Programming DSA using Python Nptel Week 2 Assignment Answers
Are you looking for the Programming, Data Structures and Algorithms using Python NPTEL Week 2 Assignment Answers 2024? You’re in the right place! This guide provides detailed solutions to the Week 2 assignment questions, covering key concepts in Python programming, data structures, and algorithms.
Table of Contents
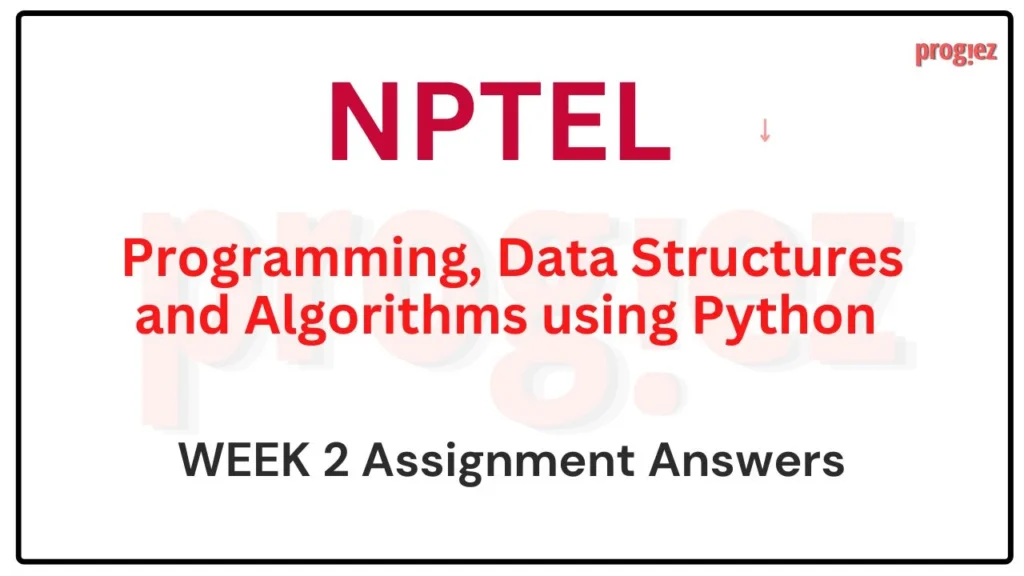
Programming DSA using Python Week 2 Assignment Answers (July-Dec 2024)
Q1.One of the following 10 statements generates an error. Which one? (Your answer should be a number between 1 and 10.)
x = [[3,5],”mimsy”,2,”borogove”,1] # Statement 1
y = x[0:50] # Statement 2
z = y # Statement 3
w = x # Statement 4
x[1] = x[1][:5] + ‘ery’ # Statement 5
y[1] = 4 # Statement 6
w[1][:3] = ‘fea’ # Statement 7
z[4] = 42 # Statement 8
x[0][0] = 5555 # Statement 9
a = (x[3][1] == 1) # Statement 10
Answer: 7
Q2.Consider the following lines of Python code.
b = [43,99,65,105,4]
a = b[2:]
d = b[1:]
c = b
d[1] = 95
b[2] = 47
c[3] = 73
Which of the following holds at the end of this code?
a[0] == 47, b[3] == 73, c[3] == 73, d[1] == 47
a[0] == 65, b[3] == 105, c[3] == 73, d[1] == 95
a[0] == 65, b[3] == 73, c[3] == 73, d[1] == 95
a[0] == 95, b[3] == 73, c[3] == 73, d[1] == 95
Answer: a[0] == 65, b[3] == 73, c[3] == 73, d[1] == 95
For answers or latest updates join our telegram channel: Click here to join
These are Programming DSA using Python Week 2 Assignment Answers
Q3. What is the value of endmsg after executing the following lines?
startmsg = “anaconda”
endmsg = “”
for i in range(1,1+len(startmsg)):
endmsg = endmsg + startmsg[-i]
Answer: “adnocana”
Q4. What is the value of mylist after the following lines are executed?
