902. Numbers At Most N Given Digit Set LeetCode Solution
In this guide, you will get 902. Numbers At Most N Given Digit Set LeetCode Solution with the best time and space complexity. The solution to Numbers At Most N Given Digit Set problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Numbers At Most N Given Digit Set solution in C++
- Numbers At Most N Given Digit Set solution in Java
- Numbers At Most N Given Digit Set solution in Python
- Additional Resources
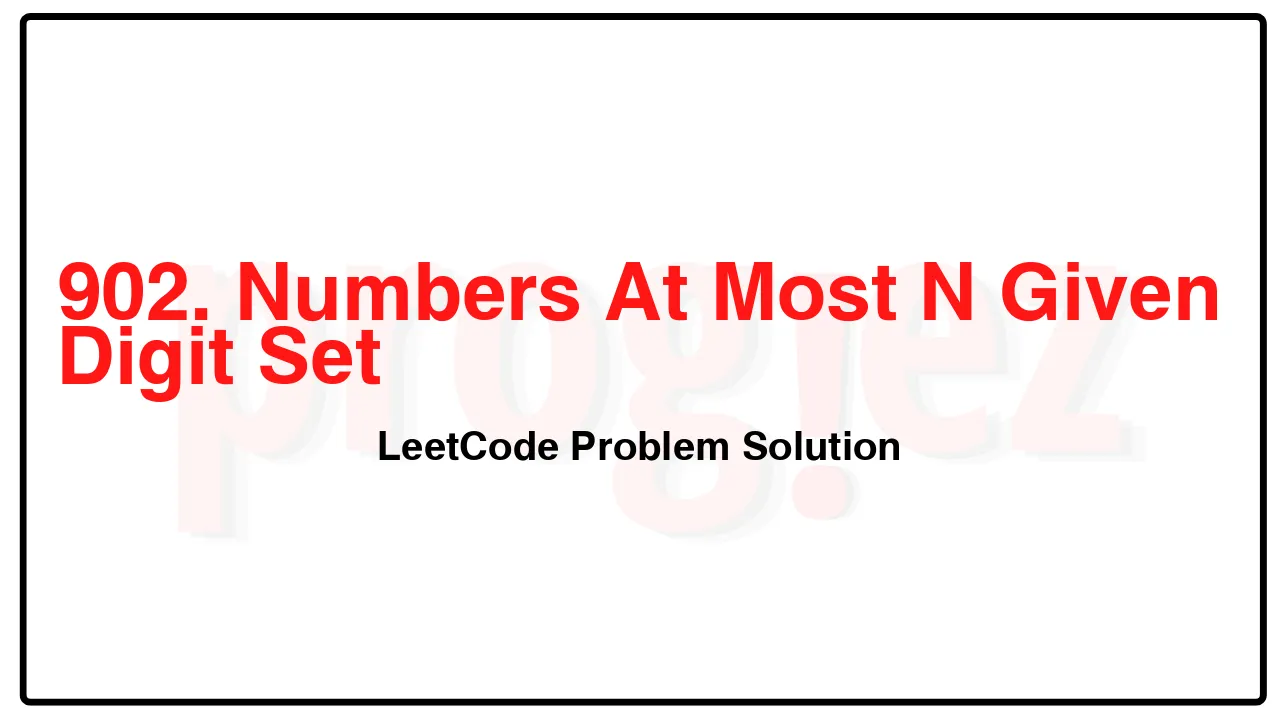
Problem Statement of Numbers At Most N Given Digit Set
Given an array of digits which is sorted in non-decreasing order. You can write numbers using each digits[i] as many times as we want. For example, if digits = [‘1′,’3′,’5′], we may write numbers such as ’13’, ‘551’, and ‘1351315’.
Return the number of positive integers that can be generated that are less than or equal to a given integer n.
Example 1:
Input: digits = [“1″,”3″,”5″,”7”], n = 100
Output: 20
Explanation:
The 20 numbers that can be written are:
1, 3, 5, 7, 11, 13, 15, 17, 31, 33, 35, 37, 51, 53, 55, 57, 71, 73, 75, 77.
Example 2:
Input: digits = [“1″,”4″,”9”], n = 1000000000
Output: 29523
Explanation:
We can write 3 one digit numbers, 9 two digit numbers, 27 three digit numbers,
81 four digit numbers, 243 five digit numbers, 729 six digit numbers,
2187 seven digit numbers, 6561 eight digit numbers, and 19683 nine digit numbers.
In total, this is 29523 integers that can be written using the digits array.
Example 3:
Input: digits = [“7”], n = 8
Output: 1
Constraints:
1 <= digits.length <= 9
digits[i].length == 1
digits[i] is a digit from '1' to '9'.
All the values in digits are unique.
digits is sorted in non-decreasing order.
1 <= n <= 109
Complexity Analysis
- Time Complexity: O(\log n)
- Space Complexity: O(\log n)
902. Numbers At Most N Given Digit Set LeetCode Solution in C++
class Solution {
public:
int atMostNGivenDigitSet(vector<string>& digits, int n) {
int ans = 0;
const string num = to_string(n);
for (int i = 1; i < num.length(); ++i)
ans += pow(digits.size(), i);
for (int i = 0; i < num.length(); ++i) {
bool dHasSameNum = false;
for (const string& digit : digits) {
if (digit[0] < num[i])
ans += pow(digits.size(), num.length() - i - 1);
else if (digit[0] == num[i])
dHasSameNum = true;
}
if (!dHasSameNum)
return ans;
}
return ans + 1;
}
};
/* code provided by PROGIEZ */
902. Numbers At Most N Given Digit Set LeetCode Solution in Java
class Solution {
public int atMostNGivenDigitSet(String[] digits, int n) {
int ans = 0;
final String num = String.valueOf(n);
for (int i = 1; i < num.length(); ++i)
ans += (int) Math.pow(digits.length, i);
for (int i = 0; i < num.length(); ++i) {
boolean dHasSameNum = false;
for (final String digit : digits) {
if (digit.charAt(0) < num.charAt(i))
ans += (int) Math.pow(digits.length, num.length() - i - 1);
else if (digit.charAt(0) == num.charAt(i))
dHasSameNum = true;
}
if (!dHasSameNum)
return ans;
}
return ans + 1;
}
}
// code provided by PROGIEZ
902. Numbers At Most N Given Digit Set LeetCode Solution in Python
class Solution:
def atMostNGivenDigitSet(self, digits: list[str], n: int) -> int:
ans = 0
num = str(n)
for i in range(1, len(num)):
ans += pow(len(digits), i)
for i, c in enumerate(num):
dHasSameNum = False
for digit in digits:
if digit[0] < c:
ans += pow(len(digits), len(num) - i - 1)
elif digit[0] == c:
dHasSameNum = True
if not dHasSameNum:
return ans
return ans + 1
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.