a, b] follows
a, b are from arr
a < b
b – a equals to the minimum absolute difference of any two elements in arr
Example 1:
Input: arr = [4,2,1,3]
Output: [[1,2],[2,3],[3,4]]
Explanation: The minimum absolute difference is 1. List all pairs with difference equal to 1 in ascending order.
Example 2:
Input: arr = [1,3,6,10,15]
Output: [[1,3]]
Example 3:
Input: arr = [3,8,-10,23,19,-4,-14,27]
Output: [[-14,-10],[19,23],[23,27]]
Constraints:
2 <= arr.length <= 105
-106 <= arr[i] <= 106
Complexity Analysis
- Time Complexity: O(\texttt{sort})
- Space Complexity: O(n)
1200. Minimum Absolute Difference LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> minimumAbsDifference(vector<int>& arr) {
vector<vector<int>> ans;
int mn = INT_MAX;
ranges::sort(arr);
for (int i = 1; i < arr.size(); ++i) {
const int diff = arr[i] - arr[i - 1];
if (diff < mn) {
mn = diff;
ans.clear();
}
if (diff == mn)
ans.push_back({arr[i - 1], arr[i]});
}
return ans;
}
};
/* code provided by PROGIEZ */
1200. Minimum Absolute Difference LeetCode Solution in Java
class Solution {
public List<List<Integer>> minimumAbsDifference(int[] arr) {
List<List<Integer>> ans = new ArrayList<>();
int mn = Integer.MAX_VALUE;
Arrays.sort(arr);
for (int i = 1; i < arr.length; ++i) {
final int diff = arr[i] - arr[i - 1];
if (diff < mn) {
mn = diff;
ans.clear();
}
if (diff == mn)
ans.add(Arrays.asList(arr[i - 1], arr[i]));
}
return ans;
}
}
// code provided by PROGIEZ
1200. Minimum Absolute Difference LeetCode Solution in Python
class Solution:
def minimumAbsDifference(self, arr: list[int]) -> list[list[int]]:
ans = []
mn = math.inf
arr.sort()
for a, b in itertools.pairwise(arr):
diff = b - a
if diff < mn:
mn = diff
ans = []
if diff == mn:
ans.append([a, b])
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.