468. Validate IP Address LeetCode Solution
In this guide, you will get 468. Validate IP Address LeetCode Solution with the best time and space complexity. The solution to Validate IP Address problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Validate IP Address solution in C++
- Validate IP Address solution in Java
- Validate IP Address solution in Python
- Additional Resources
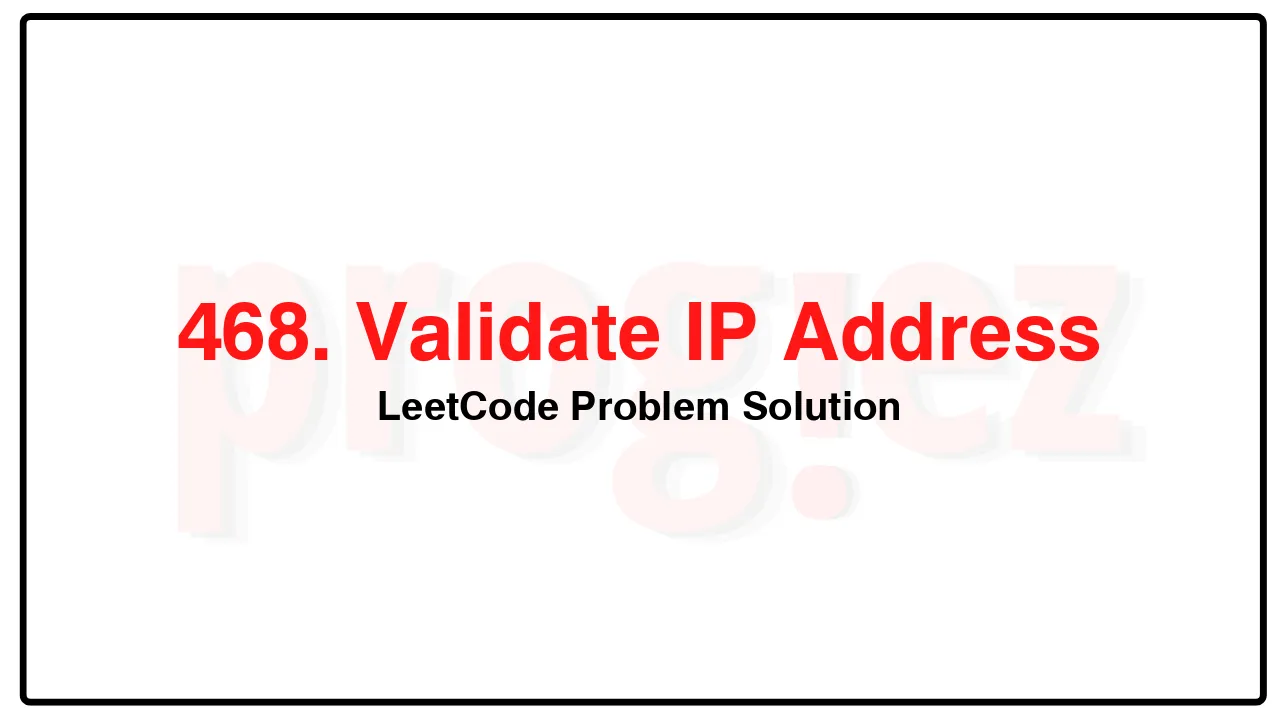
Problem Statement of Validate IP Address
Given a string queryIP, return “IPv4” if IP is a valid IPv4 address, “IPv6” if IP is a valid IPv6 address or “Neither” if IP is not a correct IP of any type.
A valid IPv4 address is an IP in the form “x1.x2.x3.x4” where 0 <= xi <= 255 and xi cannot contain leading zeros. For example, "192.168.1.1" and "192.168.1.0" are valid IPv4 addresses while "192.168.01.1", "192.168.1.00", and "192.168@1.1" are invalid IPv4 addresses.
A valid IPv6 address is an IP in the form "x1:x2:x3:x4:x5:x6:x7:x8" where:
1 <= xi.length <= 4
xi is a hexadecimal string which may contain digits, lowercase English letter ('a' to 'f') and upper-case English letters ('A' to 'F').
Leading zeros are allowed in xi.
For example, "2001:0db8:85a3:0000:0000:8a2e:0370:7334" and "2001:db8:85a3:0:0:8A2E:0370:7334" are valid IPv6 addresses, while "2001:0db8:85a3::8A2E:037j:7334" and "02001:0db8:85a3:0000:0000:8a2e:0370:7334" are invalid IPv6 addresses.
Example 1:
Input: queryIP = “172.16.254.1”
Output: “IPv4”
Explanation: This is a valid IPv4 address, return “IPv4”.
Example 2:
Input: queryIP = “2001:0db8:85a3:0:0:8A2E:0370:7334”
Output: “IPv6”
Explanation: This is a valid IPv6 address, return “IPv6”.
Example 3:
Input: queryIP = “256.256.256.256”
Output: “Neither”
Explanation: This is neither a IPv4 address nor a IPv6 address.
Constraints:
queryIP consists only of English letters, digits and the characters ‘.’ and ‘:’.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
468. Validate IP Address LeetCode Solution in C++
class Solution {
public:
string validIPAddress(string IP) {
string digit;
istringstream iss(IP);
if (ranges::count(IP, '.') == 3) {
for (int i = 0; i < 4; ++i) // Make sure that we have four parts.
if (!getline(iss, digit, '.') || !isIPv4(digit))
return "Neither";
return "IPv4";
}
if (ranges::count(IP, ':') == 7) {
for (int i = 0; i < 8; ++i) // Make sure that we have eight parts.
if (!getline(iss, digit, ':') || !isIPv6(digit))
return "Neither";
return "IPv6";
}
return "Neither";
}
private:
static inline string validIPv6Chars = "0123456789abcdefABCDEF";
bool isIPv4(const string& digit) {
if (digit.empty() || digit.length() > 3)
return false;
if (digit.length() > 1 && digit[0] == '0')
return false;
for (const char c : digit)
if (c < '0' || c > '9')
return false;
const int num = stoi(digit);
return 0 <= num && num <= 255;
}
bool isIPv6(const string& digit) {
if (digit.empty() || digit.length() > 4)
return false;
for (const char c : digit)
if (validIPv6Chars.find(c) == string::npos)
return false;
return true;
}
};
/* code provided by PROGIEZ */
468. Validate IP Address LeetCode Solution in Java
class Solution {
public String validIPAddress(String IP) {
if (IP.chars().filter(c -> c == '.').count() == 3) {
for (final String digit : IP.split("\\.", -1))
if (!isIPv4(digit))
return "Neither";
return "IPv4";
}
if (IP.chars().filter(c -> c == ':').count() == 7) {
for (final String digit : IP.split("\\:", -1))
if (!isIPv6(digit))
return "Neither";
return "IPv6";
}
return "Neither";
}
private static final String validIPv6Chars = "0123456789abcdefABCDEF";
private boolean isIPv4(final String digit) {
if (digit.isEmpty() || digit.length() > 3)
return false;
if (digit.length() > 1 && digit.charAt(0) == '0')
return false;
for (final char c : digit.toCharArray())
if (c < '0' || c > '9')
return false;
final int num = Integer.parseInt(digit);
return 0 <= num && num <= 255;
}
private boolean isIPv6(final String digit) {
if (digit.isEmpty() || digit.length() > 4)
return false;
for (final char c : digit.toCharArray())
if (!validIPv6Chars.contains("" + c))
return false;
return true;
}
}
// code provided by PROGIEZ
468. Validate IP Address LeetCode Solution in Python
N/A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.