605. Can Place Flowers LeetCode Solution
In this guide, you will get 605. Can Place Flowers LeetCode Solution with the best time and space complexity. The solution to Can Place Flowers problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Can Place Flowers solution in C++
- Can Place Flowers solution in Java
- Can Place Flowers solution in Python
- Additional Resources
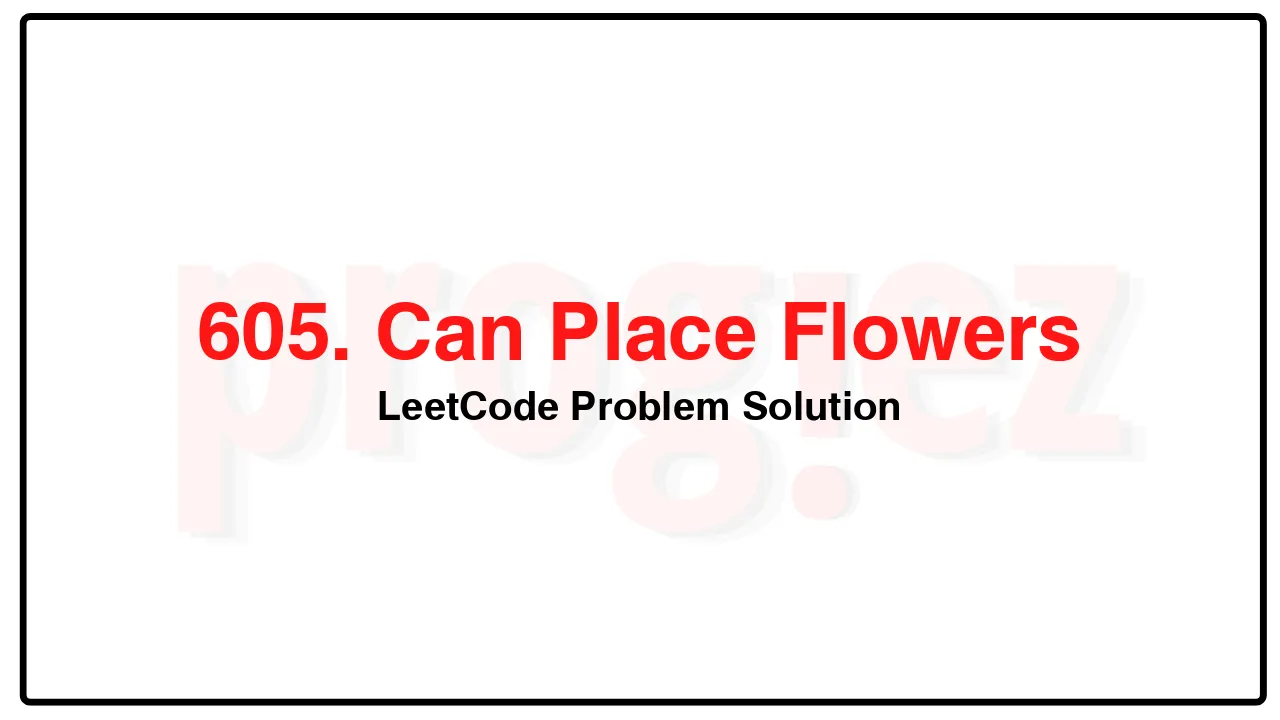
Problem Statement of Can Place Flowers
You have a long flowerbed in which some of the plots are planted, and some are not. However, flowers cannot be planted in adjacent plots.
Given an integer array flowerbed containing 0’s and 1’s, where 0 means empty and 1 means not empty, and an integer n, return true if n new flowers can be planted in the flowerbed without violating the no-adjacent-flowers rule and false otherwise.
Example 1:
Input: flowerbed = [1,0,0,0,1], n = 1
Output: true
Example 2:
Input: flowerbed = [1,0,0,0,1], n = 2
Output: false
Constraints:
1 <= flowerbed.length <= 2 * 104
flowerbed[i] is 0 or 1.
There are no two adjacent flowers in flowerbed.
0 <= n <= flowerbed.length
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
605. Can Place Flowers LeetCode Solution in C++
class Solution {
public:
bool canPlaceFlowers(vector<int>& flowerbed, int n) {
if (n == 0)
return true;
for (int i = 0; i < flowerbed.size(); ++i)
if (flowerbed[i] == 0 && (i == 0 || flowerbed[i - 1] == 0) &&
(i == flowerbed.size() - 1 || flowerbed[i + 1] == 0)) {
flowerbed[i] = 1;
if (--n == 0)
return true;
}
return false;
}
};
/* code provided by PROGIEZ */
605. Can Place Flowers LeetCode Solution in Java
class Solution {
public boolean canPlaceFlowers(int[] flowerbed, int n) {
if (n == 0)
return true;
for (int i = 0; i < flowerbed.length; ++i)
if (flowerbed[i] == 0 && (i == 0 || flowerbed[i - 1] == 0) &&
(i == flowerbed.length - 1 || flowerbed[i + 1] == 0)) {
flowerbed[i] = 1;
if (--n == 0)
return true;
}
return false;
}
}
// code provided by PROGIEZ
605. Can Place Flowers LeetCode Solution in Python
class Solution:
def canPlaceFlowers(self, flowerbed: list[int], n: int) -> bool:
for i, flower in enumerate(flowerbed):
if flower == 0 and (
i == 0 or flowerbed[i - 1] == 0) and (
i == len(flowerbed) - 1 or flowerbed[i + 1] == 0):
flowerbed[i] = 1
n -= 1
if n <= 0:
return True
return False
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.