972. Equal Rational Numbers LeetCode Solution
In this guide, you will get 972. Equal Rational Numbers LeetCode Solution with the best time and space complexity. The solution to Equal Rational Numbers problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Equal Rational Numbers solution in C++
- Equal Rational Numbers solution in Java
- Equal Rational Numbers solution in Python
- Additional Resources
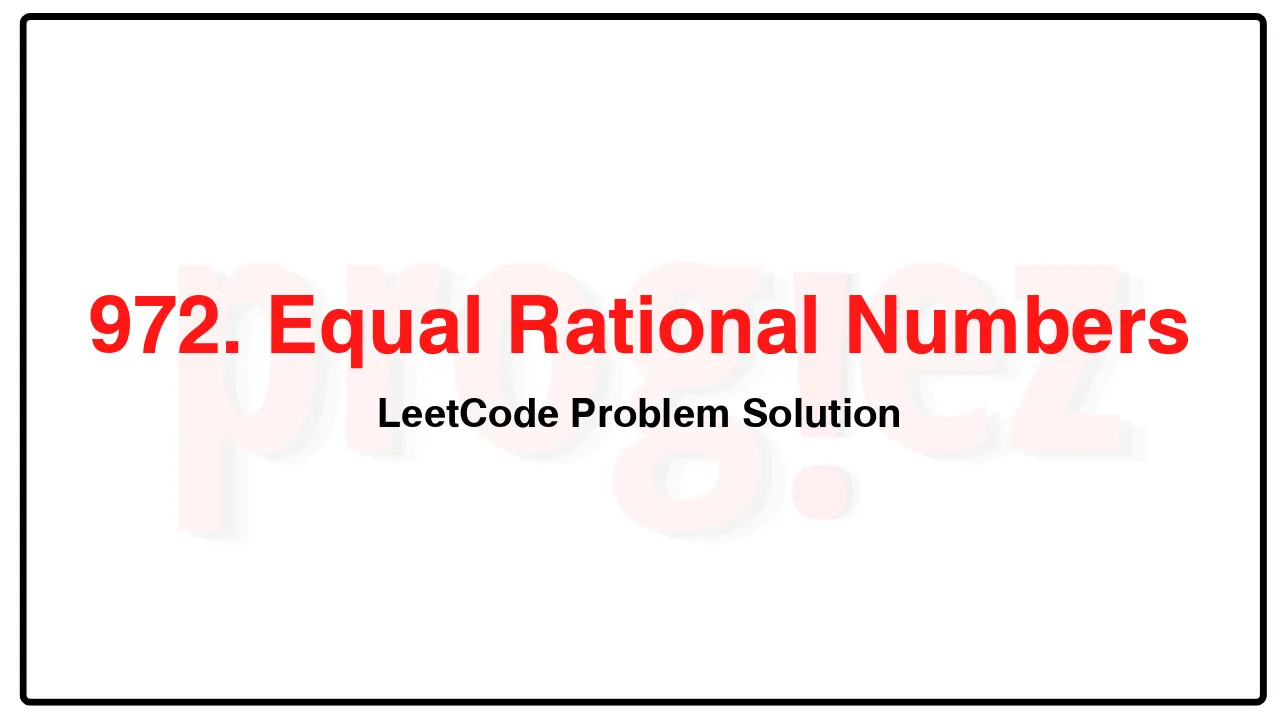
Problem Statement of Equal Rational Numbers
Given two strings s and t, each of which represents a non-negative rational number, return true if and only if they represent the same number. The strings may use parentheses to denote the repeating part of the rational number.
A rational number can be represented using up to three parts: , , and a . The number will be represented in one of the following three ways:
For example, 12, 0, and 123.
For example, 0.5, 1., 2.12, and 123.0001.
For example, 0.1(6), 1.(9), 123.00(1212).
The repeating portion of a decimal expansion is conventionally denoted within a pair of round brackets. For example:
1/6 = 0.16666666… = 0.1(6) = 0.1666(6) = 0.166(66).
Example 1:
Input: s = “0.(52)”, t = “0.5(25)”
Output: true
Explanation: Because “0.(52)” represents 0.52525252…, and “0.5(25)” represents 0.52525252525….. , the strings represent the same number.
