Problem Solving Through Programming In C Nptel Week 5 Assignment Answers
Are you looking for the Problem Solving Through Programming In C Week 5 Answers? You’ve come to the right place! This resource offers comprehensive solutions to the Week 5 assignment questions, focusing on fundamental concepts in C programming.
Table of Contents
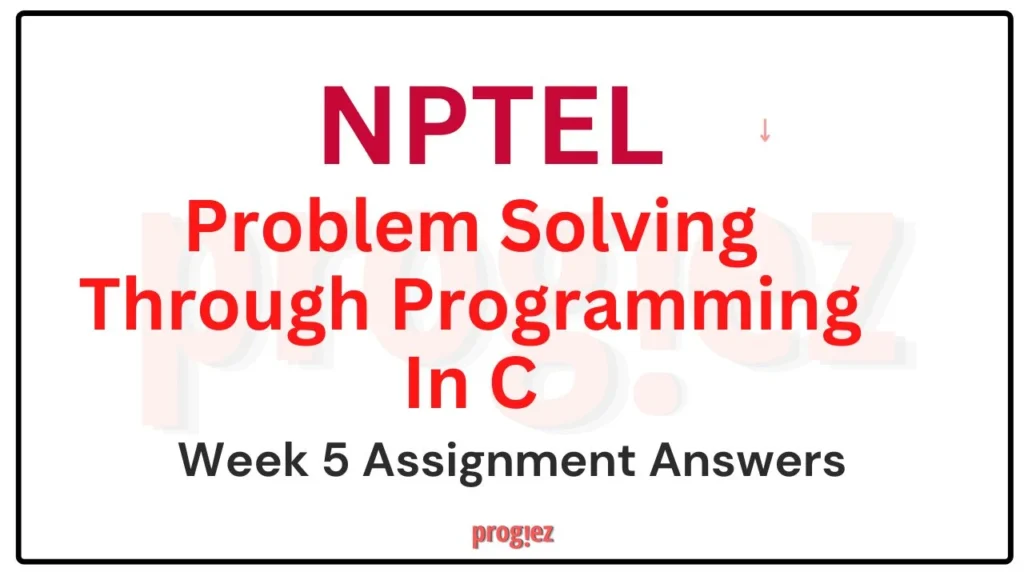
Problem Solving Through Programming In C Week 5 Answers (Jan-Apr 2025)
Course Link: Click Here
Q1. In C language, three-way transfer of control is possible using:
a) Ternary operator
b) Unary operator
c) Logical operator
d) None of the above are true
Q2. The loop in which the statements within the loop are executed at least once is called:
a) for
b) do-while
c) while
d) goto
Q3. What will be the output?
#include <stdio.h>
int main()
{
float k = 0;
for (k = 0.5; k < 3; k + 4)
printf("I love C\n");
return 0;
}
Q4. What is the output of the following code?
#include <stdio.h>
int main()
{
int i = 1;
do
{
printf("while vs do-while\n");
} while (i = 1);
printf("Out of loop");
return 0;
}
Q5. Find the output of the following C program:
#include <stdio.h>
int main()
{
int i = 0;
if (i = 0)
{
i = i + 1;
break;
}
printf("%d", i);
return 0;
}
Q6. What is the output of the following C program?
#include <stdio.h>
int main()
{
int a = 0, i, b;
for (i = 0; i <= 2; i += 0.5)
{
a++;
continue;
}
printf("%d", a);
return 0;
}
Q7. What will be the output?
#include <stdio.h>
int main()
{
int i = 0;
for (;;)
{
if (i == 10)
break;
printf("%d ", ++i);
}
return 0;
}
Q8. What is the output of the following C code?
#include <stdio.h>
int main()
{
int a = 1;
if (a)
printf("True\n");
if (!a)
printf("False\n");
return 0;
}
Q9. What is the output of the below C program?
