524. Longest Word in Dictionary through Deleting LeetCode Solution
In this guide, you will get 524. Longest Word in Dictionary through Deleting LeetCode Solution with the best time and space complexity. The solution to Longest Word in Dictionary through Deleting problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Longest Word in Dictionary through Deleting solution in C++
- Longest Word in Dictionary through Deleting solution in Java
- Longest Word in Dictionary through Deleting solution in Python
- Additional Resources
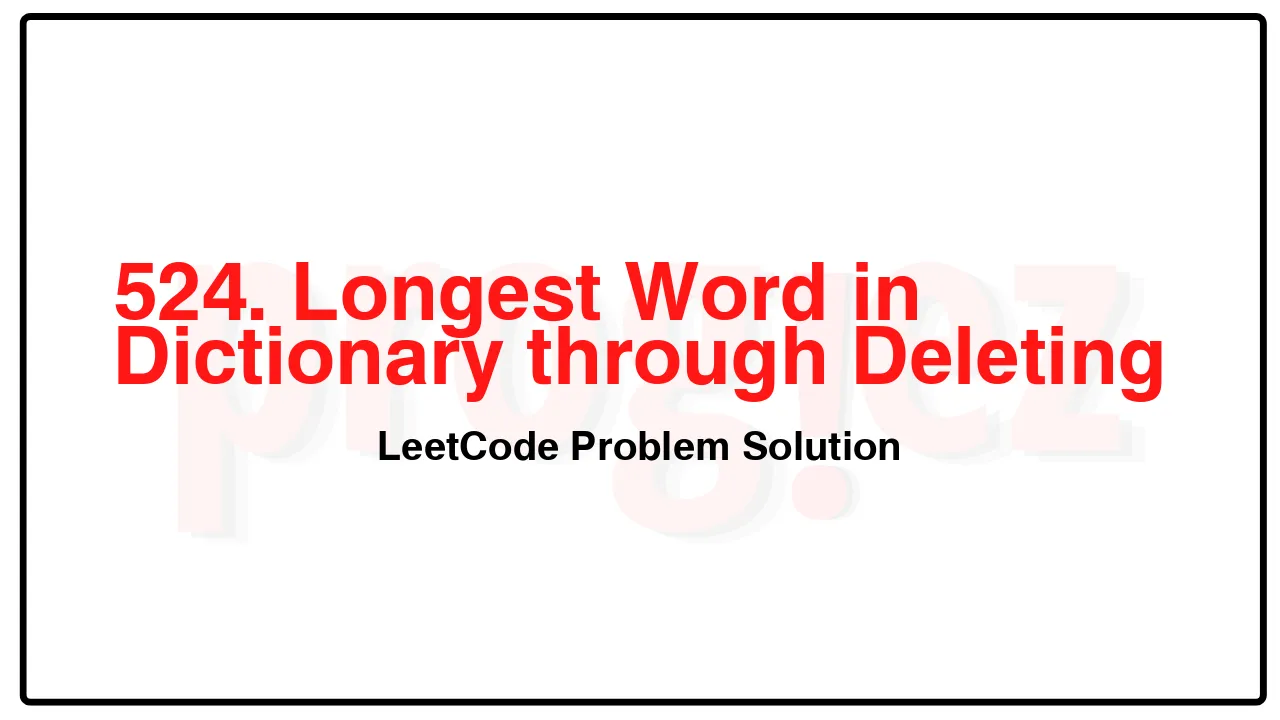
Problem Statement of Longest Word in Dictionary through Deleting
Given a string s and a string array dictionary, return the longest string in the dictionary that can be formed by deleting some of the given string characters. If there is more than one possible result, return the longest word with the smallest lexicographical order. If there is no possible result, return the empty string.
Example 1:
Input: s = “abpcplea”, dictionary = [“ale”,”apple”,”monkey”,”plea”]
Output: “apple”
Example 2:
Input: s = “abpcplea”, dictionary = [“a”,”b”,”c”]
Output: “a”
Constraints:
1 <= s.length <= 1000
1 <= dictionary.length <= 1000
1 <= dictionary[i].length <= 1000
s and dictionary[i] consist of lowercase English letters.
Complexity Analysis
- Time Complexity: O(|\texttt{d}||\texttt{s}|)
- Space Complexity: O(|\texttt{s}|)
524. Longest Word in Dictionary through Deleting LeetCode Solution in C++
class Solution {
public:
string findLongestWord(string s, vector<string>& d) {
string ans;
for (const string& word : d)
if (isSubsequence(word, s))
if (word.length() > ans.length() ||
word.length() == ans.length() && word.compare(ans) < 0)
ans = word;
return ans;
}
private:
// Returns true if a is a subsequence of b.
bool isSubsequence(const string& a, const string& b) {
int i = 0;
for (const char c : b)
if (i < a.length() && c == a[i])
++i;
return i == a.length();
};
};
/* code provided by PROGIEZ */
524. Longest Word in Dictionary through Deleting LeetCode Solution in Java
class Solution {
public String findLongestWord(String s, List<String> d) {
String ans = "";
for (final String word : d)
if (isSubsequence(word, s))
if (word.length() > ans.length() ||
word.length() == ans.length() && word.compareTo(ans) < 0)
ans = word;
return ans;
}
// Returns true if a is a subsequence of b.
private boolean isSubsequence(final String a, final String b) {
int i = 0;
for (final char c : b.toCharArray())
if (i < a.length() && c == a.charAt(i))
++i;
return i == a.length();
}
}
// code provided by PROGIEZ
524. Longest Word in Dictionary through Deleting LeetCode Solution in Python
class Solution:
def findLongestWord(self, s: str, d: list[str]) -> str:
ans = ''
for word in d:
i = 0
for c in s:
if i < len(word) and c == word[i]:
i += 1
if i == len(word):
if len(word) > len(ans) or len(word) == len(ans) and word < ans:
ans = word
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.