453. Minimum Moves to Equal Array Elements LeetCode Solution
In this guide, you will get 453. Minimum Moves to Equal Array Elements LeetCode Solution with the best time and space complexity. The solution to Minimum Moves to Equal Array Elements problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Moves to Equal Array Elements solution in C++
- Minimum Moves to Equal Array Elements solution in Java
- Minimum Moves to Equal Array Elements solution in Python
- Additional Resources
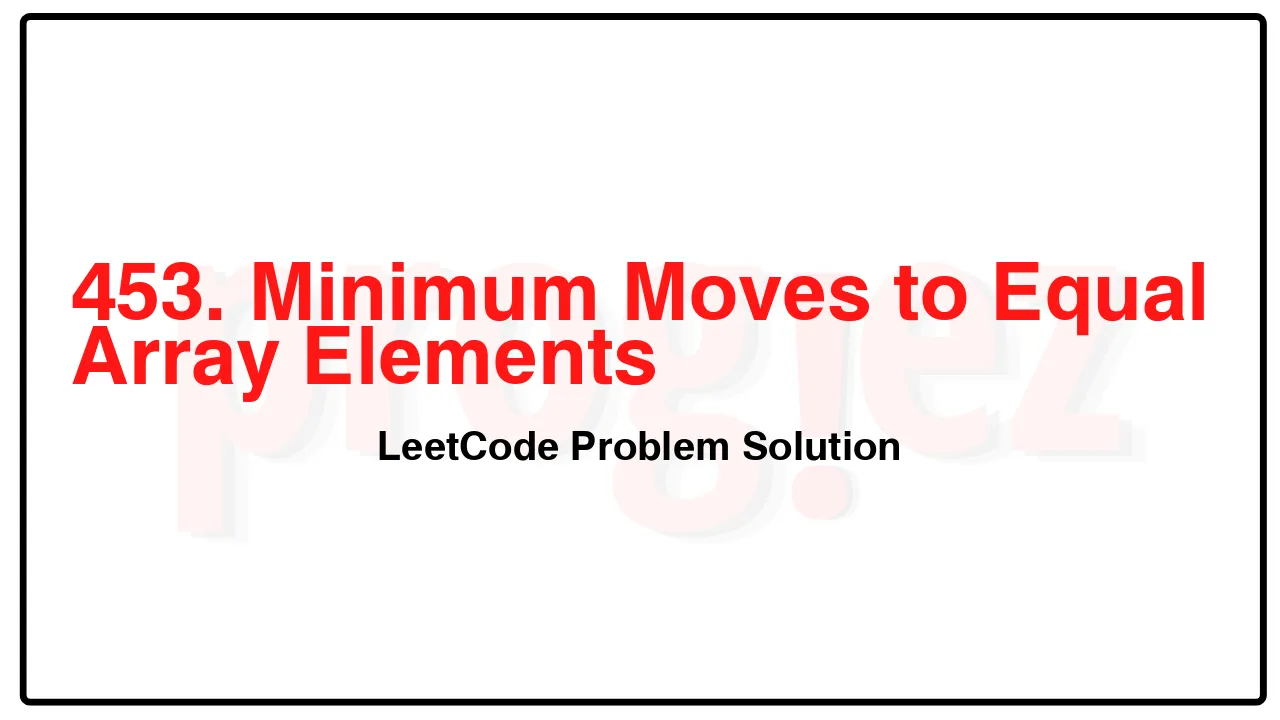
Problem Statement of Minimum Moves to Equal Array Elements
Given an integer array nums of size n, return the minimum number of moves required to make all array elements equal.
In one move, you can increment n – 1 elements of the array by 1.
Example 1:
Input: nums = [1,2,3]
Output: 3
Explanation: Only three moves are needed (remember each move increments two elements):
[1,2,3] => [2,3,3] => [3,4,3] => [4,4,4]
Example 2:
Input: nums = [1,1,1]
Output: 0
Constraints:
n == nums.length
1 <= nums.length <= 105
-109 <= nums[i] <= 109
The answer is guaranteed to fit in a 32-bit integer.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
453. Minimum Moves to Equal Array Elements LeetCode Solution in C++
class Solution {
public:
int minMoves(vector<int>& nums) {
const int mn = ranges::min(nums);
return accumulate(nums.begin(), nums.end(), 0, [&](int subtotal, int num) {
return subtotal + (num - mn);
});
}
};
/* code provided by PROGIEZ */
453. Minimum Moves to Equal Array Elements LeetCode Solution in Java
class Solution {
public int minMoves(int[] nums) {
final int sum = Arrays.stream(nums).sum();
final int mn = Arrays.stream(nums).min().getAsInt();
return sum - mn * nums.length;
}
}
// code provided by PROGIEZ
453. Minimum Moves to Equal Array Elements LeetCode Solution in Python
class Solution:
def minMoves(self, nums: list[int]) -> int:
mn = min(nums)
return sum(num - mn for num in nums)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.