#include <stdio.h>
int main()
{
short int k = 1, j = 1;
while (k <= 4 || j <= 3)
{
k = k + 2;
j++;
}
printf("%d, %d", k, j);
return 0;
}
Problem Solving Through Programming In C Week 5 Answers (July-Dec 2024)
Q1.Which looping construct is best when the number of iterations is known beforehand?
a) ‘while’ loop
b) ‘do-while’ loop
c) ‘for’ loop
d) all of the above are true
Answer: c) ‘for’ loop
Q2.In a ‘do-while’ loop, when is the condition tested?
a) Before the first iteration
b) After the first iteration
c) At the middle of each iteration
d) At the end of each iteration
Answer: d) At the end of each iteration
For answers or latest updates join our telegram channel: Click here to join
Q3.Which ‘for’ loop has range of similar indexes of ‘i’ used in for (i = 0; i < n; i++);?
a) for (i = n; i>0; i–);
b) for (i = n; i >= 0; i–);
c) for (i = n-1; i>0; i–);
d) for (i = n-1; i>-1; i–);
Answer: d) for (i = n-1; i>-1; i–);
Q4What is the output of the following code?
include
int main() {
mt i;
for(int i = 0: <10: i++):
printf{“%d”. i):
retum 0;
}
a) 10
b) 0123456789
c) Infinite loop, no output
d) Syntax error
Answer : a) 10
For answers or latest updates join our telegram channel: Click here to join
Q5.Find the output of the following C program
include
int main()
{
inti=0;
if(i==0)
L
i=itl;
break:
}
printf(“%d”, 1);
return 0:
}
a) 0
b) 1
c) No output
d) Compiler error
Answer: d) Compiler error
Q6.What is the output of the following C program?
include
int main)
{
nta=0,1b;
for (i=0:i<=2: i+=05)
{
att;
continue;
}
printf{“%d”, a);
return 0;
}
a) 5
b) 4
c) 1
d) No output
Answer: d) No output
For answers or latest updates join our telegram channel: Click here to join
These are Problem Solving Through Programming In C Week 5 Answers
Q7.‘What will be the output?
include
int main()
{
int i=0;
for(::)
{
if(i==10)
break;
printf{(“%d “, ++):
}
return 0;
}
a) Syntax error
b) 0 1 2 3 4 5 6 7 8 9 10
c) 1 2 3 4 5 6 7 8 9 10
d) 0 1 2 3 4 5 6 7 8 9
Answer: c) 1 2 3 4 5 6 7 8 9 10
Q8.If the following loop is implemented
include
int main() {
int num = 0;
do {
- – num;
printf(“%d”, num):
num ++;
}
‘while(num >= 0);
return 0;
}
a) A run time error will be reported
b) The program will not enter into the loop
c) The loop will run infinitely
d) There will be a compilation error reported
Answer: c) The loop will run infinitely
For answers or latest updates join our telegram channel: Click here to join
Q9. ‘What is the output of the below C program?
include
int main()
{
short int k=1, j=1;
while (k <= 4]|j <= 3)
{
k=k+2;
J+=1
}
printf(“%d, %d”, k, j);
return 0;
}
a) 5, 4
b) 7, 4
c) 5, 6
d) 6, 4
Answer: b) 7, 4
Q10.How many times is the loop executed in the following code?
for(inti=0;1<=10;i+=2) {
printf(“%d “, 1);
}
a) 5
b) 6
c) 10
d) 11
Answer: b) 6
For answers or latest updates join our telegram channel: Click here to join
These are Problem Solving Through Programming In C Week 5 Answers
All weeks of Problem Solving Through Programming in C : Click Here
More Nptel Courses: https://progiez.com/nptel-assignment-answers
Problem Solving Through Programming In C Week 5 Answers (JULY-DEC 2023)
Course Name: Problem Solving Through Programming In C
Course Link: Click Here
These are Problem Solving Through Programming In C Assignment 5 Answers
Q1. The statement that transfers control to the beginning of the loop is called
a) break
b) continue
c) goto
d) None of the above
Answer: b) continue
Q2. In C three way transfer of control is possible using
a) Unary operator
b) Logical operator
c) Ternary operator
d) None
Answer: c) Ternary operator
Q3. What will be the output of the following code?
