594. Longest Harmonious Subsequence LeetCode Solution
In this guide, you will get 594. Longest Harmonious Subsequence LeetCode Solution with the best time and space complexity. The solution to Longest Harmonious Subsequence problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Longest Harmonious Subsequence solution in C++
- Longest Harmonious Subsequence solution in Java
- Longest Harmonious Subsequence solution in Python
- Additional Resources
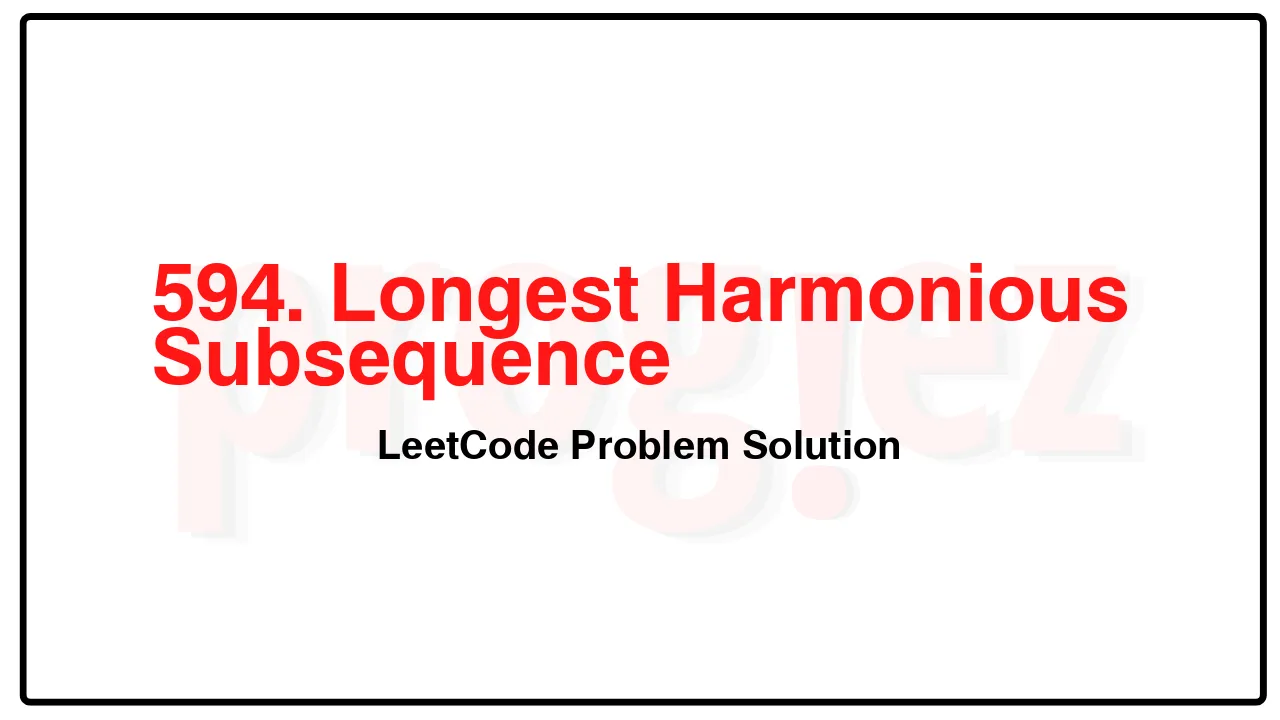
Problem Statement of Longest Harmonious Subsequence
We define a harmonious array as an array where the difference between its maximum value and its minimum value is exactly 1.
Given an integer array nums, return the length of its longest harmonious subsequence among all its possible subsequences.
Example 1:
Input: nums = [1,3,2,2,5,2,3,7]
Output: 5
Explanation:
The longest harmonious subsequence is [3,2,2,2,3].
Example 2:
Input: nums = [1,2,3,4]
Output: 2
Explanation:
The longest harmonious subsequences are [1,2], [2,3], and [3,4], all of which have a length of 2.
Example 3:
Input: nums = [1,1,1,1]
Output: 0
Explanation:
No harmonic subsequence exists.
Constraints:
1 <= nums.length <= 2 * 104
-109 <= nums[i] <= 109
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
594. Longest Harmonious Subsequence LeetCode Solution in C++
class Solution {
public:
int findLHS(vector<int>& nums) {
int ans = 0;
unordered_map<int, int> count;
for (const int num : nums)
++count[num];
for (const auto& [num, freq] : count)
if (const auto it = count.find(num + 1); it != count.cend())
ans = max(ans, freq + it->second);
return ans;
}
};
/* code provided by PROGIEZ */
594. Longest Harmonious Subsequence LeetCode Solution in Java
class Solution {
public int findLHS(int[] nums) {
int ans = 0;
Map<Integer, Integer> count = new HashMap<>();
for (final int num : nums)
count.merge(num, 1, Integer::sum);
for (final int num : count.keySet())
if (count.containsKey(num + 1))
ans = Math.max(ans, count.get(num) + count.get(num + 1));
return ans;
}
}
// code provided by PROGIEZ
594. Longest Harmonious Subsequence LeetCode Solution in Python
class Solution:
def findLHS(self, nums: list[int]) -> int:
ans = 0
count = collections.Counter(nums)
for num, freq in count.items():
if num + 1 in count:
ans = max(ans, freq + count[num + 1])
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.