Problem Solving Through Programming In C Week 8
Session: JULY-DEC 2023
Course Name: Problem Solving Through Programming In C
Course Link: Click Here
These are Problem Solving Through Programming In C Assignment 8 Answers
Q1. A function prototype is used for
a) Declaring the function logic
b) Calling the function from the main body
c) Telling the compiler, the kind of arguments used in the function
d) Telling the user for proper use of syntax while calling the function
Answer: c) Telling the compiler, the kind of arguments used in the function
Q2. What is the default return type if it is not specified in function definition?
a) void
b) integer
c) double
d) float
Answer: b) integer
Q3. What will be the output?
a) 70
b) Garbage value
c) Compilation error
d) None
Answer: c) Compilation error
These are Problem Solving Through Programming In C Assignment 8 Answers
Q4. How many times will ‘Hello world’ be printed?
a) Infinite times
b) 32767
c) 65535
d) Till stack overflows
Answer: d) Till stack overflows
Q5. How many times ‘Hi’ will be printed in the program given below
a) Only once
b) Zero times
c) Infinite times
d) Compilation error
Answer: b) Zero times
Q6. How many times the function factorial will be executed?
Answer: 6
These are Problem Solving Through Programming In C Assignment 8 Answers
Q7. What will be the output?
a) 8,4, 0, 2, 14
b) 8, 4, 0, 2, 0
c) 2, 0, 4, 8, 14
d) 2, 0, 4, 8, 0
Answer: d) 2, 0, 4, 8, 0
Q8. What is the output of the following C program?
a) 55
b) 45
c) 66
d) 10
Answer: a) 55
Q9. Consider the function
a) Maximum of a, b
b) Positive difference between a and b
c) Sum of a and b
d) Minimum of a and b
Answer: d) Minimum of a and b
These are Problem Solving Through Programming In C Assignment 8 Answers
Q10. What is the output of the C code given below
Answer: 27.08
Assignment
Question 1
Write a C Program to find HCF of 4 given numbers using recursive function
Solution:
int HCF(int x0, int y)
{
while (x0 != y)
{
if (x0 > y)
{
return HCF(x0 - y, y);
}
else
{
return HCF(x0, y - x0);
}
}
return(x0);
}
Question 2
Write a C Program to find power of a given number using recursion. The number and the power to be calculated is taken from test case
Solution:
long power(int abc, int pow)
{
if (pow)
{
return (abc * power(abc, pow - 1));
}
return 1;
}
Question 3
Write a C Program to print Binary Equivalent of an Integer using Recursion
Solution:
int binary_conversion(int xy)
{
if (xy == 0)
{
return 0;
}
else
{
return (xy % 2) + 10 * binary_conversion(xy / 2);
}
}
Question 4
Write a C Program to reverse a given word using function. e.g. INDIA should be printed as AIDNI
Solution:
int sz;
sz = strlen(str1);
reverse(str1, 0, sz - 1);
printf("The string after reversing is: %s", str1);
return 0;
}
void reverse(char str1[], int index, int size)
{
char temp;
temp = str1[index];
str1[index] = str1[size - index];
str1[size - index] = temp;
if (index == size / 2)
{
return;
}
reverse(str1, index + 1, size);
}
These are Problem Solving Through Programming In C Assignment 8 Answers
More Weeks of Problem Solving Through Programming In C: Click here
More Nptel Courses: Click here
Session: JAN-APR 2023
Course Name: Problem Solving Through Programming In C
Course Link: Click Here
These are Problem Solving Through Programming In C Assignment 8 Answers
Q1. What is the purpose of the return statement in a function in C?
a) To terminate the function and return control to the calling function
b) To assign a value to a variable
c) To print a message to the console
d) To declare a variable
Answer: a) To terminate the function and return control to the calling function
Q2. What is a function prototype in C?
a) A function definition that includes the function name, parameters, and body
b) A statement that declares the name, return type, and parameters of a function
c) A block of code that is executed when a function is called
d) A reserved keyword that specifies the type of a variable
Answer: b) A statement that declares the name, return type, and parameters of a function
These are Problem Solving Through Programming In C Assignment 8 Answers
Q3. What is the difference between a function declaration and a function definition in C?
a) A declaration specifies the function name, return type, and parameters, while a definition includes the function body.
b) A declaration includes the function body, while a definition specifies the function name, return type, and parameters.
c) There is no difference between a declaration and a definition in C.
d) None of the above.