a) ‘while vs do-while’ once
b) ‘Out of loop’ infinite times
c) Both ‘while vs do-while’ and ‘Out of loop’ once
d) ‘while vs do-while’ infinite times
Answer: d) ‘while vs do-while’ infinite times
These are Problem Solving Through Programming In C Assignment 5 Answers
Q4. What is the output of the following C program?
a) 5
b) 10
c) No output
d) Compilation error
Answer: c) No output
Q5. What is the output of the following C code?
a) True
b) False
c) Both ‘True’ and ‘False’
d) Compilation error
Answer: c) Both ‘True’ and ‘False’
Q6. What will be the output?
a) After loop x=1
b) 1
After loop x=2
c) 1 2 3 4 5 6 7 8 9 10
d) No output
Answer: d) No output
These are Problem Solving Through Programming In C Assignment 5 Answers
Q7. What will be the output?
a) Error
b) I love C – will be printed 3 times
c) I love C – will be printed 6 times
d) I love C – will be printed 5 times
Answer: b) I love C – will be printed 3 times
Q8. What will be the output?
a) 1
b) 2
c) 3
d) Error
Answer: b) 2
Q9. The following program is used to find the reverse of a number using C language. Find the missing condition inside while statement (indicated as ‘xxxx’).
a) n!=0
b) n==0
c) n%10==0
d) n/10==0
Answer: a) n!=0
These are Problem Solving Through Programming In C Assignment 5 Answers
Q10. Compute the printed value of i & j of the C program given below
a) 8,10
b) 8,9
c) 6, 9
d) 7, 10
Answer: c) 6, 9
Assignment
Question 1
Write a C program to check whether a given number (N) is a perfect number or not.
[Perfect Number – A perfect number is a positive integer number which is equals to the sum of its proper positive divisors. For example 6 is a perfect number because its proper divisors are 1, 2, 3 and it’s sum is equals to 6.]
Solution:
int sum=0;
for(int i = 1; i <= N / 2; i++)
{
if(N % i == 0)
{
sum += i;
}
}
if(sum == N){
printf("%d is a perfect number.", N);
}
else{
printf("%d is not a perfect number.", N);
}
return 0;
}
Question 2
Write a C program to count total number of digits of an Integer number (N).
Solution:
int temp, count;
count=0;
temp=N;
while(temp>0)
{
count++;
temp/=10;
}
printf("The number %d contains %d digits.",N,count);
}
Question 3
Write a C program to check whether the given number(N) can be expressed as Power of Two (2) or not.
For example 8 can be expressed as 2^3.
Solution:
int temp, flag;
temp=N;
flag=0;
while(temp!=1)
{
if(temp%2!=0){
flag=1;
break;
}
temp=temp/2;
}
if(flag==0)
printf("%d is a number that can be expressed as power of 2.",N);
else
printf("%d cannot be expressed as power of 2.",N);
}
Question 4
Write a C program to find sum of following series where the value of N is taken as input
1+ 1/2 + 1/3 + 1/4 + 1/5 + .. 1/N
Solution:
int i;
for(i=1;i<=N;i++)
{
sum = sum + ((float)1/(float)i);
}
printf("Sum of the series is: %.2f",sum);
}
These are Problem Solving Through Programming In C Assignment 5 Answers
More Weeks of Problem Solving Through Programming In C: Click here
More Nptel Courses: Click here
Problem Solving Through Programming In C Week 5 Answers (JAN-APR 2023)
Course Name: Problem Solving Through Programming In C
Course Link: Click Here
These are Problem Solving Through Programming In C Assignment 5 Answers
Q1. “continue” statement in C is used
a) to continue to the next line of code
b) to debug
c) to stop the current iteration and begin the next iteration from the beginning
d) None of the above are true
Answer: c) to stop the current iteration and begin the next iteration from the beginning
Q2. The operators << and >> are
a) Relational operator
b) Logical operator
c) Assignment operator
d) Bitwise shift operator
Answer: d) Bitwise shift operator
These are Problem Solving Through Programming In C Assignment 5 Answers
Q3. Compute the printed value of i of the C program given below
a) 4,5
b) 4,4
c) 5,5
d) 0,0
Answer: c) 5,5
Q4. How many times ‘Hello’ will be printed while executing the below C code?
