1131. Maximum of Absolute Value Expression LeetCode Solution
In this guide, you will get 1131. Maximum of Absolute Value Expression LeetCode Solution with the best time and space complexity. The solution to Maximum of Absolute Value Expression problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximum of Absolute Value Expression solution in C++
- Maximum of Absolute Value Expression solution in Java
- Maximum of Absolute Value Expression solution in Python
- Additional Resources
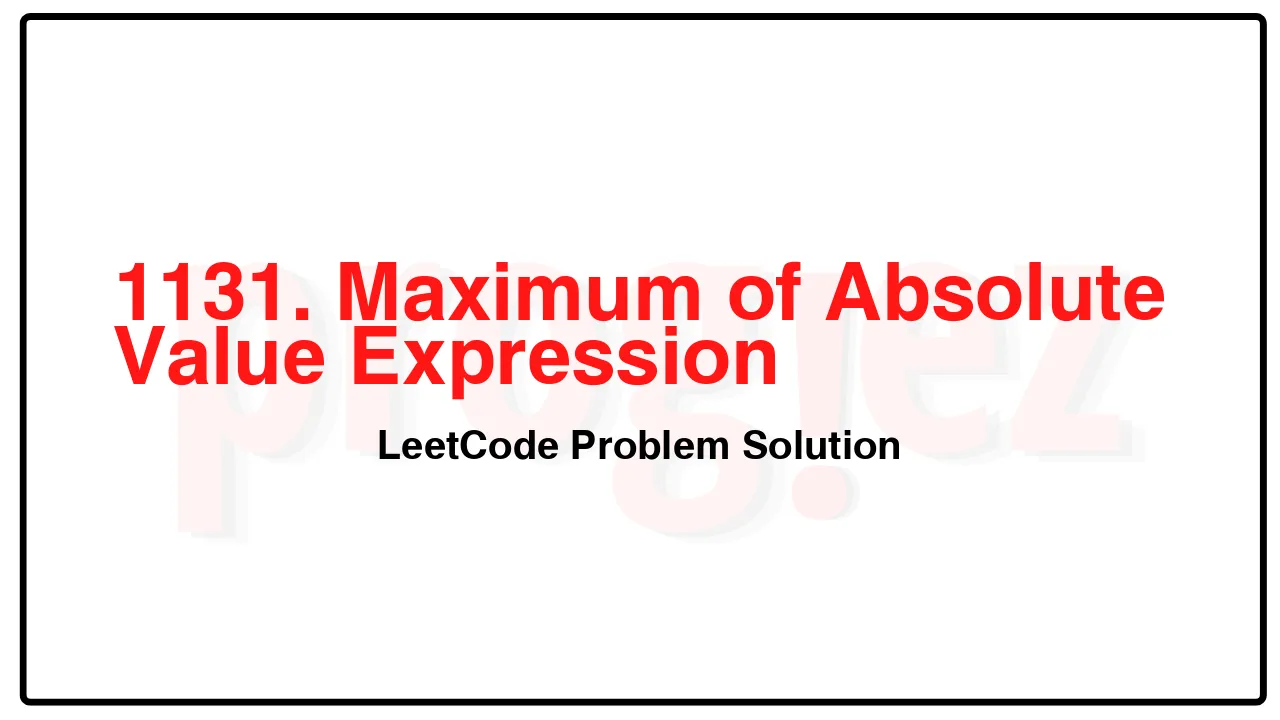
Problem Statement of Maximum of Absolute Value Expression
Given two arrays of integers with equal lengths, return the maximum value of:
|arr1[i] – arr1[j]| + |arr2[i] – arr2[j]| + |i – j|
where the maximum is taken over all 0 <= i, j < arr1.length.
Example 1:
Input: arr1 = [1,2,3,4], arr2 = [-1,4,5,6]
Output: 13
Example 2:
Input: arr1 = [1,-2,-5,0,10], arr2 = [0,-2,-1,-7,-4]
Output: 20
Constraints:
2 <= arr1.length == arr2.length <= 40000
-10^6 <= arr1[i], arr2[i] <= 10^6
Complexity Analysis
- Time Complexity:
- Space Complexity:
1131. Maximum of Absolute Value Expression LeetCode Solution in C++
class Solution {
public:
int maxAbsValExpr(vector<int>& arr1, vector<int>& arr2) {
const int n = arr1.size();
vector<int> a(n);
vector<int> b(n);
vector<int> c(n);
vector<int> d(n);
for (int i = 0; i < n; ++i) {
a[i] = arr1[i] + arr2[i] + i;
b[i] = arr1[i] + arr2[i] - i;
c[i] = arr1[i] - arr2[i] + i;
d[i] = arr1[i] - arr2[i] - i;
}
return max(max(diff(a), diff(b)), max(diff(c), diff(d)));
}
private:
int diff(vector<int>& nums) {
return ranges::max(nums) - ranges::min(nums);
}
};
/* code provided by PROGIEZ */
1131. Maximum of Absolute Value Expression LeetCode Solution in Java
class Solution {
public int maxAbsValExpr(int[] arr1, int[] arr2) {
final int n = arr1.length;
int[] a = new int[n];
int[] b = new int[n];
int[] c = new int[n];
int[] d = new int[n];
for (int i = 0; i < n; ++i) {
a[i] = arr1[i] + arr2[i] + i;
b[i] = arr1[i] + arr2[i] - i;
c[i] = arr1[i] - arr2[i] + i;
d[i] = arr1[i] - arr2[i] - i;
}
return Math.max(Math.max(diff(a), diff(b)), Math.max(diff(c), diff(d)));
}
private int diff(int[] nums) {
final int mn = Arrays.stream(nums).min().getAsInt();
final int mx = Arrays.stream(nums).max().getAsInt();
return mx - mn;
}
}
// code provided by PROGIEZ
1131. Maximum of Absolute Value Expression LeetCode Solution in Python
class Solution:
def maxAbsValExpr(self, arr1: list[int], arr2: list[int]) -> int:
n = len(arr1)
a = [arr1[i] + arr2[i] + i for i in range(n)]
b = [arr1[i] + arr2[i] - i for i in range(n)]
c = [arr1[i] - arr2[i] + i for i in range(n)]
d = [arr1[i] - arr2[i] - i for i in range(n)]
return max(map(lambda x: max(x) - min(x), (a, b, c, d)))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.