300. Longest Increasing Subsequence LeetCode Solution
In this guide, you will get 300. Longest Increasing Subsequence LeetCode Solution with the best time and space complexity. The solution to Longest Increasing Subsequence problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Longest Increasing Subsequence solution in C++
- Longest Increasing Subsequence solution in Java
- Longest Increasing Subsequence solution in Python
- Additional Resources
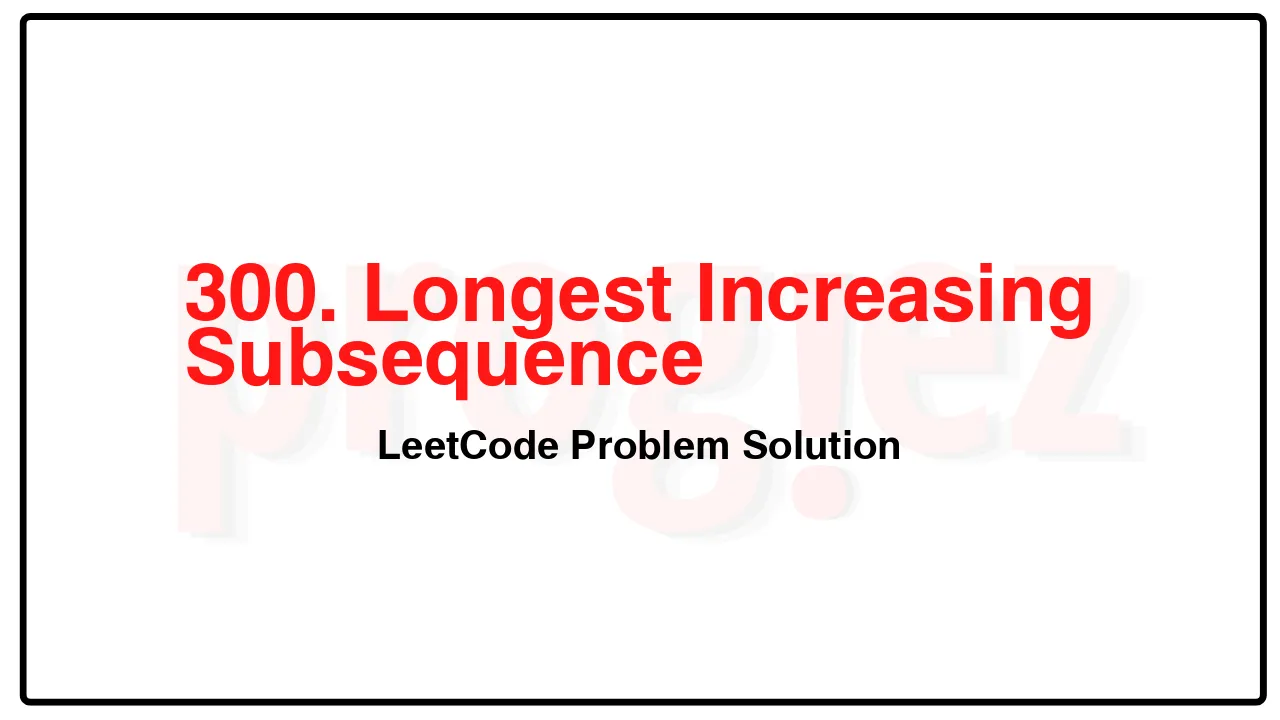
Problem Statement of Longest Increasing Subsequence
Given an integer array nums, return the length of the longest strictly increasing subsequence.
Example 1:
Input: nums = [10,9,2,5,3,7,101,18]
Output: 4
Explanation: The longest increasing subsequence is [2,3,7,101], therefore the length is 4.
Example 2:
Input: nums = [0,1,0,3,2,3]
Output: 4
Example 3:
Input: nums = [7,7,7,7,7,7,7]
Output: 1
Constraints:
1 <= nums.length <= 2500
-104 <= nums[i] <= 104
Follow up: Can you come up with an algorithm that runs in O(n log(n)) time complexity?
Complexity Analysis
- Time Complexity: O(n^2)
- Space Complexity: O(n)
300. Longest Increasing Subsequence LeetCode Solution in C++
class Solution {
public:
int lengthOfLIS(vector<int>& nums) {
if (nums.empty())
return 0;
// dp[i] := the length of LIS ending in nums[i]
vector<int> dp(nums.size(), 1);
for (int i = 1; i < nums.size(); ++i)
for (int j = 0; j < i; ++j)
if (nums[j] < nums[i])
dp[i] = max(dp[i], dp[j] + 1);
return ranges::max(dp);
}
};
/* code provided by PROGIEZ */
300. Longest Increasing Subsequence LeetCode Solution in Java
class Solution {
public int lengthOfLIS(int[] nums) {
if (nums.length == 0)
return 0;
// dp[i] := the length of LIS ending in nums[i]
int[] dp = new int[nums.length];
Arrays.fill(dp, 1);
for (int i = 1; i < nums.length; ++i)
for (int j = 0; j < i; ++j)
if (nums[j] < nums[i])
dp[i] = Math.max(dp[i], dp[j] + 1);
return Arrays.stream(dp).max().getAsInt();
}
}
// code provided by PROGIEZ
300. Longest Increasing Subsequence LeetCode Solution in Python
class Solution:
def lengthOfLIS(self, nums: list[int]) -> int:
if not nums:
return 0
# dp[i] the length of LIS ending in nums[i]
dp = [1] * len(nums)
for i in range(1, len(nums)):
for j in range(i):
if nums[j] < nums[i]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.