1195. Fizz Buzz Multithreaded LeetCode Solution
In this guide, you will get 1195. Fizz Buzz Multithreaded LeetCode Solution with the best time and space complexity. The solution to Fizz Buzz Multithreaded problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Fizz Buzz Multithreaded solution in C++
- Fizz Buzz Multithreaded solution in Java
- Fizz Buzz Multithreaded solution in Python
- Additional Resources
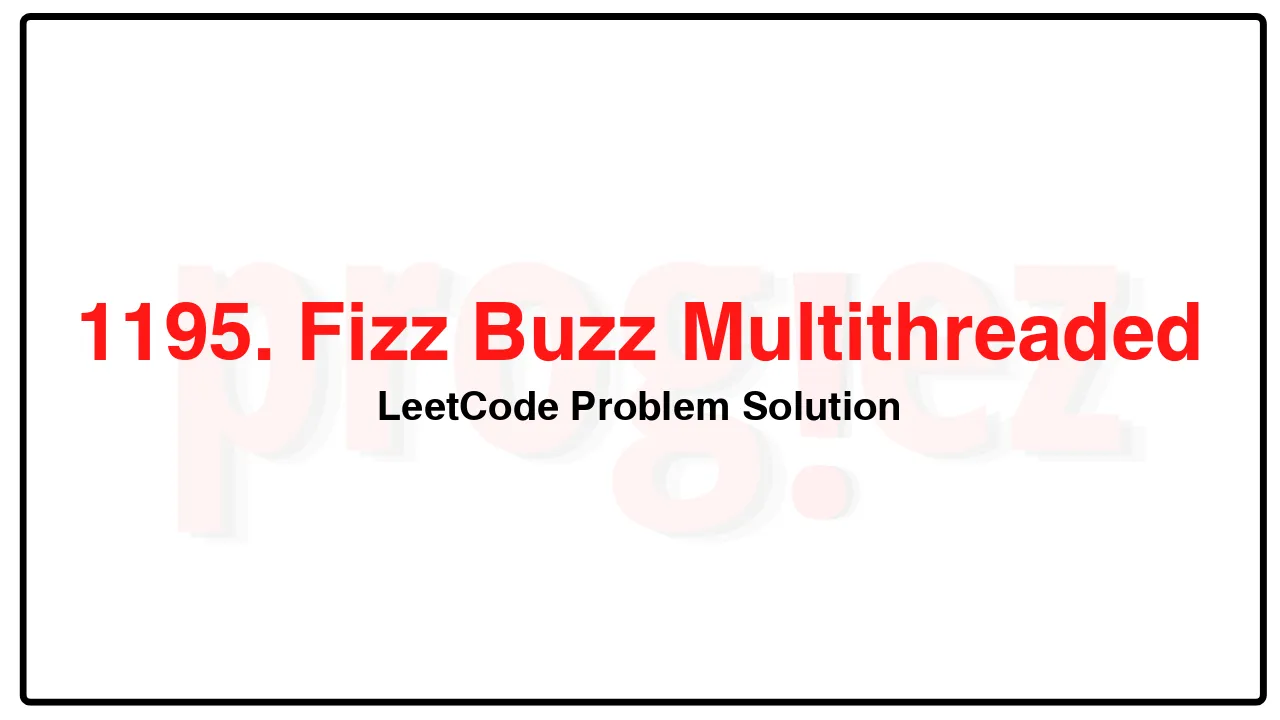
Problem Statement of Fizz Buzz Multithreaded
You have the four functions:
printFizz that prints the word “fizz” to the console,
printBuzz that prints the word “buzz” to the console,
printFizzBuzz that prints the word “fizzbuzz” to the console, and
printNumber that prints a given integer to the console.
You are given an instance of the class FizzBuzz that has four functions: fizz, buzz, fizzbuzz and number. The same instance of FizzBuzz will be passed to four different threads:
Thread A: calls fizz() that should output the word “fizz”.
Thread B: calls buzz() that should output the word “buzz”.
Thread C: calls fizzbuzz() that should output the word “fizzbuzz”.
Thread D: calls number() that should only output the integers.
Modify the given class to output the series [1, 2, “fizz”, 4, “buzz”, …] where the ith token (1-indexed) of the series is:
“fizzbuzz” if i is divisible by 3 and 5,
“fizz” if i is divisible by 3 and not 5,
“buzz” if i is divisible by 5 and not 3, or
i if i is not divisible by 3 or 5.
Implement the FizzBuzz class:
FizzBuzz(int n) Initializes the object with the number n that represents the length of the sequence that should be printed.
void fizz(printFizz) Calls printFizz to output “fizz”.
void buzz(printBuzz) Calls printBuzz to output “buzz”.
void fizzbuzz(printFizzBuzz) Calls printFizzBuzz to output “fizzbuzz”.
void number(printNumber) Calls printnumber to output the numbers.
Example 1:
Input: n = 15
Output: [1,2,”fizz”,4,”buzz”,”fizz”,7,8,”fizz”,”buzz”,11,”fizz”,13,14,”fizzbuzz”]
Example 2:
Input: n = 5
Output: [1,2,”fizz”,4,”buzz”]
Constraints:
1 <= n <= 50
Complexity Analysis
- Time Complexity: Google AdSense
- Space Complexity: Google Analytics
1195. Fizz Buzz Multithreaded LeetCode Solution in C++
// LeetCode doesn't support C++20 yet, so we don't have std::counting_semaphore
// or binary_semaphore.