def mystery(l):
l = l[2:]
return(l)
mylist = [7,11,13,17,19,21]
mystery(mylist)
Answer: [7,11,13,17,19,21]
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Programming, Data Structures and Algorithms using Python NPTEL All weeks: Click Here
More Nptel Courses: https://progiez.com/nptel-assignment-answers
Programming DSA using Python Week 2 Assignment Answers (JULY-DEC 2023)
Course Link: Click Here
These are Programming Data Structure And Algorithms Assignment 2 Answers
Q1. One of the following 10 statements generates an error. Which one? (Your answer should be a number between 1 and 10.)
x = [“slithy”,[7,10,12],2,”tove”,1] # Statement 1
y = x[0:50] # Statement 2
z = y # Statement 3
w = x # Statement 4
x[0] = x[0][:5] + ‘ery’ # Statement 5
y[2] = 4 # Statement 6
z[4] = 42 # Statement 7
w[0][:3] = ‘fea’ # Statement 8
x[1][0] = 5555 # Statement 9
a = (x[4][1] == 1) # Statement 10
Answer: 8
Q2. Consider the following lines of Python code.
b = [23,44,87,100]
a = b[1:]
d = b[2:]
c = b
d[0] = 97
c[2] = 77
Which of the following holds at the end of this code?
a[1] == 77, b[2] == 77, c[2] == 77, d[0] == 97
a[1] == 87, b[2] == 87, c[2] == 77, d[0] == 97
a[1] == 87, b[2] == 77, c[2] == 77, d[0] == 97
a[1] == 97, b[2] == 77, c[2] == 77, d[0] == 97
Answer: a[1] == 87, b[2] == 77, c[2] == 77, d[0] == 97
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Q3. What is the value of endmsg after executing the following lines?
startmsg = “python”
endmsg = “”
for i in range(1,1+len(startmsg)):
endmsg = startmsg[-i] + endmsg
Answer: python
Q4. What is the value of mylist after the following lines are executed?
def mystery(l):
l = l[1:]
return()
mylist = [7,11,13]
mystery(mylist)
Answer: [7,11,13]
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Assignment Questions
Question 1
A positive integer m is a prime product if it can be written as p×q, where p and q are both primes.
Write a Python function primeproduct(m) that takes an integer m as input and returns True if m is a prime product and False otherwise. (If m is not positive, your function should return False.)
Here are some examples of how your function should work.
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Write a function delchar(s,c) that takes as input strings s and c, where c has length 1 (i.e., a single character), and returns the string obtained by deleting all occurrences of c in s. If c has length other than 1, the function should return s
Here are some examples to show how your function should work.
Write a function shuffle(l1,l2) that takes as input two lists, 11 and l2, and returns a list consisting of the first element in l1, then the first element in l2, then the second element in l1, then the second element in l2, and so on. If the two lists are not of equal length, the remaining elements of the longer list are appended at the end of the shuffled output.
Here are some examples to show how your function should work.
Answer:
def is_prime(num):
if num <= 1:
return False
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
return False
return True
def primeproduct(m):
if m <= 0:
return False
for i in range(2, m):
if m % i == 0 and is_prime(i) and is_prime(m // i):
return True
return False
def delchar(s, c):
if len(c) != 1:
return s
return s.replace(c, '')
def shuffle(l1, l2):
result = []
min_len = min(len(l1), len(l2))
for i in range(min_len):
result.append(l1[i])
result.append(l2[i])
result.extend(l1[min_len:])
result.extend(l2[min_len:])
return result
These are Nptel Programming DSA using Python Week 2 Assignment Answers
More Weeks of Programming Data Structure And Algorithms Using Python: Click here
More Nptel Courses: Click here
Programming, DSA using Python Week 2 Assignment Answers (JAN-APR 2023)
Course Link: Click Here
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Q1. One of the following 10 statements generates an error. Which one? (Your answer should be a number between 1 and 10.)