Answer: a) A declaration specifies the function name, return type, and parameters, while a definition includes the function body.
Q4. A function prototype is used for
a) Declaring the function logic
b) Calling the function from the main body
c) Telling the compiler, the kind of arguments used in the function
d) Telling the user for proper use of syntax while calling the function
Answer: c) Telling the compiler, the kind of arguments used in the function
These are Problem Solving Through Programming In C Assignment 8 Answers
Q5. What is the scope of a variable declared inside a function in C?
a) The variable can be accessed by any function in the program
b) The variable can only be accessed within the function where it is declared
c) The variable can be accessed by any function in the same file
d) The variable can be accessed by any function in the same module
Answer: b) The variable can only be accessed within the function where it is declared
Q6. What is the output of the following C program?
a) Compiler error as foo() is not declared in main
b) 1 2
c) 2 1
d) Compile-time error due to declaration of functions inside main
Answer: b) 1 2
These are Problem Solving Through Programming In C Assignment 8 Answers
Q7. What is the output of the following code snippet?
a) x = 1, y = 2
b) x = 2, y = 1
c) Compilation error
d) Runtime error
Answer: a) x = 1, y = 2
Q8. What is the error in the following program
a) Error: Return statement cannot be used with conditional operators
b) Error: Prototype declaration
c) Error: Two return statements cannot be used in any function
d) No error
Answer: a) Error: Return statement cannot be used with conditional operators
These are Problem Solving Through Programming In C Assignment 8 Answers
Q9.
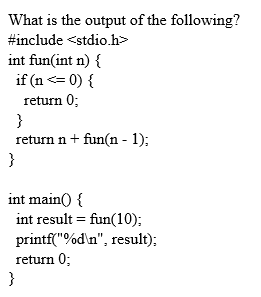
Answer: 55
Q10. Consider the function
For the input x = 95, the function will return
a) 89
b) 90
c) 91
d) 92
Answer: c) 91
These are Problem Solving Through Programming In C Assignment 8 Answers
Problem Solving Through Programming In C Programming Assignment
Question 1
Write a C Program to find HCF of 4 given numbers using recursive function
Solution:
int HCF(int x, int y)
{
while (x != y)
{
if (x > y)
{
return HCF(x - y, y);
}
else
{
return HCF(x, y - x);
}
}
return x;
}
Question 2
Write a C Program to find power of a given number using recursion. The number and the power to be calculated is taken from test case
Solution:
long power(int pow, int num) {
if (num != 0)
return (pow * power(pow, num - 1));
else
return 1;
}
These are Problem Solving Through Programming In C Assignment 8 Answers
Question 3
Write a C Program to print Binary Equivalent of an Integer using Recursion
Solution:
int binary_conversion(int num)
{
if (num == 0)
{
return 0;
}
else
{
return (num % 2) + 10 * binary_conversion(num / 2);
}
}
Question 4
Write a C program to print a triangle of prime numbers upto given number of lines of the triangle.
