Problem Solving Through Programming In C Nptel Week 4 Assignment Answers
Are you looking for the Problem Solving Through Programming In C Week 4 Answers? You’ve come to the right place! This resource offers comprehensive solutions to the Week 4 assignment questions, focusing on fundamental concepts in C programming.
Table of Contents
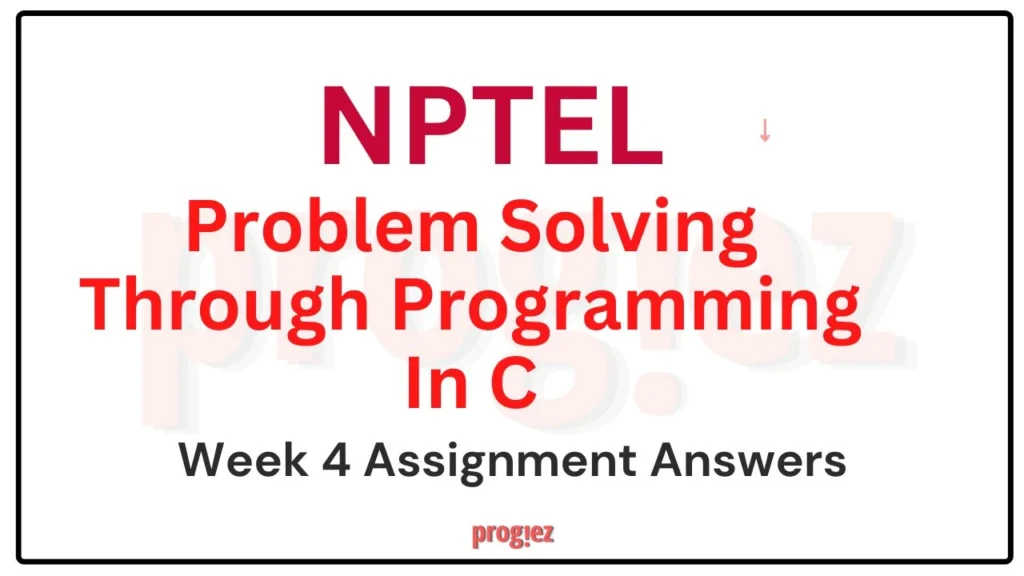
Problem Solving Through Programming In C Week 4 Answers (Jan-Apr 2025)
Course Link: Click Here
Q1. The control/conditional statements used in C is/are
a) if-else statements
b) switch statements
c) Both (a) and (b)
d) None of these
Q2. What is the output of the following C code?
#include <stdio.h>
int main() {
int x = 1;
if (x == 0)
if (x >= 0)
printf("x=0\n");
else
printf("x=1\n");
return 0;
}
a) x=0
b) x=1
c) Depends on compiler
d) No output
Q3. Compute the printed value of i
in the C program below:
#include <stdio.h>
int main() {
int i = 2;
i++;
printf("%d", i);
return 0;
}
a) 2
b) 3
c) 5
d) Compiler error
Q4. When multiple conditions are used in a single if
statement, the testing of those conditions is done:
a) From Right to Left
b) From Left to Right
c) Randomly
d) None
Q5. What is the purpose of the given program?
#include <stdio.h>
int main() {
int n, x = 0, y;
printf("Enter an integer: ");
scanf("%d", &n);
while (n != 0) {
y = n % 10;
x = x - y;
n = n / 10;
}
printf("Output is = %d", x);
return 0;
}
a) Sum of the digits of a number
b) Negative sum of the digits of a number
c) Reverse of a number
d) The same number is printed
Q6. What is the use of while
in a program?
a) False statement
b) Infinite loop
c) Terminating the loop
d) Never executed loop
Q7. What will be the values of a, b, c
after execution of the following?
int a = 5, b = 7, c = 1;
c /= ++a * b;
a) a = 5, b = 6, c = 2
b) a = 5, b = 7, c = 1
c) a = 6, b = 6, c = 2
d) a = 5, b = 7, c = ?
Q8. What will be printed when the following C code is executed?
