392. Is Subsequence LeetCode Solution
In this guide, you will get 392. Is Subsequence LeetCode Solution with the best time and space complexity. The solution to Is Subsequence problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Is Subsequence solution in C++
- Is Subsequence solution in Java
- Is Subsequence solution in Python
- Additional Resources
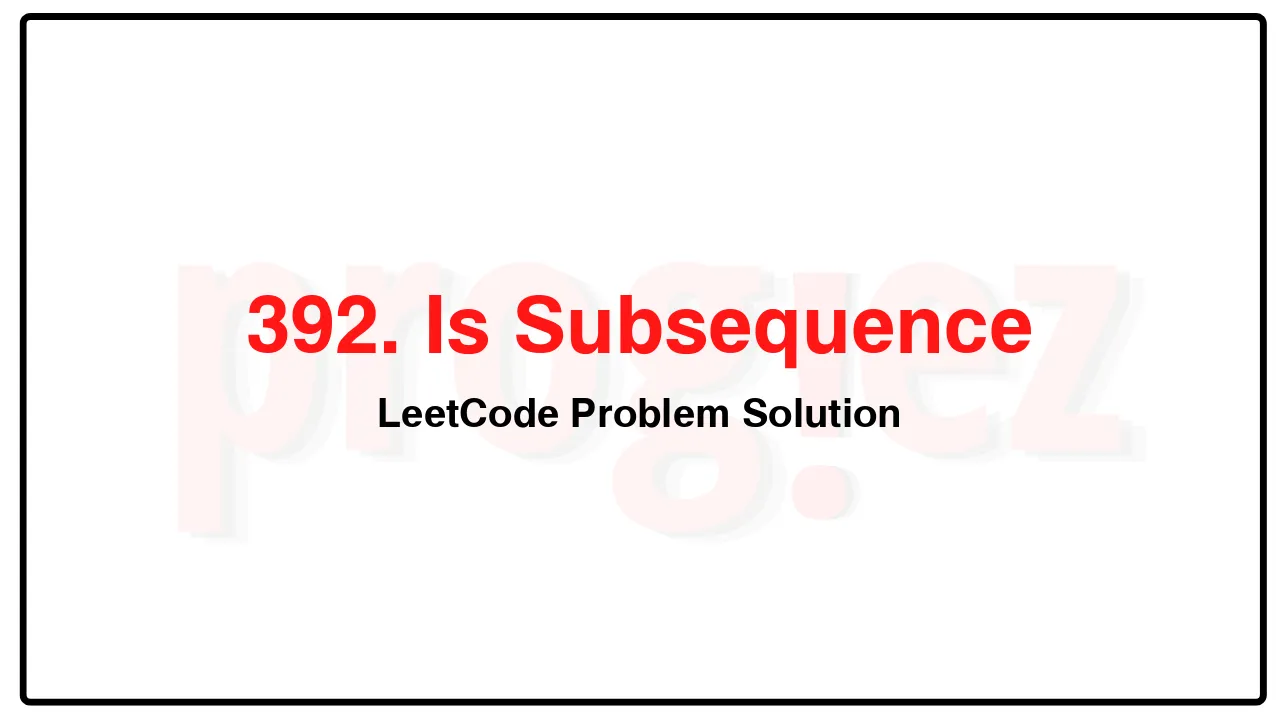
Problem Statement of Is Subsequence
Given two strings s and t, return true if s is a subsequence of t, or false otherwise.
A subsequence of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., “ace” is a subsequence of “abcde” while “aec” is not).
Example 1:
Input: s = “abc”, t = “ahbgdc”
Output: true
Example 2:
Input: s = “axc”, t = “ahbgdc”
Output: false
Constraints:
0 <= s.length <= 100
0 <= t.length = 109, and you want to check one by one to see if t has its subsequence. In this scenario, how would you change your code?
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
392. Is Subsequence LeetCode Solution in C++
class Solution {
public:
bool isSubsequence(string s, string t) {
if (s.empty())
return true;
int i = 0;
for (const char c : t)
if (s[i] == c && ++i == s.length())
return true;
return false;
}
};
/* code provided by PROGIEZ */
392. Is Subsequence LeetCode Solution in Java
class Solution {
public boolean isSubsequence(String s, String t) {
if (s.isEmpty())
return true;
int i = 0;
for (final char c : t.toCharArray())
if (s.charAt(i) == c && ++i == s.length())
return true;
return false;
}
}
// code provided by PROGIEZ
392. Is Subsequence LeetCode Solution in Python
class Solution:
def isSubsequence(self, s: str, t: str) -> bool:
if not s:
return True
i = 0
for c in t:
if s[i] == c:
i += 1
if i == len(s):
return True
return False
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.