a) 4 times
b) 20 times
c) 5 times
d) no print
Answer: c) 5 times
These are Problem Solving Through Programming In C Assignment 5 Answers
Q5. What is the output of the following C code?
a) True
b) False
c) Both ‘True’ and ‘False’
d) Compilation error
Answer: c) Both ‘True’ and ‘False’
Q6. Find the output of the following C program
a) 0
b) 1
c) No output
d) Compilation error
Answer: d) Compilation error
These are Problem Solving Through Programming In C Assignment 5 Answers
Q7. How many times the ‘Hello” will be printed in the below C code?
a) 1 time
b) 10 times
c) 11 times
d) Compilation error
Answer: a) 1 time
Q8. How many times Hello will be printed in the below C code?
a) 10 times
b) 5 times
c) 6 times
d) Infinite times
Answer: c) 6 times
These are Problem Solving Through Programming In C Assignment 5 Answers
Q9. ‘What will be the output?
a) 1
b) 2
c) 3
d) Error
Answer: b) 2
Q10. What is the output of the following C program?
a) 5
b) 10
c) No output
d) Compilation error
Answer: c) No output
These are Problem Solving Through Programming In C Assignment 5 Answers
Programming Assignment
Question 1
Write a C program to count total number of digits of an Integer number (N).
Solution:
int temp, count;
count=0;
temp=N;
while(temp>0)
{
count++;
temp/=10;
}
printf("The number %d contains %d digits.",N,count);
}
Question 2
Write a C program to check whether the given number(N) can be expressed as Power of Two (2) or not. For example 8 can be expressed as 2^3.
Solution:
int temp, flag;
temp=N;
flag=0;
while(temp!=1)
{
if(temp%2!=0){
flag=1;
break;
}
temp=temp/2;
}
if(flag==0)
printf("%d is a number that can be expressed as power of 2.",N);
else
printf("%d cannot be expressed as power of 2.",N);
}
These are Problem Solving Through Programming In C Assignment 5 Answers
Question 3
Write a C program to find sum of following series where the value of N (odd integer number) is taken as input
123252+….+n2?
Solution:
for (i = 1; i <= n; i += 2) {
sum += i * i;
}
printf("Sum=%d\n", sum);
return 0;
}
Question 4
Write a C program to print the following Pyramid pattern upto Nth row. Where N (number of rows to be printed) is taken as input.
For example when the value of N is 5 the pyramid will be printed as follows
*****
****
***
**
*
Solution:
int i,j;
for(i=N; i>0; i--)
{
for(j=0;j<i;j++)
{
printf("*");
}
printf("\n");
}
}
These are Problem Solving Through Programming In C Assignment 5 Answers
More Weeks of Problem Solving Through Programming In C: Click Here
More Nptel courses: https://progiez.com/nptel
Problem Solving Through Programming In C Week 5 Answers (July-Dec 2022)
Course Name: Problem Solving Through Programming In C NPTEL
Link of course: Click Here
These are Problem Solving Through Programming In C Assignment 5 Answers
Q1) What will be the output?
a) 10 9 8 7 6 5 4 3 2 1
b) 1 2 3 4 5 6 7 8 9 10
c) No output
d) None of the above statements are correct
Answer: c) No output
Q2) Which of the following is not an infinite loop?
a) for(; ; )
b) for(x=0; x<=10)
c) while(1)
d) while(0)
Answer: d) while(0)
Q3) Consider the following and identify the false statement(s)?
i) ‘do-while’ loop must be terminated by a semi colon.
ii) ‘do-while’ loop is always an infinite loop.
iii) Even if the condition is false, the ‘do-while’ loop executes once.
iv) ‘do-while’ loop is an entry-controlled loop.