#include <stdio.h>
int main() {
if ('A' < 'a')
printf("NPTEL");
else
printf("PROGRAMMING");
return 0;
}
a) NPTEL
b) PROGRAMMING
c) No output
d) Compiler error as A
and a
are not declared as character variables
Q9. In a switch
statement in C, which of the following data types is not accepted?
a) int
b) char
c) long
d) enum
Q10. What will be the output of the following program?
#include <stdio.h>
int main() {
int x = 1;
switch (x) {
case 1: printf("Choice is 1 \n");
default: printf("Choice other than 1");
}
return 0;
}
a) Choice is 1
b) Choice other than 1
c) Both (a) and (b)
d) Syntax error
Problem Solving Through Programming In C Week 4 Answers (July-Dec 2024)
Q1.The control/conditional statements used in C is/are
a) if-else statements
b) switch statements
c) Both (a) and (b)
d) None of the above
Answer:c) Both (a) and (b)
Q2.What is the output of the following C code?
a) x=1
b) x=0
c) Depends on compiler
d) No print statements
Answer:d) No print statements
For answers or latest updates join our telegram channel: Click here to join
These are Problem Solving Through Programming In C Week 4 Answers
Q3.Compute the printed value of i of the C program given below
a) 2
b) 3
c) 4
d) Compiler error
Answer: a) 2
Q4.f multiple conditions are used in a single if statement then the testing of those conditions are done
a) From Right to Left
b) From Left to Right
c) Randomly
d) None
Answer:b) From Left to Right
For answers or latest updates join our telegram channel: Click here to join
These are Problem Solving Through Programming In C Week 4 Answers
Q5.What is the purpose of the given program? n is the input number given by the user. #include
int main()
{
int n, x = 0, y;
printf(“Enter an integer: “);
scanf(“%d”, &n);
while (n != 0)
{
y = n % 10;
x = x – y;
n = n/10;
}
printf(“Output is = %d”, x);
return 0;
}
a) Sum of the digits of a number
b) The negative sum of the digits of a number
c) The reverse of a number
d) The same number is printed
Answer:b) The negative sum of the digits of a number
Q6.while(1) is used in a program to create
a) False statement
b) Infinite loop
c) Terminating the loop
d) Never executed loop
Answer: b) Infinite loop
For answers or latest updates join our telegram channel: Click here to join
These are Problem Solving Through Programming In C Week 4 Answers
Q7.Given the code snippet, what will be the value of ‘a’ after execution?
int a = 5, b = 10;
a += a <= b;
a) 5
b) 6
c) 10
d) 11
Answer:b) 6
Q8.What will be printed when the following C code is executed?
include
int main()
{
if(‘A'<‘a’)
printf(“NPTEL”);
else
printf(“PROGRAMMING”);
return 0;
}
a) NPTEL
b) PROGRAMMING
c) No output
d) Compilation error as A and a are not declared as character variable
Answer: a) NPTEL
For answers or latest updates join our telegram channel: Click here to join
These are Problem Solving Through Programming In C Week 4 Answers
Q9. Switch statement accepts
a) int
b) char
c) long
d) All of the above
Answer:d) All of the above
Q10.What will be the output of the following program?
include
int main()
{
int x = 1;
switch (x)
{
case 1: printf(“Choice is 1 \n”);
default: printf(“Choice other than 1 \n”);
}
return 0;
}
a) Choice is 1
b) Choice other than 1
c) Both (a) and (b)
d) Syntax error
Answer: c) Both (a) and (b)
For answers or latest updates join our telegram channel: Click here to join
These are Problem Solving Through Programming In C Week 4 Answers
All weeks of Problem Solving Through Programming in C : Click Here
More Nptel Courses: https://progiez.com/nptel-assignment-answers
Problem Solving Through Programming In C Week 4 Answers (JULY-DEC 2023)
Course Name: Problem Solving Through Programming In C
Course Link: Click Here
These are Problem Solving Through Programming In C Week 4 Answers
Q1. What is the purpose of the “if-else” statement in C?
a) To execute a block of code repeatedly.
b) To declare variables and constants.
c) To test a condition and execute different code based on the result.
d) To perform mathematical calculations.
