885. Spiral Matrix III LeetCode Solution
In this guide, you will get 885. Spiral Matrix III LeetCode Solution with the best time and space complexity. The solution to Spiral Matrix III problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Spiral Matrix III solution in C++
- Spiral Matrix III solution in Java
- Spiral Matrix III solution in Python
- Additional Resources
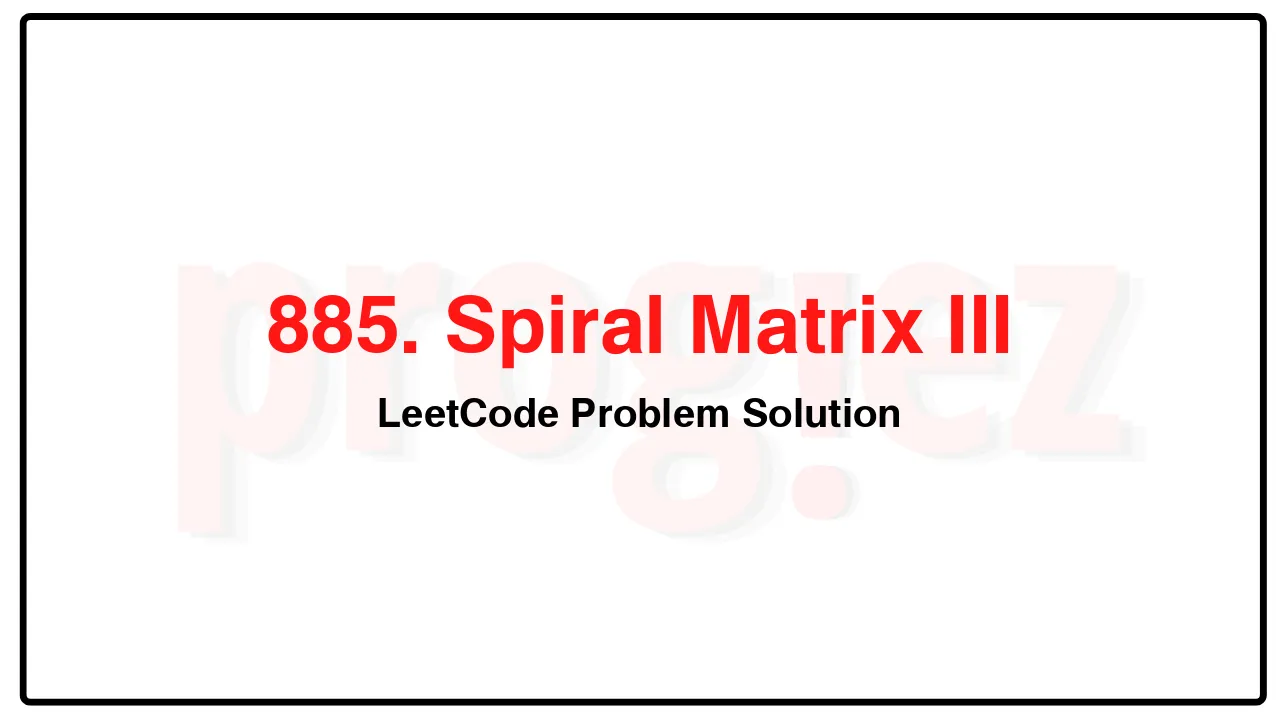
Problem Statement of Spiral Matrix III
You start at the cell (rStart, cStart) of an rows x cols grid facing east. The northwest corner is at the first row and column in the grid, and the southeast corner is at the last row and column.
You will walk in a clockwise spiral shape to visit every position in this grid. Whenever you move outside the grid’s boundary, we continue our walk outside the grid (but may return to the grid boundary later.). Eventually, we reach all rows * cols spaces of the grid.
Return an array of coordinates representing the positions of the grid in the order you visited them.
Example 1:
Input: rows = 1, cols = 4, rStart = 0, cStart = 0
Output: [[0,0],[0,1],[0,2],[0,3]]
Example 2:
Input: rows = 5, cols = 6, rStart = 1, cStart = 4
Output: [[1,4],[1,5],[2,5],[2,4],[2,3],[1,3],[0,3],[0,4],[0,5],[3,5],[3,4],[3,3],[3,2],[2,2],[1,2],[0,2],[4,5],[4,4],[4,3],[4,2],[4,1],[3,1],[2,1],[1,1],[0,1],[4,0],[3,0],[2,0],[1,0],[0,0]]
Constraints:
1 <= rows, cols <= 100
0 <= rStart < rows
0 <= cStart < cols
Complexity Analysis
- Time Complexity: O(\max^2(R, C))
- Space Complexity: O(R \cdot C)
885. Spiral Matrix III LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> spiralMatrixIII(int rows, int cols, int rStart,
int cStart) {
const vector<int> dx{1, 0, -1, 0};
const vector<int> dy{0, 1, 0, -1};
vector<vector<int>> ans{{rStart, cStart}};
for (int i = 0; ans.size() < rows * cols; ++i)
for (int step = 0; step < i / 2 + 1; ++step) {
rStart += dy[i % 4];
cStart += dx[i % 4];
if (0 <= rStart && rStart < rows && 0 <= cStart && cStart < cols)
ans.push_back({rStart, cStart});
}
return ans;
}
};
/* code provided by PROGIEZ */
885. Spiral Matrix III LeetCode Solution in Java
class Solution {
public int[][] spiralMatrixIII(int rows, int cols, int rStart, int cStart) {
final int[] dx = {1, 0, -1, 0};
final int[] dy = {0, 1, 0, -1};
List<int[]> ans = new ArrayList<>(List.of(new int[] {rStart, cStart}));
for (int i = 0; ans.size() < rows * cols; ++i)
for (int step = 0; step < i / 2 + 1; ++step) {
rStart += dy[i % 4];
cStart += dx[i % 4];
if (0 <= rStart && rStart < rows && 0 <= cStart && cStart < cols)
ans.add(new int[] {rStart, cStart});
}
return ans.stream().toArray(int[][] ::new);
}
}
// code provided by PROGIEZ
885. Spiral Matrix III LeetCode Solution in Python
class Solution:
def spiralMatrixIII(self, rows: int, cols: int, rStart: int, cStart: int) -> list[list[int]]:
dx = [1, 0, -1, 0]
dy = [0, 1, 0, -1]
ans = [[rStart, cStart]]
i = 0
while len(ans) < rows * cols:
for _ in range(i // 2 + 1):
rStart += dy[i % 4]
cStart += dx[i % 4]
if 0 <= rStart < rows and 0 <= cStart < cols:
ans.append([rStart, cStart])
i += 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.