771. Jewels and Stones LeetCode Solution
In this guide, you will get 771. Jewels and Stones LeetCode Solution with the best time and space complexity. The solution to Jewels and Stones problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Jewels and Stones solution in C++
- Jewels and Stones solution in Java
- Jewels and Stones solution in Python
- Additional Resources
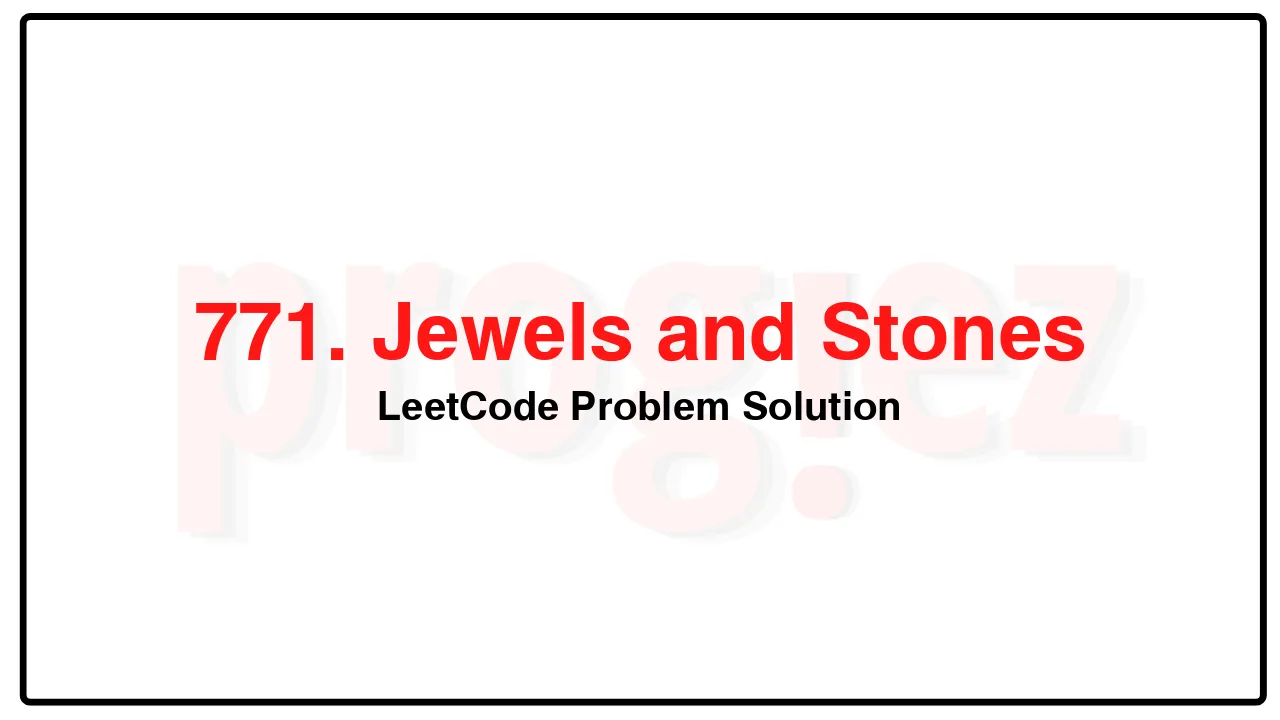
Problem Statement of Jewels and Stones
You’re given strings jewels representing the types of stones that are jewels, and stones representing the stones you have. Each character in stones is a type of stone you have. You want to know how many of the stones you have are also jewels.
Letters are case sensitive, so “a” is considered a different type of stone from “A”.
Example 1:
Input: jewels = “aA”, stones = “aAAbbbb”
Output: 3
Example 2:
Input: jewels = “z”, stones = “ZZ”
Output: 0
Constraints:
1 <= jewels.length, stones.length <= 50
jewels and stones consist of only English letters.
All the characters of jewels are unique.
Complexity Analysis
- Time Complexity: O(|\texttt{jewels}| + |\texttt{stones}|)
- Space Complexity: O(|\texttt{jewels}|)
771. Jewels and Stones LeetCode Solution in C++
class Solution {
public:
int numJewelsInStones(string jewels, string stones) {
int ans = 0;
unordered_set<char> jewelsSet(jewels.begin(), jewels.end());
for (const char stone : stones)
if (jewelsSet.contains(stone))
++ans;
return ans;
}
};
/* code provided by PROGIEZ */
771. Jewels and Stones LeetCode Solution in Java
class Solution {
public int numJewelsInStones(String jewels, String stones) {
int ans = 0;
Set<Character> jewelsSet = jewels.chars().mapToObj(c -> (char) c).collect(Collectors.toSet());
for (final char stone : stones.toCharArray())
if (jewelsSet.contains(stone))
++ans;
return ans;
}
}
// code provided by PROGIEZ
771. Jewels and Stones LeetCode Solution in Python
class Solution:
def numJewelsInStones(self, jewels: str, stones: str) -> int:
jewelsSet = set(jewels)
return sum(stone in jewelsSet for stone in stones)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.