Answer: c) To test a condition and execute different code based on the result.
Q2. What is the correct syntax for an “if-else” statement in C?
a) if condition { statement1; statement2; } else { statement3; }
b) if condition then { statement1; } else { statement2; }
c) if (condition) { statement1; } else { statement2; }
d) if condition then statement1; else statement2;
Answer: c) if (condition) { statement1; } else { statement2; }
Q3. Which of the following is true about nested “if-else” statements?
a) They are not allowed in C.
b) The “else” part is mandatory for every “if” statement.
c) They allow you to test multiple conditions and execute different blocks of code based on the results.
d) Nested “if-else” statements are only allowed up to two levels deep
Answer: c) They allow you to test multiple conditions and execute different blocks of code based on the results.
These are Problem Solving Through Programming In C Week 4 Answers
Q4. What happens if there is no “else” part in an “if-else” statement?
a) The program will not compile.
b) The program will crash at runtime.
c) If the condition is true, nothing happens; if the condition is false, the program crashes.
d) If the condition is true, the program executes the code inside the “if” block; if the condition is false, nothing happens.
Answer: d) If the condition is true, the program executes the code inside the “if” block; if the condition is false, nothing happens.
Q5. Which of the following operators can be used to combine multiple conditions in an “if” statement?
a) && (logical AND)
b) || (logical OR)
c) ! (logical NOT)
d) All of the above
Answer: d) All of the above
Q6. Compute the printed value of i of the C program given below
a) 2
b) 3
c) 4
d) Compiler error
Answer: a) 2
These are Problem Solving Through Programming In C Week 4 Answers
Q7. If multiple conditions are used in a single “if” statement then the testing of those conditions are done
a) From Right to Left
b) From Left to right
c) Randomly
d) None of the above
Answer: b) From Left to right
Q8. What is the purpose of the given program? n is the input number given by the user.
a) Sum of the digits of a number
b) The negative sum of the digits of a number
c) The reverse of a number
d) The same number is printed
Answer: b) The negative sum of the digits of a number
Q9. What will be the value of a, b, c after the execution of the followings
int a=5, b=7, c=111;
c/= ++a * b–;
a) a=5, b=6, c=2;
b) a=6, b=7, c=1;
c) a=6, b=6,c=2;
d) a=5, b=7, c=1;
Answer: c) a=6, b=6,c=2;
These are Problem Solving Through Programming In C Week 4 Answers
Q10. What will be the output of the following program?
a) Choice is 1
b) Choice other than 1
c) Both (a) and (b)
d) Syntax error
Answer: c) Both (a) and (b)
Assignment
Question 1
Write a C Program to Find the Smallest Number among Three Numbers (integer values) using Nested IF-Else statement.
Solution:
if(n1<n2)
{
if(n1<n3)
printf("%d is the smallest number.", n1);
else
printf("%d is the smallest number.", n3);
}
else
{
if(n2<n3)
printf("%d is the smallest number.", n2);
else
printf("%d is the smallest number.", n3);
}
}
Question 2
The length of three sides are taken as input. Write a C program to find whether a triangle can be formed or not. If not display “This Triangle is NOT possible.” If the triangle can be formed then check whether the triangle formed is equilateral, isosceles, scalene or a right-angled triangle. (If it is a right-angled triangle then only print Right-angle triangle do not print it as Scalene Triangle).
Solution:
if(a<(b+c)&&b<(a+c)&&c<(a+b))
{
if(a==b&&a==c&&b==c)
printf("Equilateral Triangle");
else if(a==b||a==c||b==c)
printf("Isosceles Triangle");
else if((a*a)==(b*b)+(c*c)||(b*b)==(a*a)+(c*c)||(c*c)==(a*a)+(b*b))
printf("Right-angle Triangle");
else if(a!=b&&a!=c&&b!=c)
printf("Scalene Triangle");
}
else
printf("Triangle is not possible");
}
Question 3
Write a program to find the factorial of a given number using while loop.
