1267. Count Servers that Communicate LeetCode Solution
In this guide, you will get 1267. Count Servers that Communicate LeetCode Solution with the best time and space complexity. The solution to Count Servers that Communicate problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Count Servers that Communicate solution in C++
- Count Servers that Communicate solution in Java
- Count Servers that Communicate solution in Python
- Additional Resources
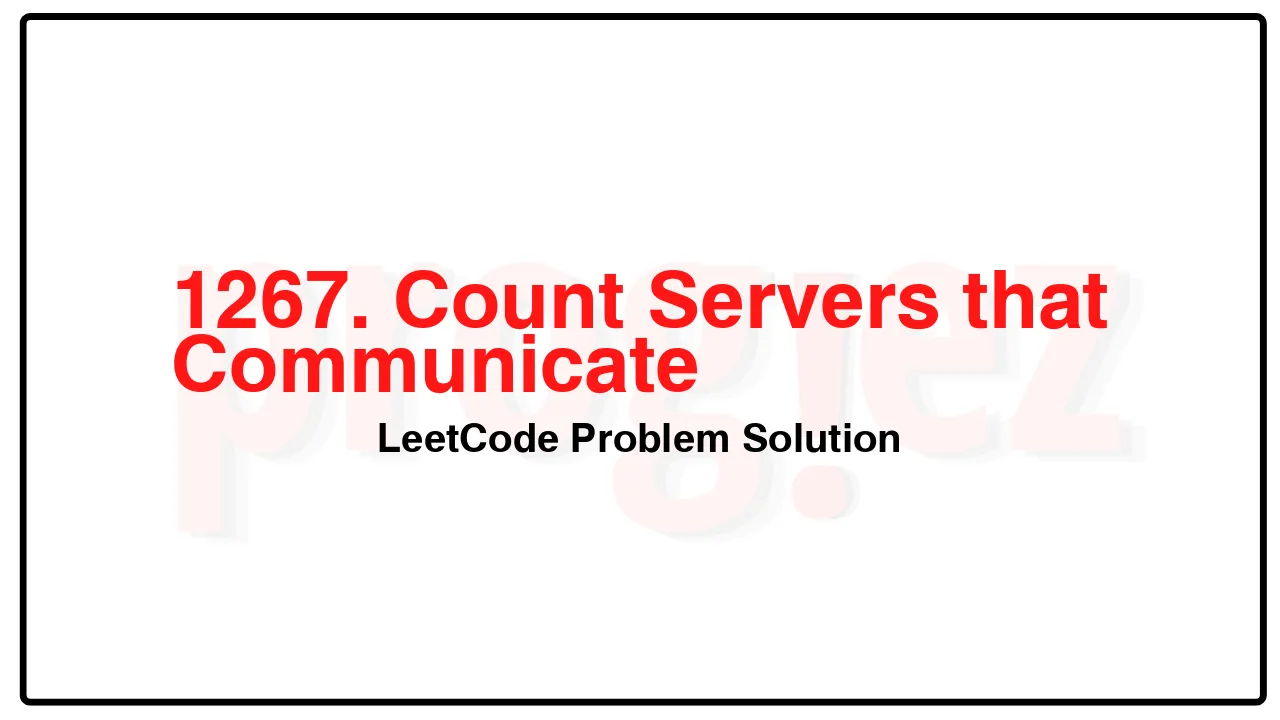
Problem Statement of Count Servers that Communicate
You are given a map of a server center, represented as a m * n integer matrix grid, where 1 means that on that cell there is a server and 0 means that it is no server. Two servers are said to communicate if they are on the same row or on the same column.
Return the number of servers that communicate with any other server.
Example 1:
Input: grid = [[1,0],[0,1]]
Output: 0
Explanation: No servers can communicate with others.
Example 2:
Input: grid = [[1,0],[1,1]]
Output: 3
Explanation: All three servers can communicate with at least one other server.
Example 3:
Input: grid = [[1,1,0,0],[0,0,1,0],[0,0,1,0],[0,0,0,1]]
Output: 4
Explanation: The two servers in the first row can communicate with each other. The two servers in the third column can communicate with each other. The server at right bottom corner can’t communicate with any other server.
Constraints:
m == grid.length
n == grid[i].length
1 <= m <= 250
1 <= n <= 250
grid[i][j] == 0 or 1
Complexity Analysis
- Time Complexity:
- Space Complexity:
1267. Count Servers that Communicate LeetCode Solution in C++
class Solution {
public:
int countServers(vector<vector<int>>& grid) {
const int m = grid.size();
const int n = grid[0].size();
int ans = 0;
vector<int> rows(m);
vector<int> cols(n);
for (int i = 0; i < m; ++i)
for (int j = 0; j < n; ++j)
if (grid[i][j] == 1) {
++rows[i];
++cols[j];
}
for (int i = 0; i < m; ++i)
for (int j = 0; j < n; ++j)
if (grid[i][j] == 1 && (rows[i] > 1 || cols[j] > 1))
++ans;
return ans;
}
};
/* code provided by PROGIEZ */
1267. Count Servers that Communicate LeetCode Solution in Java
class Solution {
public int countServers(int[][] grid) {
final int m = grid.length;
final int n = grid[0].length;
int ans = 0;
int[] rows = new int[m];
int[] cols = new int[n];
for (int i = 0; i < m; ++i)
for (int j = 0; j < n; ++j)
if (grid[i][j] == 1) {
++rows[i];
++cols[j];
}
for (int i = 0; i < m; ++i)
for (int j = 0; j < n; ++j)
if (grid[i][j] == 1 && (rows[i] > 1 || cols[j] > 1))
++ans;
return ans;
}
}
// code provided by PROGIEZ
1267. Count Servers that Communicate LeetCode Solution in Python
class Solution:
def countServers(self, grid: list[list[int]]) -> int:
m = len(grid)
n = len(grid[0])
ans = 0
rows = [0] * m
cols = [0] * n
for i in range(m):
for j in range(n):
if grid[i][j] == 1:
rows[i] += 1
cols[j] += 1
for i in range(m):
for j in range(n):
if grid[i][j] == 1 and (rows[i] > 1 or cols[j] > 1):
ans += 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.