x = ["sun",[17],2,"king",[3,4]] # Statement 1
y = x[0:8] # Statement 2
z = x # Statement 3
w = y # Statement 4
z[0] = 0 # Statement 5
y[0] = y[0][0:3] + 'k' # Statement 6
y[1][1:3] = [5,8] # Statement 7
x[0] = x[0][1:3] # Statement 8
w[4][0] = 1000 # Statement 9
a = (x[4][1] == 4) # Statement 10
Answer: 8
Q2. Consider the following lines of Python code.
x = [589,'big',397,'bash']
y = x[2:]
u = x
w = y
w = w[0:]
w[0] = 357
x[2:3] = [487]
These are Nptel Programming DSA using Python Week 2 Assignment Answers
After these execute, which of the following is correct?
a. x[2] == 487, y[0] == 397, u[2] == 487, w[0] == 357
b. x[2] == 487, y[0] == 357, u[2] == 487, w[0] == 357
c. x[2] == 487, y[0] == 397, u[2] == 397, w[0] == 357
d. x[2] == 487, y[0] == 357, u[2] == 397, w[0] == 357
Answer: a. x[2] == 487, y[0] == 397, u[2] == 487, w[0] == 357
These are Programming Data Structure And Algorithms Assignment 2 Answers
Q3. What is the value of second after executing the following lines?
first = "kaleidoscope"
second = ""
for i in range(len(first)-1,-1,-2):
second = first[i]+second
Answer: ‘aedsoe’
Q4. What is the value of list1 after the following lines are executed?
def mystery(l):
l[0:2] = l[3:5]
return()
list1 = [34,17,12,88,53,97,62]
mystery(list1)
Answer: [88, 53, 12, 88, 53, 97, 62]
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Assignment Question
Question 1
1. A positive integer m can be partitioned as primes if it can be written as p + q where p > 0, q > 0 and both p and q are prime numbers.
Write a Python function primepartition(m) that takes an integer m as input and returns True if m can be partitioned as primes and False otherwise. (If m is not positive, your function should return False.)
Here are some examples of how your function should work.
>>> primepartition(7)
True
>>> primepartition(185)
False
>>> primepartition(3432)
True
These are Programming Data Structure And Algorithms Assignment 2 Answers
2. Write a function matched(s) that takes as input a string s and checks if the brackets “(” and “)” in s are matched: that is, every “(” has a matching “)” after it and every “)” has a matching “(” before it. Your function should ignore all other symbols that appear in s. Your function should return True if s has matched brackets and False if it does not.
Here are some examples to show how your function should work.
>>> matched("zb%78")
True
>>> matched("(7)(a")
False
>>> matched("a)*(?")
False
>>> matched("((jkl)78(A)&l(8(dd(FJI:),):)?)")
True
These are Nptel Programming DSA using Python Week 2 Assignment Answers
3. A list rotation consists of taking the first element and moving it to the end. For instance, if we rotate the list [1,2,3,4,5], we get [2,3,4,5,1]. If we rotate it again, we get [3,4,5,1,2].
Write a Python function rotatelist(l,k) that takes a list l and a positive integer k and returns the list l after k rotations. If k is not positive, your function should return l unchanged. Note that your function should not change l itself, and should return the rotated list.
Here are some examples to show how your function should work.
