1491. Average Salary Excluding the Minimum and Maximum Salary LeetCode Solution
In this guide, you will get 1491. Average Salary Excluding the Minimum and Maximum Salary LeetCode Solution with the best time and space complexity. The solution to Average Salary Excluding the Minimum and Maximum Salary problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Average Salary Excluding the Minimum and Maximum Salary solution in C++
- Average Salary Excluding the Minimum and Maximum Salary solution in Java
- Average Salary Excluding the Minimum and Maximum Salary solution in Python
- Additional Resources
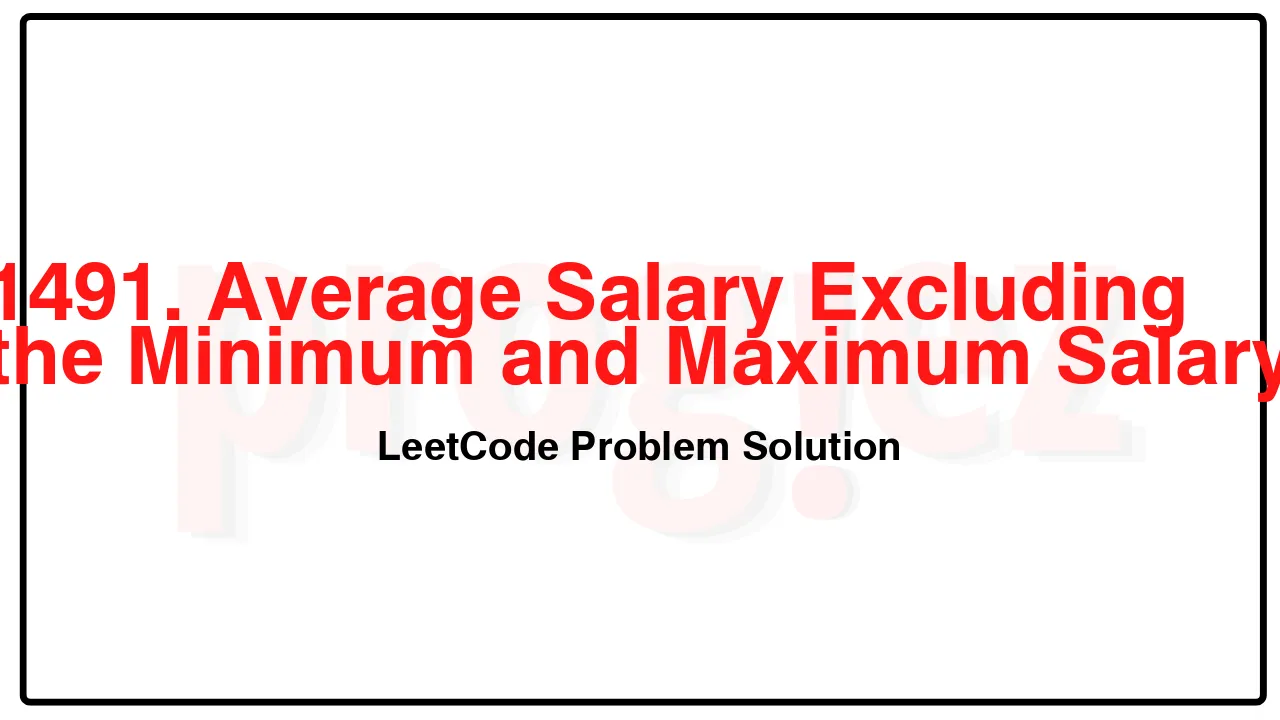
Problem Statement of Average Salary Excluding the Minimum and Maximum Salary
You are given an array of unique integers salary where salary[i] is the salary of the ith employee.
Return the average salary of employees excluding the minimum and maximum salary. Answers within 10-5 of the actual answer will be accepted.
Example 1:
Input: salary = [4000,3000,1000,2000]
Output: 2500.00000
Explanation: Minimum salary and maximum salary are 1000 and 4000 respectively.
Average salary excluding minimum and maximum salary is (2000+3000) / 2 = 2500
Example 2:
Input: salary = [1000,2000,3000]
Output: 2000.00000
Explanation: Minimum salary and maximum salary are 1000 and 3000 respectively.
Average salary excluding minimum and maximum salary is (2000) / 1 = 2000
Constraints:
3 <= salary.length <= 100
1000 <= salary[i] <= 106
All the integers of salary are unique.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
1491. Average Salary Excluding the Minimum and Maximum Salary LeetCode Solution in C++
class Solution {
public:
double average(vector<int>& salary) {
const double sum = accumulate(salary.begin(), salary.end(), 0.0);
const int mx = ranges::max(salary);
const int mn = ranges::min(salary);
return (sum - mx - mn) / (salary.size() - 2);
}
};
/* code provided by PROGIEZ */
1491. Average Salary Excluding the Minimum and Maximum Salary LeetCode Solution in Java
class Solution {
public double average(int[] salary) {
final double sum = Arrays.stream(salary).sum();
final int mx = Arrays.stream(salary).max().getAsInt();
final int mn = Arrays.stream(salary).min().getAsInt();
return (sum - mx - mn) / (salary.length - 2);
}
}
// code provided by PROGIEZ
1491. Average Salary Excluding the Minimum and Maximum Salary LeetCode Solution in Python
class Solution:
def average(self, salary: list[int]) -> float:
return (sum(salary) - max(salary) - min(salary)) / (len(salary) - 2)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.