Solution:
int i=1;
fact = 1;
while(i<=n)
{
fact*=i;
i++;
}
printf("The Factorial of %d is : %ld",n,fact);
}
Question 4
Write a Program to find the sum of all even numbers from 1 to N where the value of N is taken as input. [For example when N is 10 then the sum is 2+4+6+8+10 = 30]
Solution:
for(int i = 2; i <= N; i += 2)
{
sum += i;
}
printf("Sum = %d",sum);
}
These are Problem Solving Through Programming In C Week 4 Answers
More Weeks of Problem Solving Through Programming In C: Click here
More Nptel Courses: Click here
Problem Solving Through Programming In C Week 4 Answers (JAN-APR 2023)
Course Name: Problem Solving Through Programming In C
Course Link: Click Here
These are Problem Solving Through Programming In C Week 4 Answers
Q1. The control/conditional statements used in C is/are
a) ‘if-else’ statements
b) ‘switch’ statements
c) Both (a) and (b)
d) None of these
Answer: c) Both (a) and (b)
Q2. What is the other statement that can avoid multiple nested if conditions?
a) Functions
b) ‘switch’ statements
c) ‘if-else’ statements with ‘break’
d) Loop statements
Answer: b) ‘switch’ statements
These are Problem Solving Through Programming In C Week 4 Answers
Q3. The loop which is executed at least one is
a) while
b) do-while
c) for
d) none of the above
Answer: b) do-while
Q4. ‘switch’ statement cannot use which of the following datatype:
a) int
b) char
c) short
d) float
Answer: d) float
These are Problem Solving Through Programming In C Week 4 Answers
Q5. Which of the following is a C Conditional Operator?
a) ?:
b) : ?
c) :<
d) <:
Answer: a) ?:
Q6.
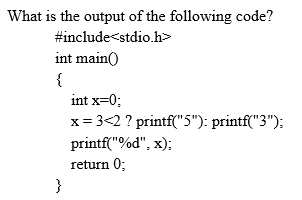
Answer: 31
These are Problem Solving Through Programming In C Week 4 Answers
Q7. Which of the following statement is correct regarding C ‘if-else’ statement?
a) ‘else if’ is compulsory to use with ‘if’ statement.
b) ‘else’ is compulsory to use with ‘if’ statement.
c) ‘else’ or ‘else if’ is optional with ‘if’ statement.
d) None of the above
Answer: c) ‘else’ or ‘else if’ is optional with ‘if’ statement.
Q8.
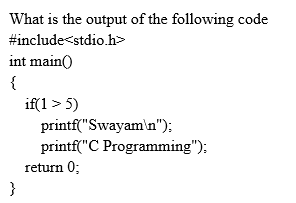
a) Swayam
b) C Programming
c) Swayam C Programming
d) It won’t print anything
Answer: b) C Programming
These are Problem Solving Through Programming In C Week 4 Answers
Q9. What will be the value of ‘i’ after the execution of the following statements?
Assume the initial values as i = 8; j = 5; k = 0.
k=(j>5)?(i<5)?i-jj-ik-j;
i-(k)?(1)?(j):(1):(k);
Answer: 3
Q10. What will be the value of a, b, c after the execution of the followings?
int a = 5, b = 7, c = 111;
c/= ++a* b–;
a) a=5, b=6, c=2;
b) a=6, b=7, c=1;
c) a=6, b=6, c=2;
d) a=5, b=7, c=1;
Answer: c) a=6, b=6, c=2;
These are Problem Solving Through Programming In C Week 4 Answers
Programming Assignment
Question 1
Write a C Program to Find the Smallest Number among Three Numbers (integer values) using Nested IF-Else statement.
Solution:
if((n1 < n2) && (n1 < n3))
printf("%d is the smallest number.", n1);
else if(n2 < n3)
printf("%d is the smallest number.", n2);
else
printf("%d is the smallest number.", n3);
}
Question 2
Write a program to find the factorial of a given number using while loop.
Solution:
fact = 1;
int num = n;
while(num>0) {
fact = fact * num;
num--;
}
printf("The Factorial of %d is : %ld",n,fact);
}
These are Problem Solving Through Programming In C Week 4 Answers
Question 3
Write a Program to find the sum of all even numbers from 1 to N where the value of N is taken as input. [For example when N is 10 then the sum is 2+4+6+8+10 = 30]
Solution:
for(int i=2;i<=N;i=i+2)
sum=sum+i;
printf("Sum = %d", sum);
}
Question 4
Write a C program to calculate the Sum of First and the Last Digit of a given Number. For example if the number is 1234 the result is 1+4 = 5.