>>> rotatelist([1,2,3,4,5],1)
[2, 3, 4, 5, 1]
>>> rotatelist([1,2,3,4,5],3)
[4, 5, 1, 2, 3]
>>> rotatelist([1,2,3,4,5],12)
[3, 4, 5, 1, 2]
Solution:
###########
def factors(n):
factorlist = []
for i in range(1,n+1):
if n%i == 0:
factorlist.append(i)
return(factorlist)
def prime(n):
return(factors(n)==[1,n])
def primepartition(n):
for i in range(1,n//2+1):
if prime(i) and prime(n-i):
return(True)
return(False)
###########
def matched(s):
nesting = 0
for c in s:
if c == '(':
nesting = nesting + 1
elif c == ')':
nesting = nesting - 1
if nesting < 0:
return(False)
return(nesting == 0)
###########
def rotatelist(l,k):
retlist = l[:]
if k <= 0:
return(retlist)
for i in range(1,k+1):
retlist = retlist[1:] + [retlist[0]]
return(retlist)
###########
import ast
def tolist(inp):
inp = "["+inp+"]"
inp = ast.literal_eval(inp)
return (inp[0],inp[1])
def parse(inp):
inp = ast.literal_eval(inp)
return (inp)
fncall = input()
lparen = fncall.find("(")
rparen = fncall.rfind(")")
fname = fncall[:lparen]
farg = fncall[lparen+1:rparen]
if fname == "primepartition":
arg = parse(farg)
print(primepartition(arg))
elif fname == "matched":
arg = parse(farg)
print(matched(arg))
elif fname == "rotatelist":
(arg1,arg2) = parse(farg)
myarg1 = arg1[:]
rotatelist(arg1,arg2)
if myarg1 == arg1:
print(rotatelist(arg1,arg2))
else:
print("Illegal side effect")
else:
print("Function", fname, "unknown")
These are Nptel Programming DSA using Python Week 2 Assignment Answers
More Weeks of Programming Data Structure And Algorithms: Click Here
More Nptel courses: https://progiez.com/nptel
Programming DSA using Python Week 2 Assignment Answers(JULY-DEC 2022)
Q1. One of the following 10 statements generates an error. Which one? (Your answer should be a number between 1 and 10.)
x = [[3,5],"mimsy",2,"borogove",1] # Statement 1
y = x[0:50] # Statement 2
z = y # Statement 3
w = x # Statement 4
x[1] = x[1][:5] + 'ery' # Statement 5
y[1] = 4 # Statement 6
w[1][:3] = 'fea' # Statement 7
z[4] = 42 # Statement 8
x[0][0] = 5555 # Statement 9
a = (x[3][1] == 1) # Statement 10
Answer: 7
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Q2. Consider the following lines of Python code.
Which of the following holds at the end of this code?
b = [43,99,65,105,4]
a = b[2:]
d = b[1:]
c = b
d[1] = 95
b[2] = 47
c[3] = 73
Answer: c – a[0] == 65, b[3] == 73, c[3] == 73, d[1] == 95
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Q3. What is the value of endmsg after executing the following lines?
startmsg = "anaconda"
endmsg = ""
for i in range(1,1+len(startmsg)):
endmsg = endmsg + startmsg[-i]
Answer: ‘anaconda’
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Q4. What is the value of mylist after the following lines are executed?
def mystery(l):
l = l[2:]
return(l)
mylist = [7,11,13,17,19,21]
mystery(mylist)
Answer: [7,11,13,17,19,21]
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Assignment Answers
Q1. Write a function intreverse(n) that takes as input a positive integer n and returns the integer obtained by reversing the digits in n. Write a function matched(s) that takes as input a string s and checks if the brackets “(” and “)” in s are matched: that is, every “(” has a matching “)” after it and every “)” has a matching “(” before it. Your function should ignore all other symbols that appear in s. Your function should return True if s has matched brackets and False if it does not. Write a function sumprimes(l) that takes as input a list of integers l and retuns the sum of all the prime numbers in l.
Sol:-
def intreverse(n):
ans = 0
while n > 0:
(d,n) = (n%10,n//10)
ans = 10*ans + d
return(ans)
def matched(s):
nested = 0
for i in range(0,len(s)):
if s[i] == "(":
nested = nested+1
elif s[i] == ")":
nested = nested-1
if nested < 0:
return(False)
return(nested == 0)
def factors(n):
factorlist = []
for i in range(1,n+1):
if n%i == 0:
factorlist = factorlist + [i]
return(factorlist)
def isprime(n):
return(factors(n) == [1,n])
def sumprimes(l):
sum = 0
for i in range(0,len(l)):
if isprime(l[i]):
sum = sum+l[i]
return(sum)
These are Nptel Programming DSA using Python Week 2 Assignment Answers