728. Self Dividing Numbers LeetCode Solution
In this guide, you will get 728. Self Dividing Numbers LeetCode Solution with the best time and space complexity. The solution to Self Dividing Numbers problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Self Dividing Numbers solution in C++
- Self Dividing Numbers solution in Java
- Self Dividing Numbers solution in Python
- Additional Resources
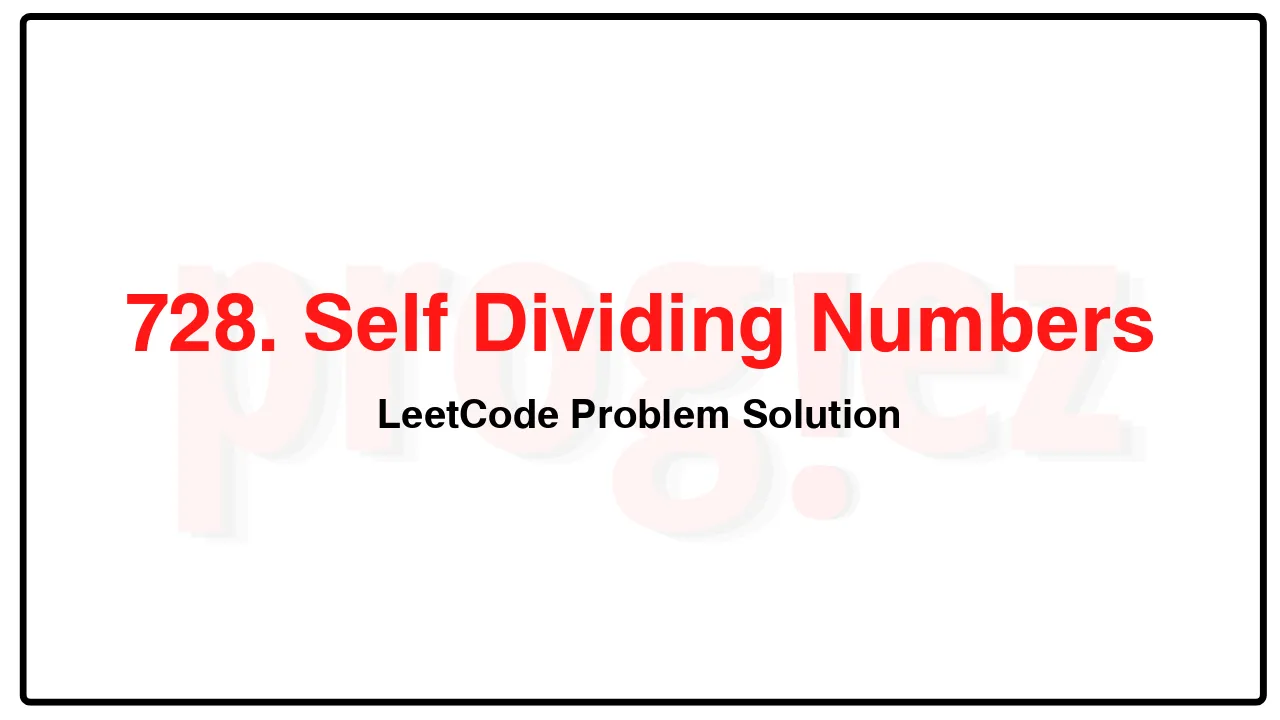
Problem Statement of Self Dividing Numbers
A self-dividing number is a number that is divisible by every digit it contains.
For example, 128 is a self-dividing number because 128 % 1 == 0, 128 % 2 == 0, and 128 % 8 == 0.
A self-dividing number is not allowed to contain the digit zero.
Given two integers left and right, return a list of all the self-dividing numbers in the range [left, right] (both inclusive).
Example 1:
Input: left = 1, right = 22
Output: [1,2,3,4,5,6,7,8,9,11,12,15,22]
Example 2:
Input: left = 47, right = 85
Output: [48,55,66,77]
Constraints:
1 <= left <= right <= 104
Complexity Analysis
- Time Complexity: O(n\log n)
- Space Complexity: O(1)
728. Self Dividing Numbers LeetCode Solution in C++
class Solution {
public:
vector<int> selfDividingNumbers(int left, int right) {
vector<int> ans;
for (int num = left; num <= right; ++num)
if (selfDividingNumbers(num))
ans.push_back(num);
return ans;
}
private:
bool selfDividingNumbers(int num) {
for (int n = num; n > 0; n /= 10)
if (n % 10 == 0 || num % (n % 10) != 0)
return false;
return true;
}
};
/* code provided by PROGIEZ */
728. Self Dividing Numbers LeetCode Solution in Java
class Solution {
public List<Integer> selfDividingNumbers(int left, int right) {
List<Integer> ans = new ArrayList<>();
for (int num = left; num <= right; ++num)
if (dividingNumber(num))
ans.add(num);
return ans;
}
private boolean dividingNumber(int num) {
for (int n = num; n > 0; n /= 10)
if (n % 10 == 0 || num % (n % 10) != 0)
return false;
return true;
}
}
// code provided by PROGIEZ
728. Self Dividing Numbers LeetCode Solution in Python
class Solution:
def selfDividingNumbers(self, left: int, right: int) -> list[int]:
return [num for num in range(left, right + 1) if all(n != 0 and num % n == 0 for n in map(int, str(num)))]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.