766. Toeplitz Matrix LeetCode Solution
In this guide, you will get 766. Toeplitz Matrix LeetCode Solution with the best time and space complexity. The solution to Toeplitz Matrix problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Toeplitz Matrix solution in C++
- Toeplitz Matrix solution in Java
- Toeplitz Matrix solution in Python
- Additional Resources
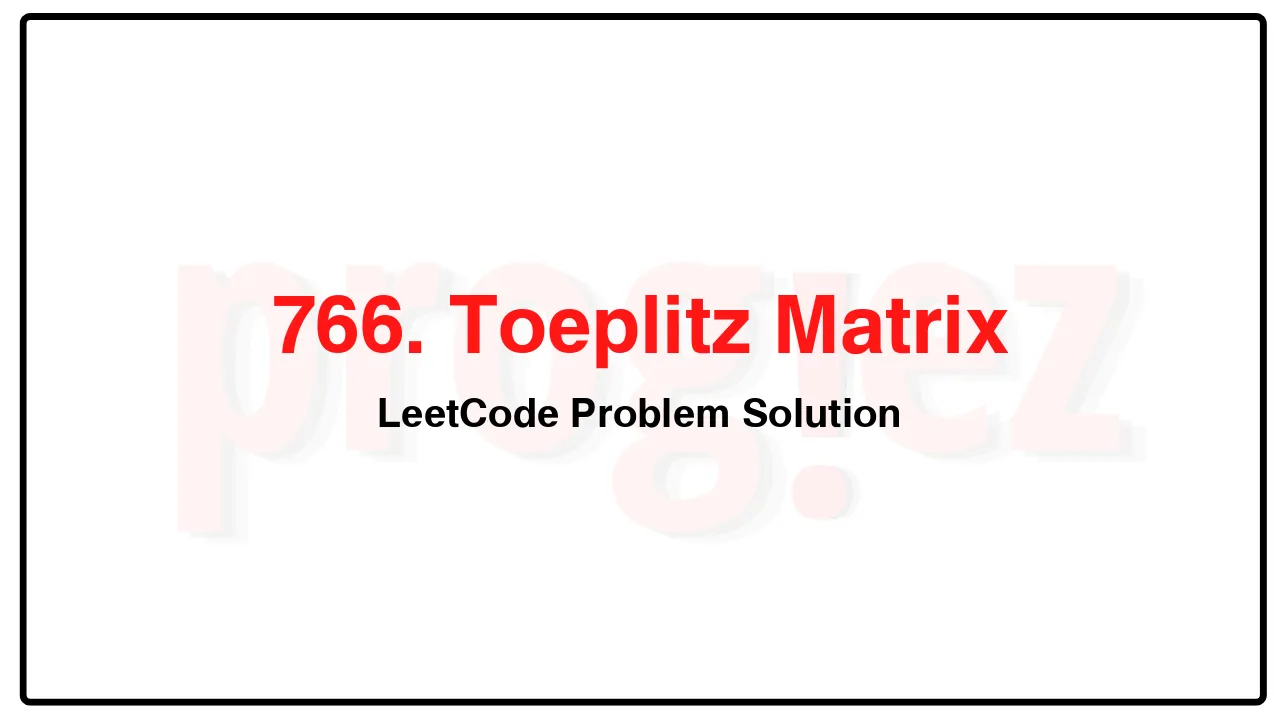
Problem Statement of Toeplitz Matrix
Given an m x n matrix, return true if the matrix is Toeplitz. Otherwise, return false.
A matrix is Toeplitz if every diagonal from top-left to bottom-right has the same elements.
Example 1:
Input: matrix = [[1,2,3,4],[5,1,2,3],[9,5,1,2]]
Output: true
Explanation:
In the above grid, the diagonals are:
“[9]”, “[5, 5]”, “[1, 1, 1]”, “[2, 2, 2]”, “[3, 3]”, “[4]”.
In each diagonal all elements are the same, so the answer is True.
Example 2:
Input: matrix = [[1,2],[2,2]]
Output: false
Explanation:
The diagonal “[1, 2]” has different elements.
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 20
0 <= matrix[i][j] <= 99
Follow up:
What if the matrix is stored on disk, and the memory is limited such that you can only load at most one row of the matrix into the memory at once?
What if the matrix is so large that you can only load up a partial row into the memory at once?
Complexity Analysis
- Time Complexity: O(mn)
- Space Complexity: O(1)
766. Toeplitz Matrix LeetCode Solution in C++
class Solution {
public:
bool isToeplitzMatrix(vector<vector<int>>& matrix) {
for (int i = 0; i + 1 < matrix.size(); ++i)
for (int j = 0; j + 1 < matrix[0].size(); ++j)
if (matrix[i][j] != matrix[i + 1][j + 1])
return false;
return true;
}
};
/* code provided by PROGIEZ */
766. Toeplitz Matrix LeetCode Solution in Java
class Solution {
public boolean isToeplitzMatrix(int[][] matrix) {
for (int i = 0; i + 1 < matrix.length; ++i)
for (int j = 0; j + 1 < matrix[0].length; ++j)
if (matrix[i][j] != matrix[i + 1][j + 1])
return false;
return true;
}
}
// code provided by PROGIEZ
766. Toeplitz Matrix LeetCode Solution in Python
class Solution:
def isToeplitzMatrix(self, matrix: list[list[int]]) -> bool:
for i in range(len(matrix) - 1):
for j in range(len(matrix[0]) - 1):
if matrix[i][j] != matrix[i + 1][j + 1]:
return False
return True
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.