e.g If number of lines is 3 the triangle will be
2
3 5
7 11 13
Solution:
int i, j, k=2;
for(i=1; i<=lines; i++)
{
for(j=1; j<=i; j++) {
while(!prime(k))
k++;
printf("%d\t", k);
k++;
}
printf("\n");
}
return 0;
}
int prime(int num) {
int i;
for(i=2; i<=num/2; i++) {
if(num%i == 0) {
return 0;
}
}
return 1;
}
These are Problem Solving Through Programming In C Assignment 8 Answers
More Weeks of Problem Solving Through Programming In C: Click Here
More Nptel courses: https://progiez.com/nptel
Session: JULY-DEC 2022
Course Name: Problem Solving Through Programming In C NPTEL
Link of course: Click Here
These are Problem Solving Through Programming In C Assignment 8 Answers
Q1. What will be the output?
a) 70
b) Garbage value
c) Compilation error
d) None
Answer: c) Compilation error
Q2. Which of the following statements are correct in C?
a) A function with the same name cannot have different signatures
b) A function with the same name cannot have different return types
c) A function with the same name cannot have a different number of parameters
d) All of the mentioned
Answer: d) All of the mentioned
Q3. What will the function return?
a) x * y where x and y are integers
b) x * y where x and y are non-negative integers
c) x + y where x and y are integers
d) x + y where x and y are non-negative integers
Answer: b) x * y where x and y are non-negative integers
These are Problem Solving Through Programming In C Assignment 8 Answers
Q4. What is the output of the following C program?
a) Compiler error as foo() is not declared in main
b) 1 2
c) 2 1
d) Compile time error due to declaration of functions inside main
Answer: b) 1 2
Q5. What will be the output?
a) Choice 1
b) CChoice1
c) DefaultChoice1
d) CChoice1Choice2
Answer: b) CChoice1
Q6. What will be the output of the C code?
a) Compilation error
b) 0, 1, 2 …….., 127
c) 0, 1, 2, …….., 127, -128, -127,…infinite loop
d) 1, 2, 3…….,12
Answer: c) 0, 1, 2, …….., 127, -128, -127,…infinite loop
These are Problem Solving Through Programming In C Assignment 8 Answers
Q7. What is the output of the following C program?
a) 55
b) 45
c) 66
d) 10
Answer: a) 55
Q8. Consider the function
find(int x, int y)
{
return((x<y) ? 0 : (x-y));
}
Let a and b be two non-negative integers. The call find(a, find(a, b)) can be used to find the
a) Maximum of a, b
b) Positive difference between a and b
c) Sum of a and b
d) Minimum of a and b
Answer: d) Minimum of a and b
These are Problem Solving Through Programming In C Assignment 8 Answers
Q9. The function above has a flaw that may result in a serious error during some invocations.
Which one of the following describes the deficiency illustrated above?
a) For some values of n, the environment will almost certainly exhaust its stack space before the calculation completes.
b) An error in the algorithm causes unbounded recursion for all values of n.
c) A break statement should be inserted after each case. Fall-through is not desirable here.
d) The fibonacci() function includes calls to itself. This is not directly supported by Standard C due to its unreliability.
Answer: a) For some values of n, the environment will almost certainly exhaust its stack space before the calculation completes.
Q10. What is the output of the C code given below.
Answer: 27.08
These are Problem Solving Through Programming In C Assignment 8 Answers
Programming Assigment
Question: 1 Write a C Program to find HCF of 4 given numbers using recursive function
Solution:
//Code
These are Problem Solving Through Programming In C Assignment 8 Answers
Question: 2 Write a C Program to print Binary Equivalent of an Integer using Recursion
Solution:
//Code
These are Problem Solving Through Programming In C Assignment 8 Answers
Question: 3 Write a program to calculate the sum of a given series.
Solution:
//Code
These are Problem Solving Through Programming In C Assignment 8 Answers
Question : 4 Write a program to express a given integer as a Sum of Two Prime Numbers
For example if the number is 10
The result will be
10 = 3 + 7
10 = 5 + 5
Solution:
//Code
These are Problem Solving Through Programming In C Assignment 8 Answers
More NPTEL Solution: https://progiez.com/nptel

The content uploaded on this website is for reference purposes only. Please do it yourself first. |