1380. Lucky Numbers in a Matrix LeetCode Solution
In this guide, you will get 1380. Lucky Numbers in a Matrix LeetCode Solution with the best time and space complexity. The solution to Lucky Numbers in a Matrix problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Lucky Numbers in a Matrix solution in C++
- Lucky Numbers in a Matrix solution in Java
- Lucky Numbers in a Matrix solution in Python
- Additional Resources
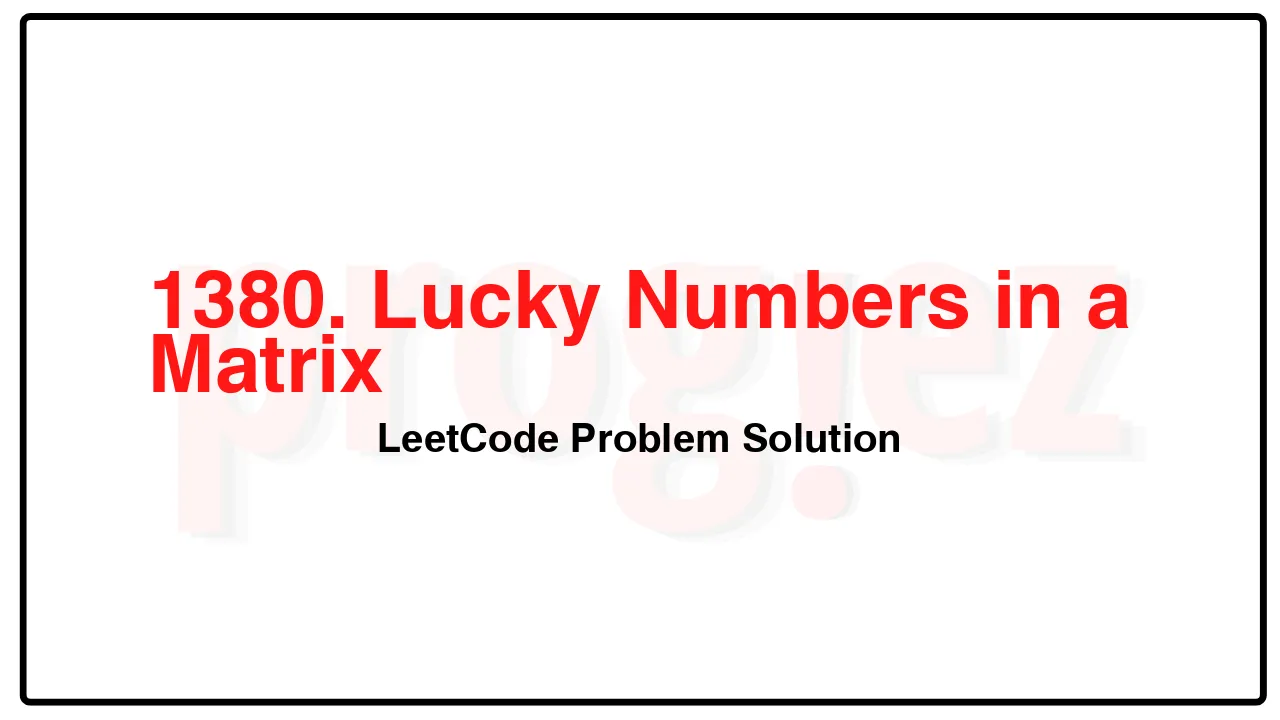
Problem Statement of Lucky Numbers in a Matrix
Given an m x n matrix of distinct numbers, return all lucky numbers in the matrix in any order.
A lucky number is an element of the matrix such that it is the minimum element in its row and maximum in its column.
Example 1:
Input: matrix = [[3,7,8],[9,11,13],[15,16,17]]
Output: [15]
Explanation: 15 is the only lucky number since it is the minimum in its row and the maximum in its column.
Example 2:
Input: matrix = [[1,10,4,2],[9,3,8,7],[15,16,17,12]]
Output: [12]
Explanation: 12 is the only lucky number since it is the minimum in its row and the maximum in its column.
Example 3:
Input: matrix = [[7,8],[1,2]]
Output: [7]
Explanation: 7 is the only lucky number since it is the minimum in its row and the maximum in its column.
Constraints:
m == mat.length
n == mat[i].length
1 <= n, m <= 50
1 <= matrix[i][j] <= 105.
All elements in the matrix are distinct.
Complexity Analysis
- Time Complexity: O(mn)
- Space Complexity: O(1)
1380. Lucky Numbers in a Matrix LeetCode Solution in C++
class Solution {
public:
vector<int> luckyNumbers(vector<vector<int>>& matrix) {
for (const vector<int>& row : matrix) {
const int minIndex = distance(row.begin(), ranges::min_element(row));
if (row[minIndex] == maxNumOfColumn(matrix, minIndex))
return {row[minIndex]};
}
return {};
}
private:
int maxNumOfColumn(const vector<vector<int>>& matrix, int j) {
int res = 0;
for (int i = 0; i < matrix.size(); ++i)
res = max(res, matrix[i][j]);
return res;
}
};
/* code provided by PROGIEZ */
1380. Lucky Numbers in a Matrix LeetCode Solution in Java
class Solution {
public List<Integer> luckyNumbers(int[][] matrix) {
for (int[] row : matrix) {
final int minIndex = getMinIndex(row);
if (row[minIndex] == maxNumOfColumn(matrix, minIndex))
return List.of(row[minIndex]);
}
return new ArrayList<>();
}
private int getMinIndex(int[] row) {
int minIndex = 0;
for (int j = 0; j < row.length; ++j)
if (row[j] < row[minIndex])
minIndex = j;
return minIndex;
}
private int maxNumOfColumn(int[][] matrix, int j) {
int res = 0;
for (int i = 0; i < matrix.length; ++i)
res = Math.max(res, matrix[i][j]);
return res;
}
}
// code provided by PROGIEZ
1380. Lucky Numbers in a Matrix LeetCode Solution in Python
class Solution:
def luckyNumbers(self, matrix: list[list[int]]) -> list[int]:
for row in matrix:
minIndex = row.index(min(row))
if row[minIndex] == max(list(zip(*matrix))[minIndex]):
return [row[minIndex]]
return []
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.