Solution:
Last_digit = N % 10;
while(N > 0) {
First_digit = N;
N = N / 10;
}
These are Problem Solving Through Programming In C Week 4 Answers
More Weeks of Problem Solving Through Programming In C: Click Here
More Nptel courses: https://progiez.com/nptel
Problem Solving Through Programming In C Week 4 Answers (JULY-DEC 2022)
Course Name: Problem Solving Through Programming In C NPTEL
Link of course: Click Here
These are Problem Solving Through Programming In C Week 4 Answers
Q1) The loop which is executed at least once is
a) “while” loop
b) “do-while” loop
c) “for” loop
d) None of the above
Answer: b) “do-while” loop
Q2) In the C programming language negative numbers when used in if-else conditional checking, are treated as
a) TRUE
b) FALSE
c) Depends on the implementation
d) None of these
Answer: a) TRUE
Q3) Choose the correct statement to use “if-else” statement in C Language
a) “else if” is compulsory to use with “if” statement.
b) “else” is compulsory to use with “if” statement.
c) “else” or “else if” is optional with the “if” statement.
d) None of the above are correct
Answer: c) “else” or “else if” is optional with the “if” statement.
These are Problem Solving Through Programming In C Week 4 Answers
Q4) What is the output of the following C code?
#include
int main()
{
int a = 1;
if (a–)
printf(“True\n”);
if (++a)
printf(“False\n”);
return 0;
}
a) True
b) False
c) Both ‘True’ and ‘False’ are printed
d) Compilation error
Answer: c) Both ‘True’ and ‘False’ are printed
These are Problem Solving Through Programming In C Week 4 Answers
Q5) In the following example, tell which statement is correct
if(condition1== 1)) && if( (condition2==1)
printf(“Swayam”);
a) Condition 1 will be evaluated first, and condition2 will be evaluated second
b) Condition2 will be evaluated first, and condition1 will be evaluated second
c) Condition 1 will be evaluated first, condition2 will be evaluated only if the condition1 is TRUE
d) Condition2 will be evaluated first, and condition1 will be evaluated only if condition2 is TRUE
Answer: c) Condition 1 will be evaluated first, condition2 will be evaluated only if the condition1 is TRUE
Q6) Which one of the following is the correct syntax for Ternary Operator in C language?
a) condition? expression1 : expression2
b) condition : expression1 ? expression2
c) condition? expression 1 < expression2
d) condition expression1 ? expression2
Answer: a) condition? expression1 : expression2
These are Problem Solving Through Programming In C Week 4 Answers
Q7) The purpose of the following program fragment is to
b=s+b;
s=b-s;
b=b-s;(where s and b are two integers)
a) Transfer the content of s to b
b) Transfer the content of b to s
c) Exchange (swap) the content of s and b
d) Negate the contents of s and b
Answer: c) Exchange (swap) the content of s and b
Q8) What will be the output?
#include
int main()
{
int x=0;
x = printf(“3”);
printf(“%d”,x);
return 0;
}
a) 11
b) 33
c) 31
d) 13
Answer: c) 31
These are Problem Solving Through Programming In C Week 4 Answers
Q9) What will be the output?
#include <stdio.h>
int main()
{
int i=0, j=1;
printf(“\n%d i++ && ++j);
printf(“\n%d”, %d”, ij);
return 0;
}
a) 0 12
b) 1 11
c) 0 00
d) 0 11
Answer: d) 0 11
Q10) What will be the value of a, b, and c after the execution of the following
int a = 5, b = 7, c = 111;
c/= ++a* b–;
a) a=5, b=6, c=2;
b) a=6, b=7, c=1;
c) a=6, b=6, c=2;
d) a=5, b=7, c=1;
Answer: c) a=6, b=6, c=2;
These are Problem Solving Through Programming In C Week 4 Answers