899. Orderly Queue LeetCode Solution
In this guide, you will get 899. Orderly Queue LeetCode Solution with the best time and space complexity. The solution to Orderly Queue problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Orderly Queue solution in C++
- Orderly Queue solution in Java
- Orderly Queue solution in Python
- Additional Resources
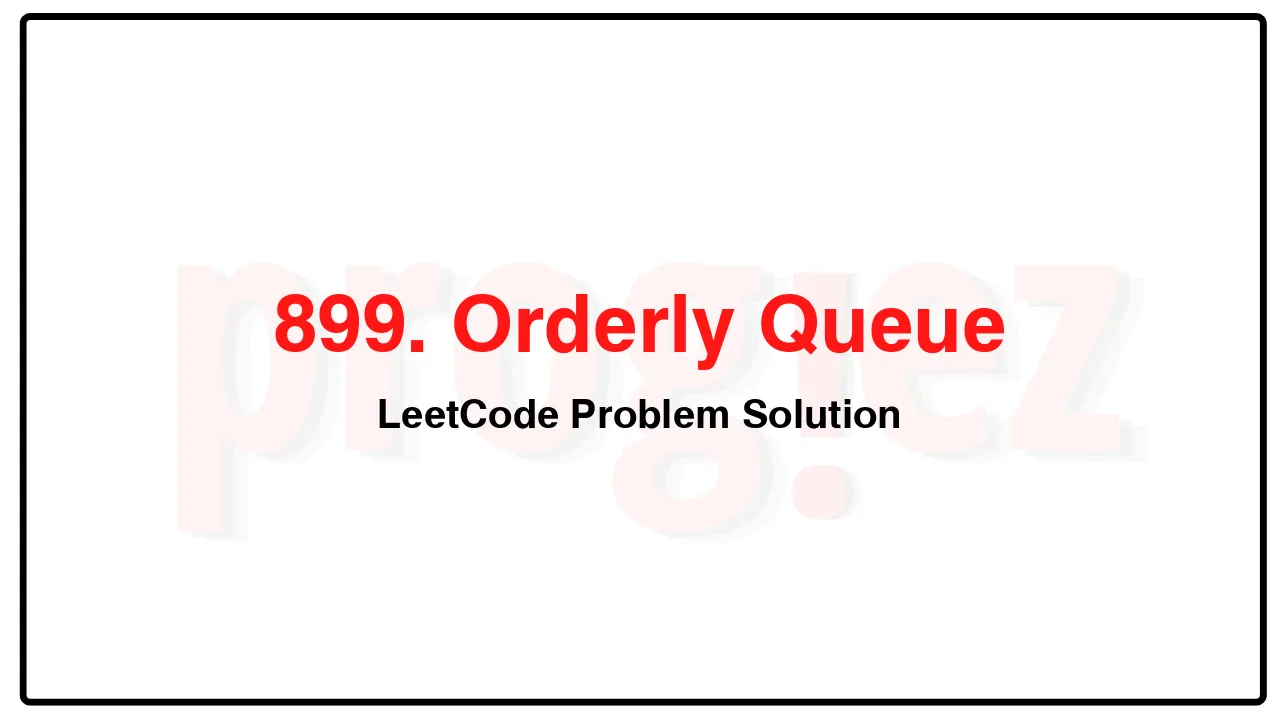
Problem Statement of Orderly Queue
You are given a string s and an integer k. You can choose one of the first k letters of s and append it at the end of the string.
Return the lexicographically smallest string you could have after applying the mentioned step any number of moves.
Example 1:
Input: s = “cba”, k = 1
Output: “acb”
Explanation:
In the first move, we move the 1st character ‘c’ to the end, obtaining the string “bac”.
In the second move, we move the 1st character ‘b’ to the end, obtaining the final result “acb”.
Example 2:
Input: s = “baaca”, k = 3
Output: “aaabc”
Explanation:
In the first move, we move the 1st character ‘b’ to the end, obtaining the string “aacab”.
In the second move, we move the 3rd character ‘c’ to the end, obtaining the final result “aaabc”.
Constraints:
1 <= k <= s.length <= 1000
s consist of lowercase English letters.
Complexity Analysis
- Time Complexity: O(n^2)
- Space Complexity: O(n)
899. Orderly Queue LeetCode Solution in C++
class Solution {
public:
string orderlyQueue(string s, int k) {
if (k > 1) {
ranges::sort(s);
return s;
}
string ans = s;
for (int i = 1; i < s.length(); ++i)
ans = min(ans, s.substr(i) + s.substr(0, i));
return ans;
}
};
/* code provided by PROGIEZ */
899. Orderly Queue LeetCode Solution in Java
class Solution {
public String orderlyQueue(String s, int k) {
if (k > 1) {
char[] chars = s.toCharArray();
Arrays.sort(chars);
return String.valueOf(chars);
}
String ans = s;
for (int i = 1; i < s.length(); ++i) {
String t = s.substring(i) + s.substring(0, i);
if (ans.compareTo(t) > 0)
ans = t;
}
return ans;
}
}
// code provided by PROGIEZ
899. Orderly Queue LeetCode Solution in Python
class Solution:
def orderlyQueue(self, s: str, k: int) -> str:
return (''.join(sorted(s)) if k > 1
else min(s[i:] + s[:i] for i in range(len(s))))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.