33. Search in Rotated Sorted Array LeetCode Solution
In this guide we will provide 33. Search in Rotated Sorted Array LeetCode Solution with best time and space complexity. The solution to Search in Rotated Sorted Array problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Search in Rotated Sorted Array solution in C++
- Search in Rotated Sorted Array soution in Java
- Search in Rotated Sorted Array solution Python
- Additional Resources
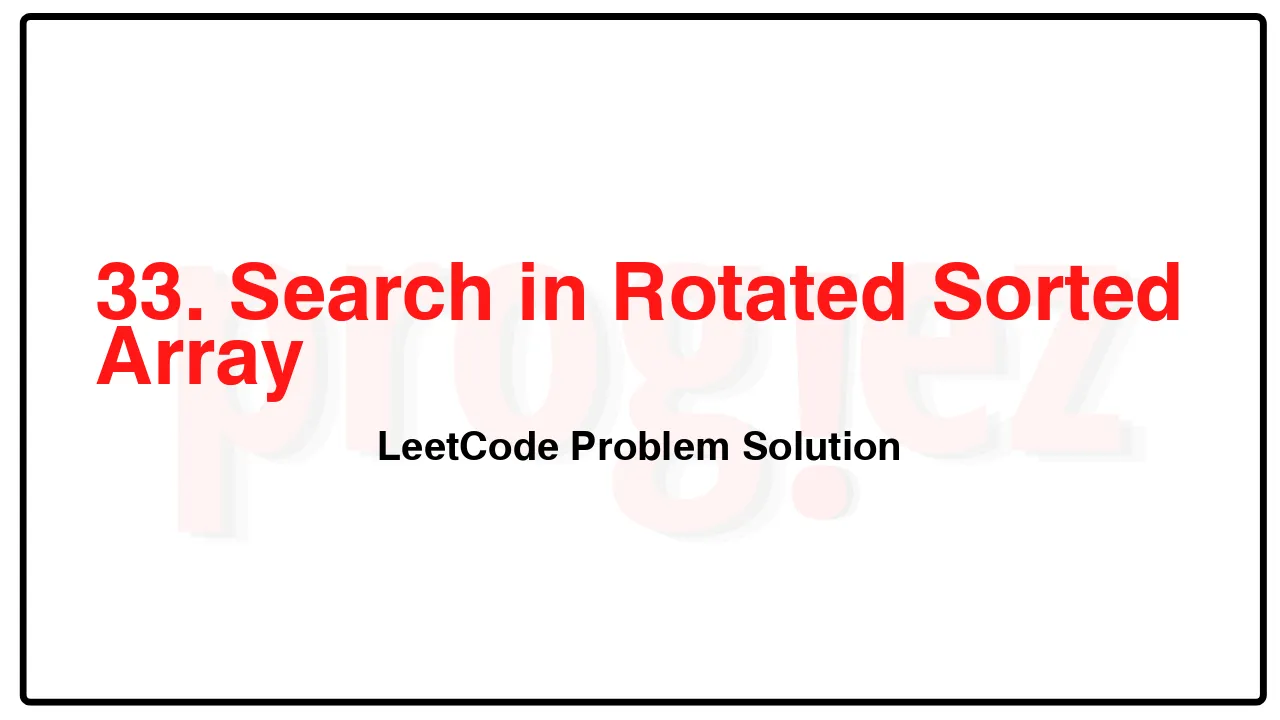
Problem Statement of Search in Rotated Sorted Array
There is an integer array nums sorted in ascending order (with distinct values).
Prior to being passed to your function, nums is possibly rotated at an unknown pivot index k (1 <= k < nums.length) such that the resulting array is [nums[k], nums[k+1], …, nums[n-1], nums[0], nums[1], …, nums[k-1]] (0-indexed). For example, [0,1,2,4,5,6,7] might be rotated at pivot index 3 and become [4,5,6,7,0,1,2].
Given the array nums after the possible rotation and an integer target, return the index of target if it is in nums, or -1 if it is not in nums.
You must write an algorithm with O(log n) runtime complexity.
Example 1:
Input: nums = [4,5,6,7,0,1,2], target = 0
Output: 4
Example 2:
Input: nums = [4,5,6,7,0,1,2], target = 3
Output: -1
Example 3:
Input: nums = [1], target = 0
Output: -1
Constraints:
1 <= nums.length <= 5000
-104 <= nums[i] <= 104
All values of nums are unique.
nums is an ascending array that is possibly rotated.
-104 <= target <= 104
Complexity Analysis
- Time Complexity: O(\log n)
- Space Complexity: O(1)
33. Search in Rotated Sorted Array LeetCode Solution in C++
class Solution {
public:
int search(vector<int>& nums, int target) {
int l = 0;
int r = nums.size() - 1;
while (l <= r) {
const int m = (l + r) / 2;
if (nums[m] == target)
return m;
if (nums[l] <= nums[m]) { // nums[l..m] are sorted.
if (nums[l] <= target && target < nums[m])
r = m - 1;
else
l = m + 1;
} else { // nums[m..n - 1] are sorted.
if (nums[m] < target && target <= nums[r])
l = m + 1;
else
r = m - 1;
}
}
return -1;
}
};
/* code provided by PROGIEZ */
33. Search in Rotated Sorted Array LeetCode Solution in Java
class Solution {
public int search(int[] nums, int target) {
int l = 0;
int r = nums.length - 1;
while (l <= r) {
final int m = (l + r) / 2;
if (nums[m] == target)
return m;
if (nums[l] <= nums[m]) { // nums[l..m] are sorted.
if (nums[l] <= target && target < nums[m])
r = m - 1;
else
l = m + 1;
} else { // nums[m..n - 1] are sorted.
if (nums[m] < target && target <= nums[r])
l = m + 1;
else
r = m - 1;
}
}
return -1;
}
}
// code provided by PROGIEZ
33. Search in Rotated Sorted Array LeetCode Solution in Python
class Solution:
def search(self, nums: list[int], target: int) -> int:
l = 0
r = len(nums) - 1
while l <= r:
m = (l + r) // 2
if nums[m] == target:
return m
if nums[l] <= nums[m]: # nums[l..m] are sorted.
if nums[l] <= target < nums[m]:
r = m - 1
else:
l = m + 1
else: # nums[m..n - 1] are sorted.
if nums[m] < target <= nums[r]:
l = m + 1
else:
r = m - 1
return -1
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us