1154. Day of the Year LeetCode Solution
In this guide, you will get 1154. Day of the Year LeetCode Solution with the best time and space complexity. The solution to Day of the Year problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Day of the Year solution in C++
- Day of the Year solution in Java
- Day of the Year solution in Python
- Additional Resources
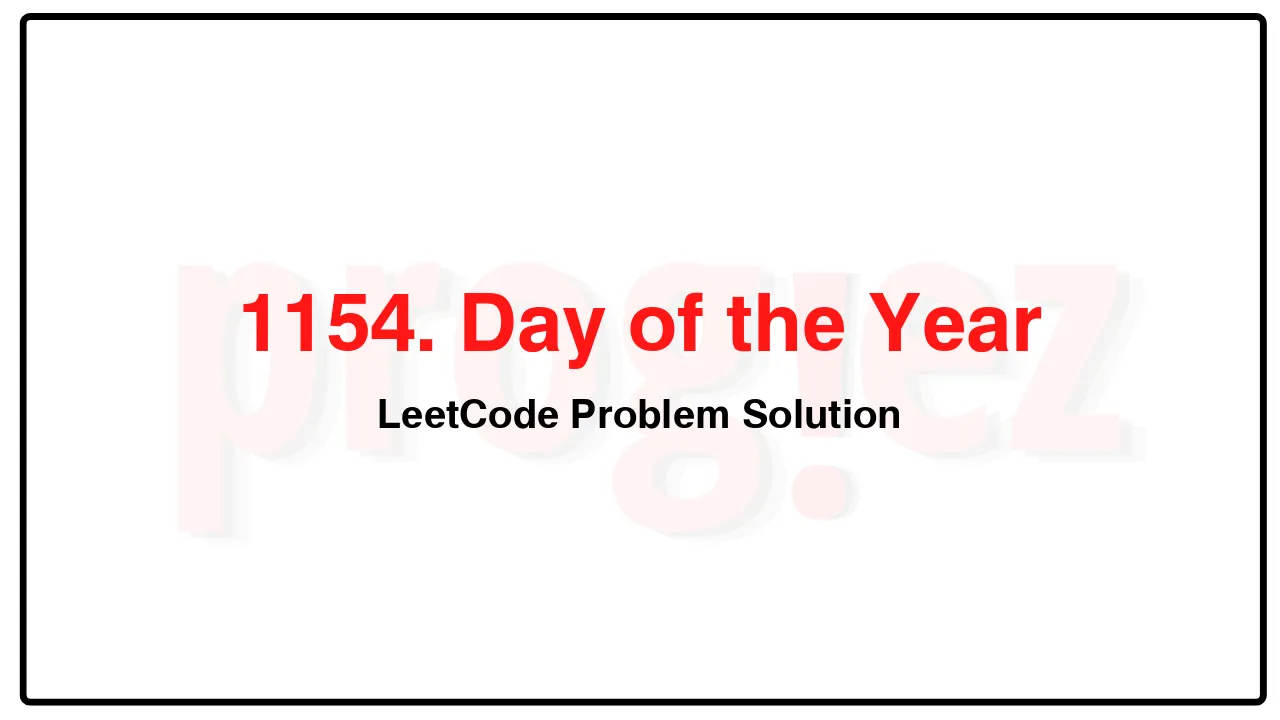
Problem Statement of Day of the Year
Given a string date representing a Gregorian calendar date formatted as YYYY-MM-DD, return the day number of the year.
Example 1:
Input: date = “2019-01-09”
Output: 9
Explanation: Given date is the 9th day of the year in 2019.
Example 2:
Input: date = “2019-02-10”
Output: 41
Constraints:
date.length == 10
date[4] == date[7] == ‘-‘, and all other date[i]’s are digits
date represents a calendar date between Jan 1st, 1900 and Dec 31st, 2019.
Complexity Analysis
- Time Complexity:
- Space Complexity:
1154. Day of the Year LeetCode Solution in C++
class Solution {
public:
int dayOfYear(string date) {
int year = stoi(date.substr(0, 4));
int month = stoi(date.substr(5, 2));
int day = stoi(date.substr(8));
vector<int> days = {
, isLeapYear(year) ? 29 : 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
return accumulate(days.begin(), days.begin() + month - 1, 0) + day;
}
private:
bool isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
};
/* code provided by PROGIEZ */
1154. Day of the Year LeetCode Solution in Java
class Solution {
public int dayOfYear(String date) {
int ans = 0;
int year = Integer.valueOf(date.substring(0, 4));
int month = Integer.valueOf(date.substring(5, 7));
int day = Integer.valueOf(date.substring(8));
int[] days = new int[] {31, isLeapYear(year) ? 29 : 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
for (int i = 0; i < month - 1; ++i)
ans += days[i];
return ans + day;
}
private boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
}
// code provided by PROGIEZ
1154. Day of the Year LeetCode Solution in Python
class Solution:
def dayOfYear(self, date: str) -> int:
def isLeapYear(year: int) -> bool:
return (year % 4 == 0 and year % 100 != 0) or year % 400 == 0
year = int(date[:4])
month = int(date[5:7])
day = int(date[8:])
days = [31, 29 if isLeapYear(
year) else 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
return sum(days[:month - 1]) + day
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.