2. Add Two Numbers LeetCode Solution
In this guide we will provide 2. Add Two Numbers LeetCode Solution with best time and space complexity. The solution to Add Two Numbers problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Add Two Numbers solution in C++
- Add Two Numbers soution in Java
- Add Two Numbers solution Python
- Additional Resources
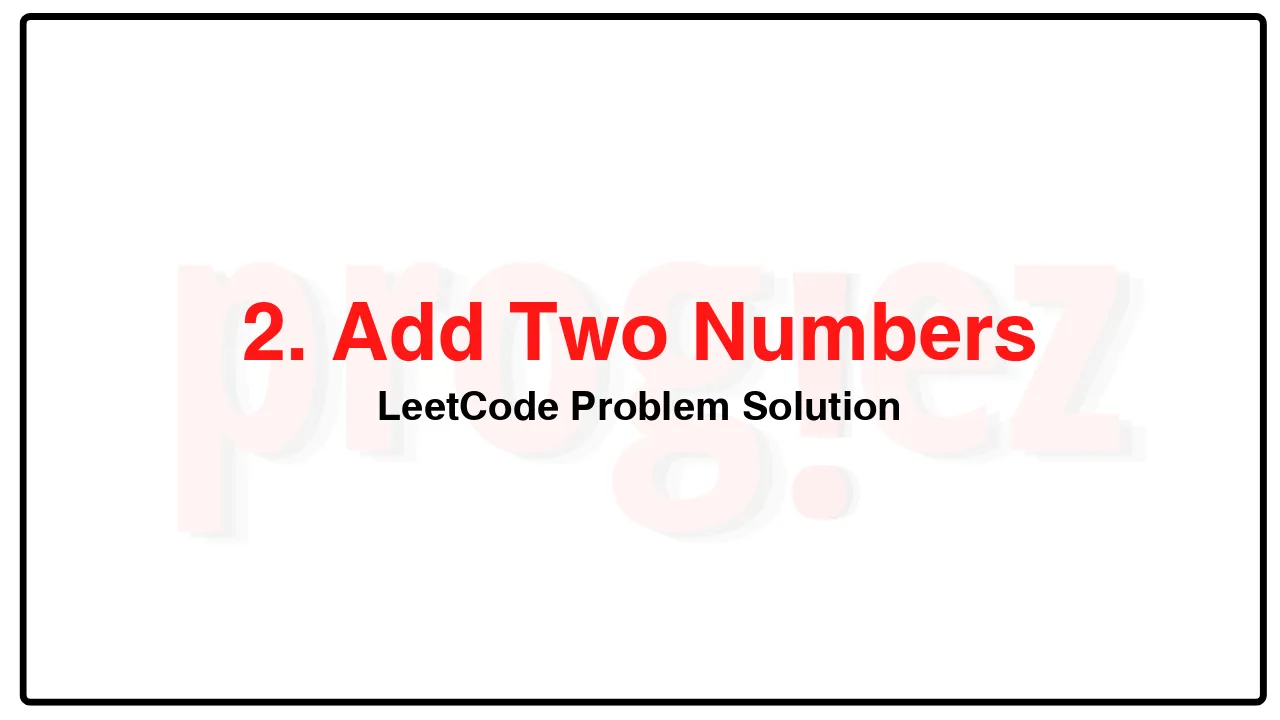
Problem Statement of Add Two Numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example 1:
Input: l1 = [2,4,3], l2 = [5,6,4]
Output: [7,0,8]
Explanation: 342 + 465 = 807.
Example 2:
Input: l1 = [0], l2 = [0]
Output: [0]
Example 3:
Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9]
Output: [8,9,9,9,0,0,0,1]
Constraints:
The number of nodes in each linked list is in the range [1, 100].
0 <= Node.val <= 9
It is guaranteed that the list represents a number that does not have leading zeros.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
2. Add Two Numbers LeetCode Solution in C++
class Solution {
public:
ListNode* addTwoNumbers(ListNode* l1, ListNode* l2) {
ListNode dummy(0);
ListNode* curr = &dummy;
int carry = 0;
while (l1 != nullptr || l2 != nullptr || carry > 0) {
if (l1 != nullptr) {
carry += l1->val;
l1 = l1->next;
}
if (l2 != nullptr) {
carry += l2->val;
l2 = l2->next;
}
curr->next = new ListNode(carry % 10);
carry /= 10;
curr = curr->next;
}
return dummy.next;
}
};
/* code provided by PROGIEZ */
2. Add Two Numbers LeetCode Solution in Java
class Solution {
public ListNode addTwoNumbers(ListNode l1, ListNode l2) {
ListNode dummy = new ListNode(0);
ListNode curr = dummy;
int carry = 0;
while (l1 != null || l2 != null || carry > 0) {
if (l1 != null) {
carry += l1.val;
l1 = l1.next;
}
if (l2 != null) {
carry += l2.val;
l2 = l2.next;
}
curr.next = new ListNode(carry % 10);
carry /= 10;
curr = curr.next;
}
return dummy.next;
}
}
// code provided by PROGIEZ
2. Add Two Numbers LeetCode Solution in Python
class Solution:
def addTwoNumbers(self, l1: ListNode, l2: ListNode) -> ListNode:
dummy = ListNode(0)
curr = dummy
carry = 0
while carry or l1 or l2:
if l1:
carry += l1.val
l1 = l1.next
if l2:
carry += l2.val
l2 = l2.next
curr.next = ListNode(carry % 10)
carry //= 10
curr = curr.next
return dummy.next
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us