788. Rotated Digits LeetCode Solution
In this guide, you will get 788. Rotated Digits LeetCode Solution with the best time and space complexity. The solution to Rotated Digits problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Rotated Digits solution in C++
- Rotated Digits solution in Java
- Rotated Digits solution in Python
- Additional Resources
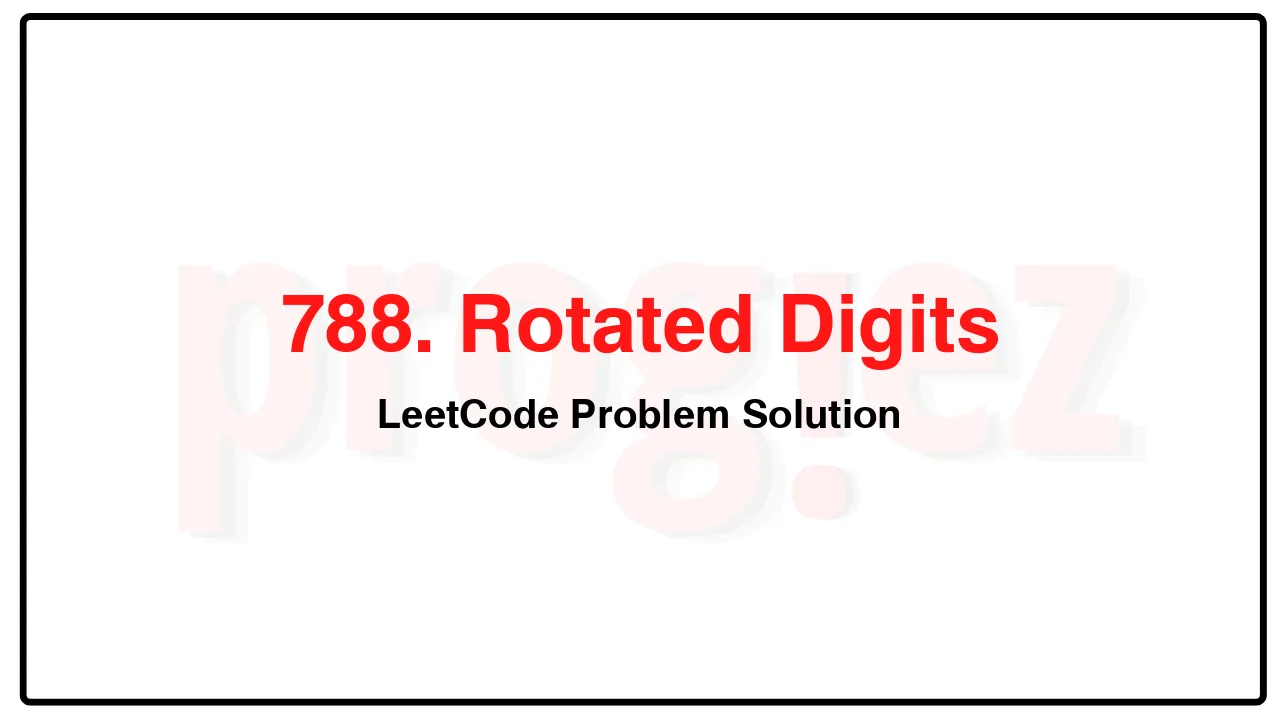
Problem Statement of Rotated Digits
An integer x is a good if after rotating each digit individually by 180 degrees, we get a valid number that is different from x. Each digit must be rotated – we cannot choose to leave it alone.
A number is valid if each digit remains a digit after rotation. For example:
0, 1, and 8 rotate to themselves,
2 and 5 rotate to each other (in this case they are rotated in a different direction, in other words, 2 or 5 gets mirrored),
6 and 9 rotate to each other, and
the rest of the numbers do not rotate to any other number and become invalid.
Given an integer n, return the number of good integers in the range [1, n].
Example 1:
Input: n = 10
Output: 4
Explanation: There are four good numbers in the range [1, 10] : 2, 5, 6, 9.
Note that 1 and 10 are not good numbers, since they remain unchanged after rotating.
Example 2:
Input: n = 1
Output: 0
Example 3:
Input: n = 2
Output: 1
Constraints:
1 <= n <= 104
Complexity Analysis
- Time Complexity: O(n\log n)
- Space Complexity: O(1)
788. Rotated Digits LeetCode Solution in C++
class Solution {
public:
int rotatedDigits(int n) {
int ans = 0;
for (int i = 1; i <= n; ++i)
if (isGoodNumber(i))
++ans;
return ans;
}
private:
bool isGoodNumber(int i) {
bool isRotated = false;
for (const char c : to_string(i)) {
if (c == '0' || c == '1' || c == '8')
continue;
if (c == '2' || c == '5' || c == '6' || c == '9')
isRotated = true;
else
return false;
}
return isRotated;
}
};
/* code provided by PROGIEZ */
788. Rotated Digits LeetCode Solution in Java
class Solution {
public int rotatedDigits(int n) {
int ans = 0;
for (int i = 1; i <= n; ++i)
if (isGoodNumber(i))
++ans;
return ans;
}
private boolean isGoodNumber(int i) {
boolean isRotated = false;
for (final char c : String.valueOf(i).toCharArray()) {
if (c == '0' || c == '1' || c == '8')
continue;
if (c == '2' || c == '5' || c == '6' || c == '9')
isRotated = true;
else
return false;
}
return isRotated;
}
}
// code provided by PROGIEZ
788. Rotated Digits LeetCode Solution in Python
class Solution:
def rotatedDigits(self, n: int) -> int:
def isGoodNumber(i: int) -> bool:
isRotated = False
for c in str(i):
if c == '0' or c == '1' or c == '8':
continue
if c == '2' or c == '5' or c == '6' or c == '9':
isRotated = True
else:
return False
return isRotated
return sum(isGoodNumber(i) for i in range(1, n + 1))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.