#include <semaphore.h>
class FizzBuzz {
public:
FizzBuzz(int n) : n(n) {
sem_init(&fizzSemaphore, /*pshared=*/0, /*value=*/0);
sem_init(&buzzSemaphore, /*pshared=*/0, /*value=*/0);
sem_init(&fizzbuzzSemaphore, /*pshared=*/0, /*value=*/0);
sem_init(&numberSemaphore, /*pshared=*/0, /*value=*/1);
}
~FizzBuzz() {
sem_destroy(&fizzSemaphore);
sem_destroy(&buzzSemaphore);
sem_destroy(&fizzbuzzSemaphore);
sem_destroy(&numberSemaphore);
}
// printFizz() outputs "fizz".
void fizz(function<void()> printFizz) {
for (int i = 1; i <= n; ++i)
if (i % 3 == 0 && i % 15 != 0) {
sem_wait(&fizzSemaphore);
printFizz();
sem_post(&numberSemaphore);
}
}
// printBuzz() outputs "buzz".
void buzz(function<void()> printBuzz) {
for (int i = 1; i <= n; ++i)
if (i % 5 == 0 && i % 15 != 0) {
sem_wait(&buzzSemaphore);
printBuzz();
sem_post(&numberSemaphore);
}
}
// printFizzBuzz() outputs "fizzbuzz".
void fizzbuzz(function<void()> printFizzBuzz) {
for (int i = 1; i <= n; ++i)
if (i % 15 == 0) {
sem_wait(&fizzbuzzSemaphore);
printFizzBuzz();
sem_post(&numberSemaphore);
}
}
// printNumber(x) outputs "x", where x is an integer.
void number(function<void(int)> printNumber) {
for (int i = 1; i <= n; ++i) {
sem_wait(&numberSemaphore);
if (i % 15 == 0)
sem_post(&fizzbuzzSemaphore);
else if (i % 3 == 0)
sem_post(&fizzSemaphore);
else if (i % 5 == 0)
sem_post(&buzzSemaphore);
else {
printNumber(i);
sem_post(&numberSemaphore);
}
}
}
private:
const int n;
sem_t fizzSemaphore;
sem_t buzzSemaphore;
sem_t fizzbuzzSemaphore;
sem_t numberSemaphore;
};
/* code provided by PROGIEZ */
1195. Fizz Buzz Multithreaded LeetCode Solution in Java
class FizzBuzz {
public FizzBuzz(int n) {
this.n = n;
}
// printFizz.run() outputs "fizz".
public void fizz(Runnable printFizz) throws InterruptedException {
for (int i = 1; i <= n; ++i)
if (i % 3 == 0 && i % 15 != 0) {
fizzSemaphore.acquire();
printFizz.run();
numberSemaphore.release();
}
}
// printBuzz.run() outputs "buzz".
public void buzz(Runnable printBuzz) throws InterruptedException {
for (int i = 1; i <= n; ++i)
if (i % 5 == 0 && i % 15 != 0) {
buzzSemaphore.acquire();
printBuzz.run();
numberSemaphore.release();
}
}
// printFizzBuzz.run() outputs "fizzbuzz".
public void fizzbuzz(Runnable printFizzBuzz) throws InterruptedException {
for (int i = 1; i <= n; ++i)
if (i % 15 == 0) {
fizzbuzzSemaphore.acquire();
printFizzBuzz.run();
numberSemaphore.release();
}
}
// printNumber.accept(x) outputs "x", where x is an integer.
public void number(IntConsumer printNumber) throws InterruptedException {
for (int i = 1; i <= n; ++i) {
numberSemaphore.acquire();
if (i % 15 == 0)
fizzbuzzSemaphore.release();
else if (i % 3 == 0)
fizzSemaphore.release();
else if (i % 5 == 0)
buzzSemaphore.release();
else {
printNumber.accept(i);
numberSemaphore.release();
}
}
}
private int n;
private Semaphore fizzSemaphore = new Semaphore(0);
private Semaphore buzzSemaphore = new Semaphore(0);
private Semaphore fizzbuzzSemaphore = new Semaphore(0);
private Semaphore numberSemaphore = new Semaphore(1);
}
// code provided by PROGIEZ
1195. Fizz Buzz Multithreaded LeetCode Solution in Python
from threading import Semaphore
class FizzBuzz:
def __init__(self, n: int):
self.n = n
self.fizzSemaphore = Semaphore(0)
self.buzzSemaphore = Semaphore(0)
self.fizzbuzzSemaphore = Semaphore(0)
self.numberSemaphore = Semaphore(1)
# printFizz() outputs "fizz"
def fizz(self, printFizz: 'Callable[[], None]') -> None:
for i in range(1, self.n + 1):
if i % 3 == 0 and i % 15 != 0:
self.fizzSemaphore.acquire()
printFizz()
self.numberSemaphore.release()
# printBuzz() outputs "buzz"
def buzz(self, printBuzz: 'Callable[[], None]') -> None:
for i in range(1, self.n + 1):
if i % 5 == 0 and i % 15 != 0:
self.buzzSemaphore.acquire()
printBuzz()
self.numberSemaphore.release()
# printFizzBuzz() outputs "fizzbuzz"
def fizzbuzz(self, printFizzBuzz: 'Callable[[], None]') -> None:
for i in range(1, self.n + 1):
if i % 15 == 0:
self.fizzbuzzSemaphore.acquire()
printFizzBuzz()
self.numberSemaphore.release()
# printNumber(x) outputs "x", where x is an integer.
def number(self, printNumber: 'Callable[[int], None]') -> None:
for i in range(1, self.n + 1):
self.numberSemaphore.acquire()
if i % 15 == 0:
self.fizzbuzzSemaphore.release()
elif i % 3 == 0:
self.fizzSemaphore.release()
elif i % 5 == 0:
self.buzzSemaphore.release()
else:
printNumber(i)
self.numberSemaphore.release()
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.