Answer: (ii), (iv)
Q4) Compute the printed value of ‘m’ and ‘n’ of the C program given below?
a) 5,7
b) 5,5
c) 7,7
d) 0,0
Answer: c) 7,7
These are Problem Solving Through Programming In C Assignment 5 Answers
Q5) What should be in the place of so that except i=8, rest of the values of i (as defined in the ‘for’ loop : i=0 to i=19) will be printed?
a) break
b) continue
c) switch
d) exit
Answer: b) continue
Q6) What will be the output?
a) NPTEL
b) IIT/IISc
c) NPTELSWAWAMIIT/IISC
d)Compilation error
Answer: c) NPTELSWAWAMIIT/IISC
Q7) What will be the output?
a) 1,3
b) 1,3 3,1
c) 1,3 2,2 3,1
d) 0,0
Answer: c) 1,3 2,2 3,1
These are Problem Solving Through Programming In C Assignment 5 Answers
Q8) What will be the output of the program?
a) 4 will print 1 times
b) 4 will print 3 times
c) 4 will print 4 times
d) No output
Answer: b) 4 will print 3 times
Q9) For the C program given below, if the input given by the user is 7. What will be shown on the output window?
a) The number is odd
b) The number is prime
c) The number is odd
d) The number is prime Syntax Error
Answer: b) The number is prime
Q10) What will be the output?
a) 0123456789 11 12…..infinite times
b) 123456789 11 12….infinite times
c) Won’t print anything
d) Error
Answer: c) Won’t print anything
These are Problem Solving Through Programming In C Assignment 5 Answers
Programming Assignment
Question 1
Write a C program to count total number of digits of an Integer number (N).
Solution:
#include <stdio.h>
int main()
{
int N;
scanf("%d",&N);
int temp, count;
count=0;
temp=N;
while(temp>0)
{
count++;
temp/=10;
}
printf("The number %d contains %d digits.",N,count);
}
These are Problem Solving Through Programming In C Assignment 5 Answers
Question 2
Write a C program to find sum of following series where the value of N is taken as input
1+ 1/2 + 1/3 + 1/4 + 1/5 + .. 1/N
Solution:
#include < stdio.h >
int main()
{
int N;
float sum = 0.0;
scanf("%d",&N);
int i;
for(i=1;i < = N;i++)
{
sum = sum + ((float)1/(float)i);
}
printf("Sum of the series is: %.2f\n",sum);
}
These are Problem Solving Through Programming In C Assignment 5 Answers
Question 3
Write a C program to check whether the given number(N) can be expressed as Power of Two (2) or not.
For example 8 can be expressed as 2^3
Solution:
#include < stdio.h>
int main()
{
int N;
scanf("%d",&N);
int temp, flag;
temp=N;
flag=0;
while(temp!=1)
{
if(temp%2!=0)
{
flag=1;
break;
}
temp=temp/2;
}
if(flag==0)
printf("%d is a number that can be expressed as power of 2.",N);
else
printf("%d cannot be expressed as power of 2.",N);
}
These are Problem Solving Through Programming In C Assignment 5 Answers
Question 4
Write a C program to print the following Pyramid pattern upto Nth row. Where N (number of rows to be printed) is taken as input.
For example when the value of N is 5 the pyramid will be printed as follows:
*****
****
***
**
*
Solution:
#include<stdio.h>
int main()
{
int N;
scanf("%d", &N);
int i, j;
for(i=N; i>0; i--)
{
for(j=0;j < i;j++)
{
printf("*");
}
printf("\n");
}
}