1185. Day of the Week LeetCode Solution
In this guide, you will get 1185. Day of the Week LeetCode Solution with the best time and space complexity. The solution to Day of the Week problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Day of the Week solution in C++
- Day of the Week solution in Java
- Day of the Week solution in Python
- Additional Resources
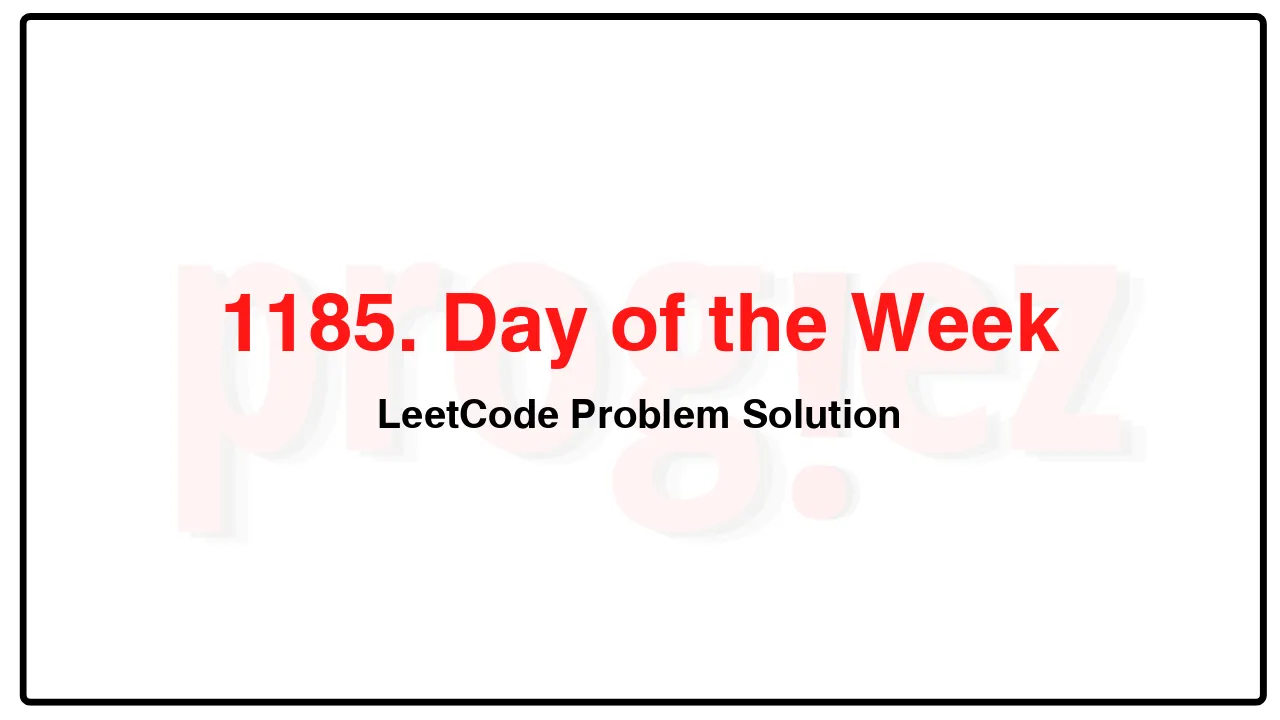
Problem Statement of Day of the Week
Given a date, return the corresponding day of the week for that date.
The input is given as three integers representing the day, month and year respectively.
Return the answer as one of the following values {“Sunday”, “Monday”, “Tuesday”, “Wednesday”, “Thursday”, “Friday”, “Saturday”}.
Example 1:
Input: day = 31, month = 8, year = 2019
Output: “Saturday”
Example 2:
Input: day = 18, month = 7, year = 1999
Output: “Sunday”
Example 3:
Input: day = 15, month = 8, year = 1993
Output: “Sunday”
Constraints:
The given dates are valid dates between the years 1971 and 2100.
Complexity Analysis
- Time Complexity:
- Space Complexity:
1185. Day of the Week LeetCode Solution in C++
class Solution {
public:
string dayOfTheWeek(int day, int month, int year) {
vector<string> week = {"Sunday", "Monday", "Tuesday", "Wednesday",
"Thursday", "Friday", "Saturday"};
vector<int> days = {
, isLeapYear(year) ? 29 : 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int count = 0;
for (int i = 1971; i < year; ++i)
count += i % 4 == 0 ? 366 : 365;
for (int i = 0; i < month - 1; ++i)
count += days[i];
count += day;
return week[(count + 4) % 7];
}
private:
bool isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
};
/* code provided by PROGIEZ */
1185. Day of the Week LeetCode Solution in Java
class Solution {
public String dayOfTheWeek(int day, int month, int year) {
String[] week = {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"};
int[] days = {31, isLeapYear(year) ? 29 : 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int count = 0;
for (int i = 1971; i < year; ++i)
count += i % 4 == 0 ? 366 : 365;
for (int i = 0; i < month - 1; ++i)
count += days[i];
count += day;
return week[(count + 4) % 7];
}
private boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
}
// code provided by PROGIEZ
1185. Day of the Week LeetCode Solution in Python
class Solution:
def dayOfTheWeek(self, day: int, month: int, year: int) -> str:
def isLeapYear(year: int) -> bool:
return (year % 4 == 0 and year % 100 != 0) or year % 400 == 0
week = ["Sunday", "Monday", "Tuesday",
"Wednesday", "Thursday", "Friday", "Saturday"]
days = [31, 29 if isLeapYear(
year) else 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
count = 0
for i in range(1971, year):
count += 366 if i % 4 == 0 else 365
for i in range(month - 1):
count += days[i]
count += day
return week[(count + 4) % 7]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.