59. Spiral Matrix II LeetCode Solution
In this guide, you will get 59. Spiral Matrix II LeetCode Solution with the best time and space complexity. The solution to Spiral Matrix II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Spiral Matrix II solution in C++
- Spiral Matrix II solution in Java
- Spiral Matrix II solution in Python
- Additional Resources
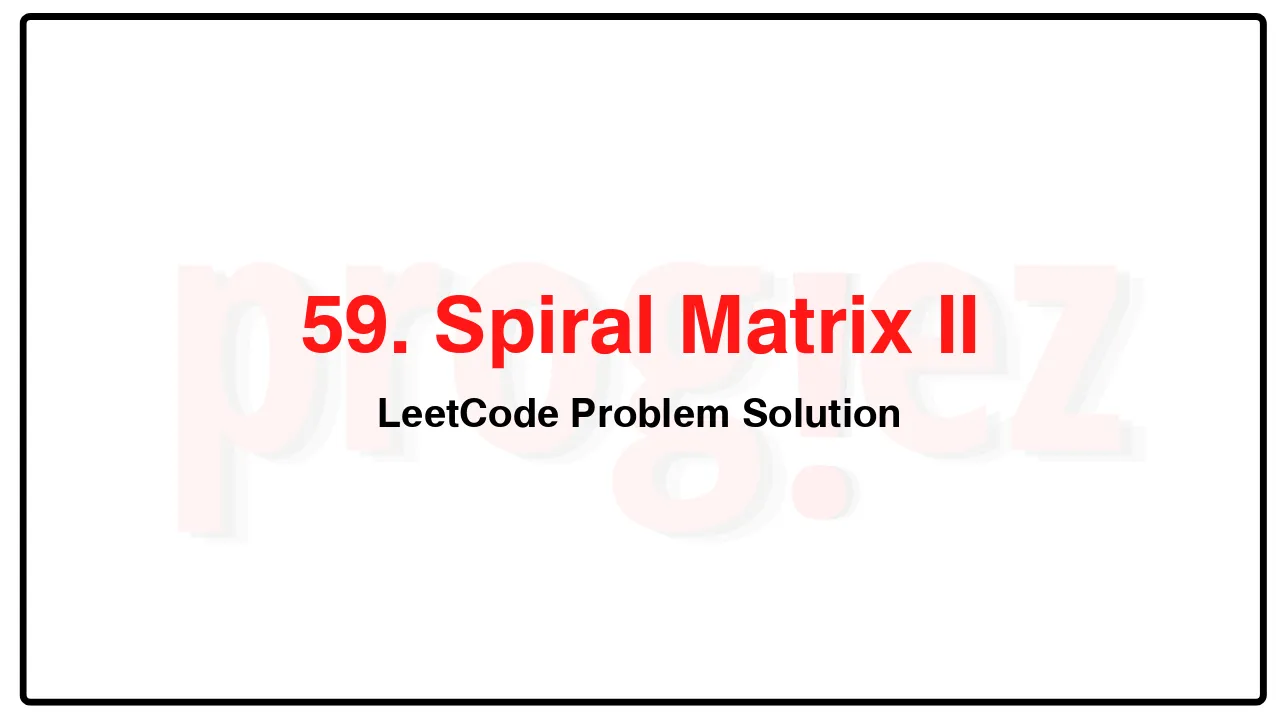
Problem Statement of Spiral Matrix II
Given a positive integer n, generate an n x n matrix filled with elements from 1 to n2 in spiral order.
Example 1:
Input: n = 3
Output: [[1,2,3],[8,9,4],[7,6,5]]
Example 2:
Input: n = 1
Output: [[1]]
Constraints:
1 <= n <= 20
Complexity Analysis
- Time Complexity: O(n^2)
- Space Complexity: O(n^2)
59. Spiral Matrix II LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> generateMatrix(int n) {
vector<vector<int>> ans(n, vector<int>(n));
int count = 1;
for (int mn = 0; mn < n / 2; ++mn) {
const int mx = n - mn - 1;
for (int i = mn; i < mx; ++i)
ans[mn][i] = count++;
for (int i = mn; i < mx; ++i)
ans[i][mx] = count++;
for (int i = mx; i > mn; --i)
ans[mx][i] = count++;
for (int i = mx; i > mn; --i)
ans[i][mn] = count++;
}
if (n % 2 == 1)
ans[n / 2][n / 2] = count;
return ans;
}
};
/* code provided by PROGIEZ */
59. Spiral Matrix II LeetCode Solution in Java
class Solution {
public int[][] generateMatrix(int n) {
int[][] ans = new int[n][n];
int count = 1;
for (int mn = 0; mn < n / 2; ++mn) {
final int mx = n - mn - 1;
for (int i = mn; i < mx; ++i)
ans[mn][i] = count++;
for (int i = mn; i < mx; ++i)
ans[i][mx] = count++;
for (int i = mx; i > mn; --i)
ans[mx][i] = count++;
for (int i = mx; i > mn; --i)
ans[i][mn] = count++;
}
if (n % 2 == 1)
ans[n / 2][n / 2] = count;
return ans;
}
}
// code provided by PROGIEZ
59. Spiral Matrix II LeetCode Solution in Python
class Solution:
def generateMatrix(self, n: int) -> list[list[int]]:
ans = [[0] * n for _ in range(n)]
count = 1
for mn in range(n // 2):
mx = n - mn - 1
for i in range(mn, mx):
ans[mn][i] = count
count += 1
for i in range(mn, mx):
ans[i][mx] = count
count += 1
for i in range(mx, mn, -1):
ans[mx][i] = count
count += 1
for i in range(mx, mn, -1):
ans[i][mn] = count
count += 1
if n % 2 == 1:
ans[n // 2][n // 2] = count
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.