Example 2:
Input: s = “0.1666(6)”, t = “0.166(66)”
Output: true
Example 3:
Input: s = “0.9(9)”, t = “1.”
Output: true
Explanation: “0.9(9)” represents 0.999999999… repeated forever, which equals 1. [See this link for an explanation.]
“1.” represents the number 1, which is formed correctly: (IntegerPart) = “1” and (NonRepeatingPart) = “”.
Constraints:
Each part consists only of digits.
The does not have leading zeros (except for the zero itself).
1 <= .length <= 4
0 <= .length <= 4
1 <= .length <= 4
Complexity Analysis
- Time Complexity: O(|\texttt{s}| + |\texttt{t}|)
- Space Complexity: O(1)
972. Equal Rational Numbers LeetCode Solution in C++
class Solution {
public:
bool isRationalEqual(string s, string t) {
return abs(valueOf(s) - valueOf(t)) < 1e-9;
}
private:
const vector<double> ratios{1.0, 1.0 / 9, 1.0 / 99, 1.0 / 999, 1.0 / 9999};
double valueOf(const string& s) {
if (s.find('(') == string::npos)
return stod(s);
// Get the indices..
const int leftParenIndex = s.find_first_of('(');
const int rightParenIndex = s.find_first_of(')');
const int dotIndex = s.find_first_of('.');
// integerAndNonRepeating := <IntegerPart><.><NonRepeatingPart>
const double integerAndNonRepeating = stod(s.substr(0, leftParenIndex));
const int nonRepeatingLength = leftParenIndex - dotIndex - 1;
// repeating := <RepeatingPart>
const int repeating = stoi(s.substr(leftParenIndex + 1, rightParenIndex));
const int repeatingLength = rightParenIndex - leftParenIndex - 1;
return integerAndNonRepeating +
repeating * pow(0.1, nonRepeatingLength) * ratios[repeatingLength];
}
};
/* code provided by PROGIEZ */
972. Equal Rational Numbers LeetCode Solution in Java
class Solution {
public boolean isRationalEqual(String s, String t) {
return Math.abs(valueOf(s) - valueOf(t)) < 1e-9;
}
private static double[] ratios = new double[] {1.0, 1.0 / 9, 1.0 / 99, 1.0 / 999, 1.0 / 9999};
private double valueOf(final String s) {
if (!s.contains("("))
return Double.valueOf(s);
// Get the indices..
final int leftParenIndex = s.indexOf('(');
final int rightParenIndex = s.indexOf(')');
final int dotIndex = s.indexOf('.');
// integerAndNonRepeating := <IntegerPart><.><NonRepeatingPart>
final double nonRepeating = Double.valueOf(s.substring(0, leftParenIndex));
final int nonRepeatingLength = leftParenIndex - dotIndex - 1;
// repeating := <RepeatingPart>
final int repeating = Integer.parseInt(s.substring(leftParenIndex + 1, rightParenIndex));
final int repeatingLength = rightParenIndex - leftParenIndex - 1;
return nonRepeating + repeating * Math.pow(0.1, nonRepeatingLength) * ratios[repeatingLength];
}
}
// code provided by PROGIEZ
972. Equal Rational Numbers LeetCode Solution in Python
class Solution:
def isRationalEqual(self, s: str, t: str) -> bool:
ratios = [1, 1 / 9, 1 / 99, 1 / 999, 1 / 9999]
def valueOf(s: str) -> float:
if s.find('(') == -1:
return float(s)
# Get the indices.
leftParenIndex = s.find('(')
rightParenIndex = s.find(')')
dotIndex = s.find('.')
# integerAndNonRepeating := <IntegerPart><.><NonRepeatingPart>
integerAndNonRepeating = float(s[:leftParenIndex])
nonRepeatingLength = leftParenIndex - dotIndex - 1
# repeating := <RepeatingPart>
repeating = int(s[leftParenIndex + 1:rightParenIndex])
repeatingLength = rightParenIndex - leftParenIndex - 1
return integerAndNonRepeating + repeating * 0.1**nonRepeatingLength * ratios[repeatingLength]
return abs(valueOf(s) - valueOf(t)) < 1e-9
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.