845. Longest Mountain in Array LeetCode Solution
In this guide, you will get 845. Longest Mountain in Array LeetCode Solution with the best time and space complexity. The solution to Longest Mountain in Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Longest Mountain in Array solution in C++
- Longest Mountain in Array solution in Java
- Longest Mountain in Array solution in Python
- Additional Resources
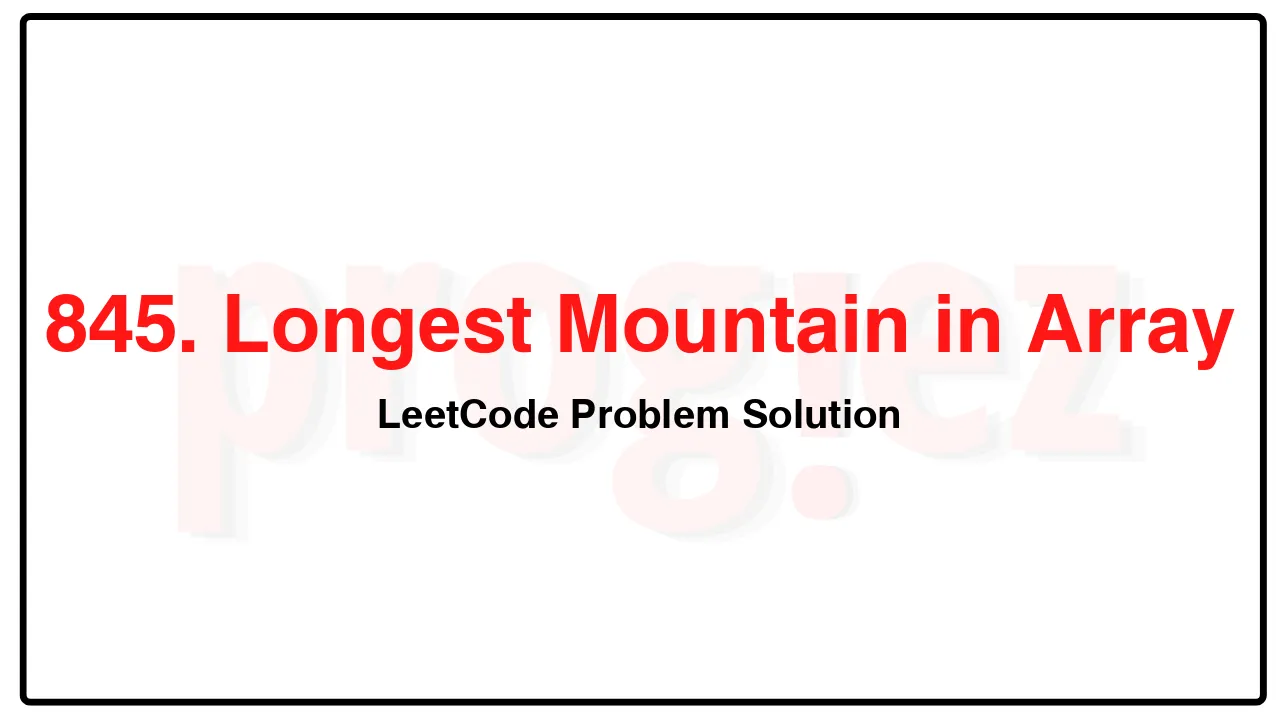
Problem Statement of Longest Mountain in Array
You may recall that an array arr is a mountain array if and only if:
arr.length >= 3
There exists some index i (0-indexed) with 0 < i < arr.length – 1 such that:
arr[0] < arr[1] < … < arr[i – 1] arr[i + 1] > … > arr[arr.length – 1]
Given an integer array arr, return the length of the longest subarray, which is a mountain. Return 0 if there is no mountain subarray.
Example 1:
Input: arr = [2,1,4,7,3,2,5]
Output: 5
Explanation: The largest mountain is [1,4,7,3,2] which has length 5.
Example 2:
Input: arr = [2,2,2]
Output: 0
Explanation: There is no mountain.
Constraints:
1 <= arr.length <= 104
0 <= arr[i] <= 104
Follow up:
Can you solve it using only one pass?
Can you solve it in O(1) space?
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
845. Longest Mountain in Array LeetCode Solution in C++
class Solution {
public:
int longestMountain(vector<int>& arr) {
int ans = 0;
for (int i = 0; i + 1 < arr.size();) {
while (i + 1 < arr.size() && arr[i] == arr[i + 1])
++i;
int increasing = 0;
int decreasing = 0;
while (i + 1 < arr.size() && arr[i] < arr[i + 1]) {
++increasing;
++i;
}
while (i + 1 < arr.size() && arr[i] > arr[i + 1]) {
++decreasing;
++i;
}
if (increasing > 0 && decreasing > 0)
ans = max(ans, increasing + decreasing + 1);
}
return ans;
}
};
/* code provided by PROGIEZ */
845. Longest Mountain in Array LeetCode Solution in Java
class Solution {
public int longestMountain(int[] arr) {
int ans = 0;
for (int i = 0; i + 1 < arr.length;) {
while (i + 1 < arr.length && arr[i] == arr[i + 1])
++i;
int increasing = 0;
int decreasing = 0;
while (i + 1 < arr.length && arr[i] < arr[i + 1]) {
++increasing;
++i;
}
while (i + 1 < arr.length && arr[i] > arr[i + 1]) {
++decreasing;
++i;
}
if (increasing > 0 && decreasing > 0)
ans = Math.max(ans, increasing + decreasing + 1);
}
return ans;
}
}
// code provided by PROGIEZ
845. Longest Mountain in Array LeetCode Solution in Python
class Solution:
def longestMountain(self, arr: list[int]) -> int:
ans = 0
i = 0
while i + 1 < len(arr):
while i + 1 < len(arr) and arr[i] == arr[i + 1]:
i += 1
increasing = 0
decreasing = 0
while i + 1 < len(arr) and arr[i] < arr[i + 1]:
increasing += 1
i += 1
while i + 1 < len(arr) and arr[i] > arr[i + 1]:
decreasing += 1
i += 1
if increasing > 0 and decreasing > 0:
ans = max(ans, increasing + decreasing + 1)
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.