815. Bus Routes LeetCode Solution
In this guide, you will get 815. Bus Routes LeetCode Solution with the best time and space complexity. The solution to Bus Routes problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Bus Routes solution in C++
- Bus Routes solution in Java
- Bus Routes solution in Python
- Additional Resources
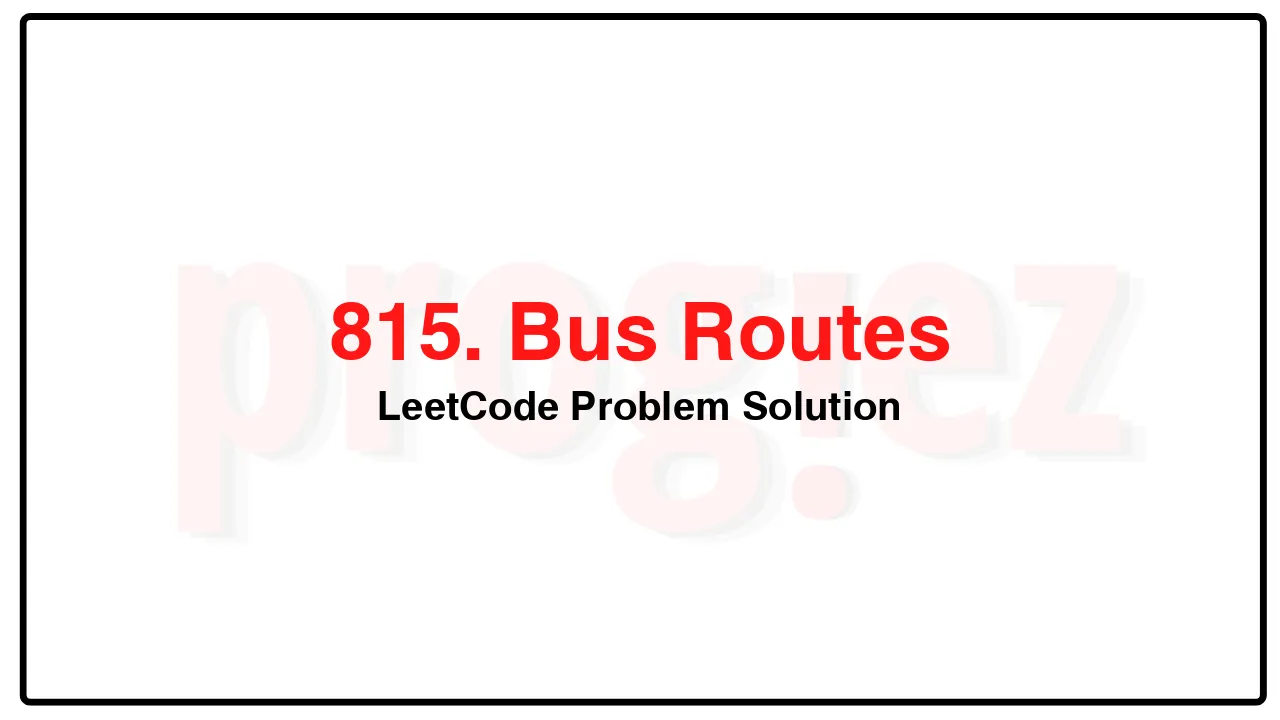
Problem Statement of Bus Routes
You are given an array routes representing bus routes where routes[i] is a bus route that the ith bus repeats forever.
For example, if routes[0] = [1, 5, 7], this means that the 0th bus travels in the sequence 1 -> 5 -> 7 -> 1 -> 5 -> 7 -> 1 -> … forever.
You will start at the bus stop source (You are not on any bus initially), and you want to go to the bus stop target. You can travel between bus stops by buses only.
Return the least number of buses you must take to travel from source to target. Return -1 if it is not possible.
Example 1:
Input: routes = [[1,2,7],[3,6,7]], source = 1, target = 6
Output: 2
Explanation: The best strategy is take the first bus to the bus stop 7, then take the second bus to the bus stop 6.
Example 2:
Input: routes = [[7,12],[4,5,15],[6],[15,19],[9,12,13]], source = 15, target = 12
Output: -1
Constraints:
1 <= routes.length <= 500.
1 <= routes[i].length <= 105
All the values of routes[i] are unique.
sum(routes[i].length) <= 105
0 <= routes[i][j] < 106
0 <= source, target < 106
Complexity Analysis
- Time Complexity: O(n^2), where n = |\texttt{routes}|
- Space Complexity: O(n^2) + \Sigma |\texttt{routes[i]}|
815. Bus Routes LeetCode Solution in C++
class Solution {
public:
int numBusesToDestination(vector<vector<int>>& routes, int source,
int target) {
if (source == target)
return 0;
unordered_map<int, vector<int>> graph; // {route: [buses]}
unordered_set<int> usedBuses;
for (int i = 0; i < routes.size(); ++i)
for (const int route : routes[i])
graph[route].push_back(i);
queue<int> q{{source}};
for (int step = 1; !q.empty(); ++step)
for (int sz = q.size(); sz > 0; --sz) {
const int route = q.front();
q.pop();
for (const int bus : graph[route])
if (usedBuses.insert(bus).second)
for (const int nextRoute : routes[bus]) {
if (nextRoute == target)
return step;
q.push(nextRoute);
}
}
return -1;
}
};
/* code provided by PROGIEZ */
815. Bus Routes LeetCode Solution in Java
class Solution {
public int numBusesToDestination(int[][] routes, int source, int target) {
if (source == target)
return 0;
Map<Integer, List<Integer>> graph = new HashMap<>(); // {route: [buses]}
Set<Integer> usedBuses = new HashSet<>();
for (int i = 0; i < routes.length; ++i)
for (final int route : routes[i]) {
graph.putIfAbsent(route, new ArrayList<>());
graph.get(route).add(i);
}
Queue<Integer> q = new ArrayDeque<>(List.of(source));
for (int step = 1; !q.isEmpty(); ++step)
for (int sz = q.size(); sz > 0; --sz) {
for (final int bus : graph.getOrDefault(q.poll(), new ArrayList<>()))
if (usedBuses.add(bus))
for (final int nextRoute : routes[bus]) {
if (nextRoute == target)
return step;
q.offer(nextRoute);
}
}
return -1;
}
}
// code provided by PROGIEZ
815. Bus Routes LeetCode Solution in Python
class Solution:
def numBusesToDestination(
self,
routes: list[list[int]],
source: int,
target: int,
) -> int:
if source == target:
return 0
graph = collections.defaultdict(list)
usedBuses = set()
for i in range(len(routes)):
for route in routes[i]:
graph[route].append(i)
q = collections.deque([source])
step = 1
while q:
for _ in range(len(q)):
for bus in graph[q.popleft()]:
if bus in usedBuses:
continue
usedBuses.add(bus)
for nextRoute in routes[bus]:
if nextRoute == target:
return step
q.append(nextRoute)
step += 1
return -1
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.