768. Max Chunks To Make Sorted II LeetCode Solution
In this guide, you will get 768. Max Chunks To Make Sorted II LeetCode Solution with the best time and space complexity. The solution to Max Chunks To Make Sorted II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Max Chunks To Make Sorted II solution in C++
- Max Chunks To Make Sorted II solution in Java
- Max Chunks To Make Sorted II solution in Python
- Additional Resources
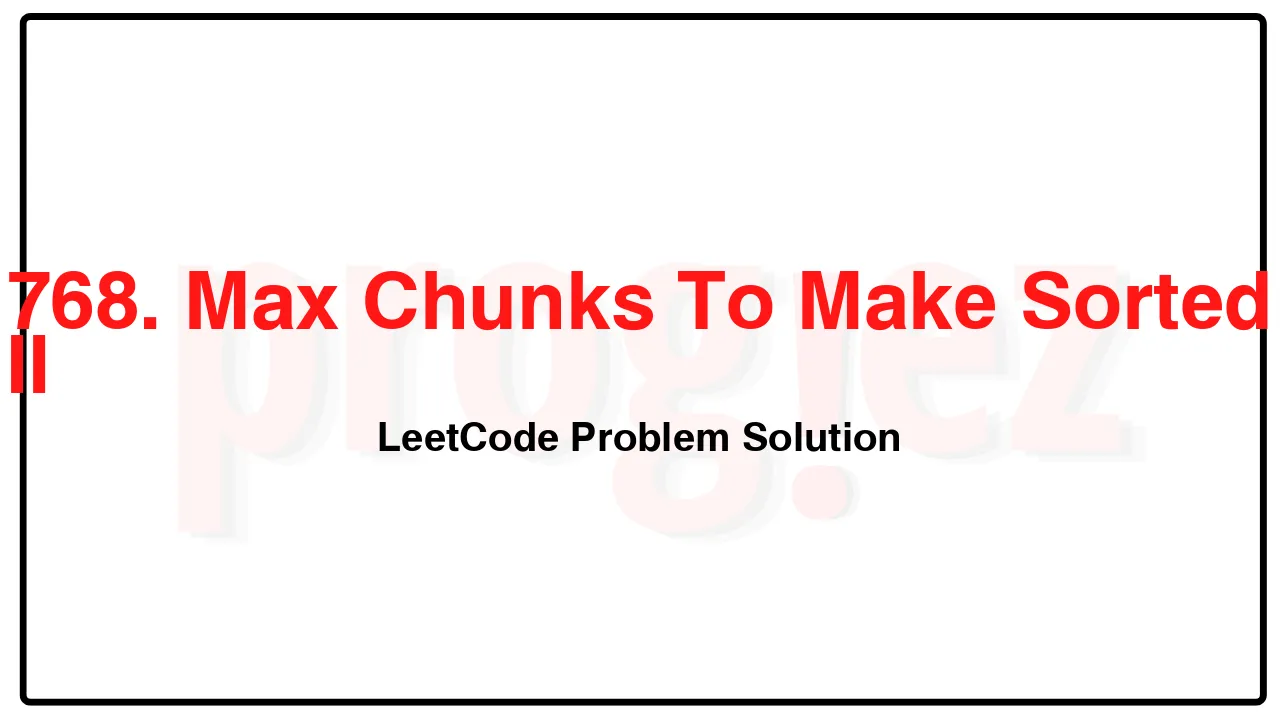
Problem Statement of Max Chunks To Make Sorted II
You are given an integer array arr.
We split arr into some number of chunks (i.e., partitions), and individually sort each chunk. After concatenating them, the result should equal the sorted array.
Return the largest number of chunks we can make to sort the array.
Example 1:
Input: arr = [5,4,3,2,1]
Output: 1
Explanation:
Splitting into two or more chunks will not return the required result.
For example, splitting into [5, 4], [3, 2, 1] will result in [4, 5, 1, 2, 3], which isn’t sorted.
Example 2:
Input: arr = [2,1,3,4,4]
Output: 4
Explanation:
We can split into two chunks, such as [2, 1], [3, 4, 4].
However, splitting into [2, 1], [3], [4], [4] is the highest number of chunks possible.
Constraints:
1 <= arr.length <= 2000
0 <= arr[i] <= 108
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
768. Max Chunks To Make Sorted II LeetCode Solution in C++
class Solution {
public:
int maxChunksToSorted(vector<int>& arr) {
const int n = arr.size();
int ans = 0;
vector<int> maxL(n); // l[i] := max(arr[0..i])
vector<int> minR(n); // r[i] := min(arr[i..n))
for (int i = 0; i < n; ++i)
maxL[i] = i == 0 ? arr[i] : max(arr[i], maxL[i - 1]);
for (int i = n - 1; i >= 0; --i)
minR[i] = i == n - 1 ? arr[i] : min(arr[i], minR[i + 1]);
for (int i = 0; i + 1 < n; ++i)
if (maxL[i] <= minR[i + 1])
++ans;
return ans + 1;
}
};
/* code provided by PROGIEZ */
768. Max Chunks To Make Sorted II LeetCode Solution in Java
class Solution {
public int maxChunksToSorted(int[] arr) {
final int n = arr.length;
int ans = 0;
int[] maxL = new int[n]; // l[i] := max(arr[0..i])
int[] minR = new int[n]; // r[i] := min(arr[i..n))
for (int i = 0; i < n; ++i)
maxL[i] = i == 0 ? arr[i] : Math.max(arr[i], maxL[i - 1]);
for (int i = n - 1; i >= 0; --i)
minR[i] = i == n - 1 ? arr[i] : Math.min(arr[i], minR[i + 1]);
for (int i = 0; i + 1 < n; ++i)
if (maxL[i] <= minR[i + 1])
++ans;
return ans + 1;
}
}
// code provided by PROGIEZ
768. Max Chunks To Make Sorted II LeetCode Solution in Python
class Solution:
def maxChunksToSorted(self, arr: list[int]) -> int:
n = len(arr)
ans = 0
mx = -math.inf
mn = [arr[-1]] * n
for i in reversed(range(n - 1)):
mn[i] = min(mn[i + 1], arr[i])
for i in range(n - 1):
mx = max(mx, arr[i])
if mx <= mn[i + 1]:
ans += 1
return ans + 1
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.