423. Reconstruct Original Digits from English LeetCode Solution
In this guide, you will get 423. Reconstruct Original Digits from English LeetCode Solution with the best time and space complexity. The solution to Reconstruct Original Digits from English problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Reconstruct Original Digits from English solution in C++
- Reconstruct Original Digits from English solution in Java
- Reconstruct Original Digits from English solution in Python
- Additional Resources
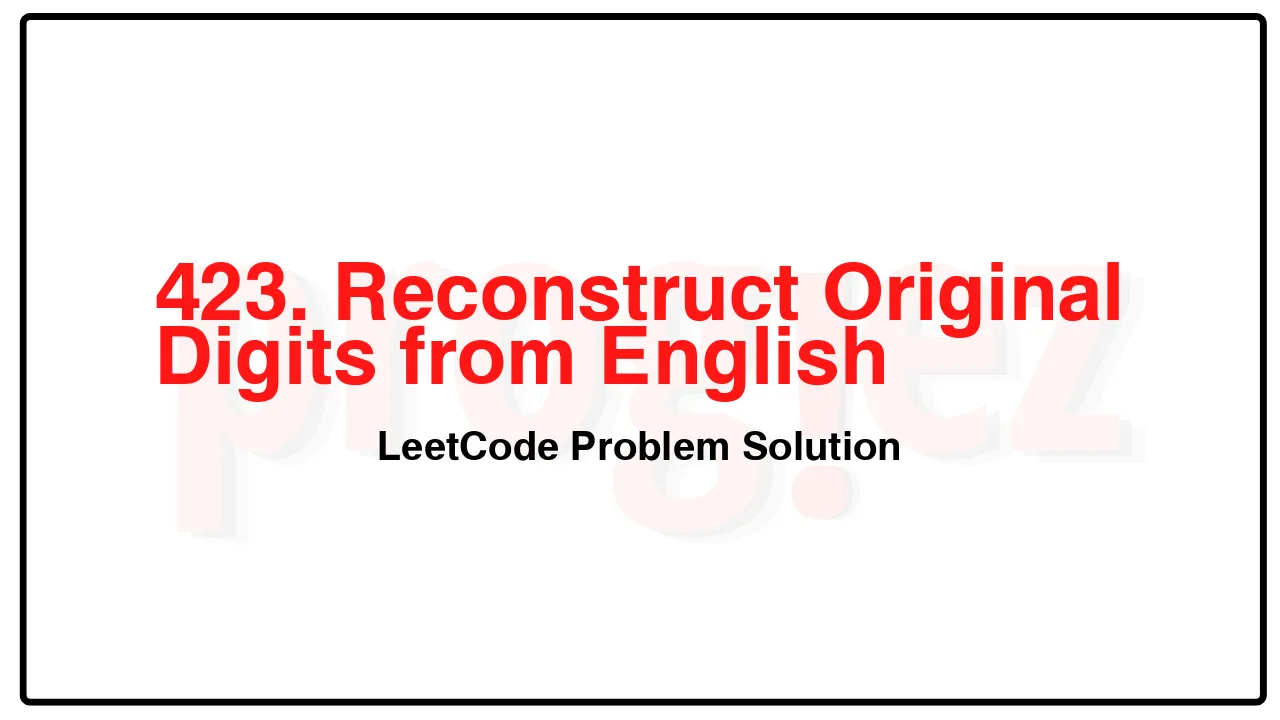
Problem Statement of Reconstruct Original Digits from English
Given a string s containing an out-of-order English representation of digits 0-9, return the digits in ascending order.
Example 1:
Input: s = “owoztneoer”
Output: “012”
Example 2:
Input: s = “fviefuro”
Output: “45”
Constraints:
1 <= s.length <= 105
s[i] is one of the characters ["e","g","f","i","h","o","n","s","r","u","t","w","v","x","z"].
s is guaranteed to be valid.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
423. Reconstruct Original Digits from English LeetCode Solution in C++
class Solution {
public:
string originalDigits(string s) {
string ans;
vector<int> count(10);
for (const char c : s) {
if (c == 'z')
++count[0];
if (c == 'o')
++count[1];
if (c == 'w')
++count[2];
if (c == 'h')
++count[3];
if (c == 'u')
++count[4];
if (c == 'f')
++count[5];
if (c == 'x')
++count[6];
if (c == 's')
++count[7];
if (c == 'g')
++count[8];
if (c == 'i')
++count[9];
}
count[1] -= count[0] + count[2] + count[4];
count[3] -= count[8];
count[5] -= count[4];
count[7] -= count[6];
count[9] -= count[5] + count[6] + count[8];
for (int i = 0; i < 10; ++i)
for (int j = 0; j < count[i]; ++j)
ans += i + '0';
return ans;
}
};
/* code provided by PROGIEZ */
423. Reconstruct Original Digits from English LeetCode Solution in Java
class Solution {
public String originalDigits(String s) {
StringBuilder sb = new StringBuilder();
int[] count = new int[10];
for (final char c : s.toCharArray()) {
if (c == 'z')
++count[0];
if (c == 'o')
++count[1];
if (c == 'w')
++count[2];
if (c == 'h')
++count[3];
if (c == 'u')
++count[4];
if (c == 'f')
++count[5];
if (c == 'x')
++count[6];
if (c == 's')
++count[7];
if (c == 'g')
++count[8];
if (c == 'i')
++count[9];
}
count[1] -= count[0] + count[2] + count[4];
count[3] -= count[8];
count[5] -= count[4];
count[7] -= count[6];
count[9] -= count[5] + count[6] + count[8];
for (int i = 0; i < 10; ++i)
for (int j = 0; j < count[i]; ++j)
sb.append(i);
return sb.toString();
}
}
// code provided by PROGIEZ
423. Reconstruct Original Digits from English LeetCode Solution in Python
class Solution:
def originalDigits(self, s: str) -> str:
count = [0] * 10
for c in s:
if c == 'z':
count[0] += 1
if c == 'o':
count[1] += 1
if c == 'w':
count[2] += 1
if c == 'h':
count[3] += 1
if c == 'u':
count[4] += 1
if c == 'f':
count[5] += 1
if c == 'x':
count[6] += 1
if c == 's':
count[7] += 1
if c == 'g':
count[8] += 1
if c == 'i':
count[9] += 1
count[1] -= count[0] + count[2] + count[4]
count[3] -= count[8]
count[5] -= count[4]
count[7] -= count[6]
count[9] -= count[5] + count[6] + count[8]
return ''.join(chr(i + ord('0')) for i, c in enumerate(count)
for _ in range(c))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.