2373. Largest Local Values in a Matrix LeetCode Solution
In this guide, you will get 2373. Largest Local Values in a Matrix LeetCode Solution with the best time and space complexity. The solution to Largest Local Values in a Matrix problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Largest Local Values in a Matrix solution in C++
- Largest Local Values in a Matrix solution in Java
- Largest Local Values in a Matrix solution in Python
- Additional Resources
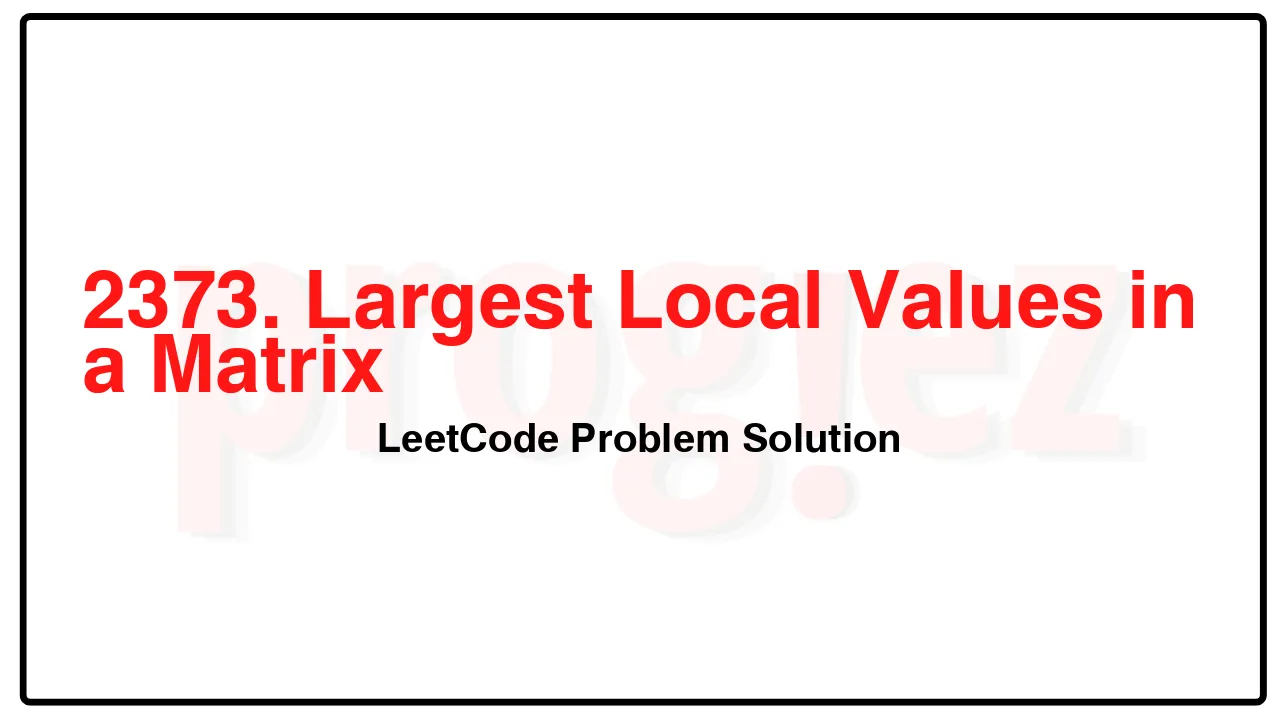
Problem Statement of Largest Local Values in a Matrix
You are given an n x n integer matrix grid.
Generate an integer matrix maxLocal of size (n – 2) x (n – 2) such that:
maxLocal[i][j] is equal to the largest value of the 3 x 3 matrix in grid centered around row i + 1 and column j + 1.
In other words, we want to find the largest value in every contiguous 3 x 3 matrix in grid.
Return the generated matrix.
Example 1:
Input: grid = [[9,9,8,1],[5,6,2,6],[8,2,6,4],[6,2,2,2]]
Output: [[9,9],[8,6]]
Explanation: The diagram above shows the original matrix and the generated matrix.
Notice that each value in the generated matrix corresponds to the largest value of a contiguous 3 x 3 matrix in grid.
Example 2:
Input: grid = [[1,1,1,1,1],[1,1,1,1,1],[1,1,2,1,1],[1,1,1,1,1],[1,1,1,1,1]]
Output: [[2,2,2],[2,2,2],[2,2,2]]
Explanation: Notice that the 2 is contained within every contiguous 3 x 3 matrix in grid.
Constraints:
n == grid.length == grid[i].length
3 <= n <= 100
1 <= grid[i][j] <= 100
Complexity Analysis
- Time Complexity: O(mn)
- Space Complexity: O(mn)
2373. Largest Local Values in a Matrix LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> largestLocal(vector<vector<int>>& grid) {
const int n = grid.size();
vector<vector<int>> ans(n - 2, vector<int>(n - 2));
for (int i = 0; i < n - 2; ++i)
for (int j = 0; j < n - 2; ++j)
for (int x = i; x < i + 3; ++x)
for (int y = j; y < j + 3; ++y)
ans[i][j] = max(ans[i][j], grid[x][y]);
return ans;
}
};
/* code provided by PROGIEZ */
2373. Largest Local Values in a Matrix LeetCode Solution in Java
class Solution {
public int[][] largestLocal(int[][] grid) {
final int n = grid.length;
int[][] ans = new int[n - 2][n - 2];
for (int i = 0; i < n - 2; ++i)
for (int j = 0; j < n - 2; ++j)
for (int x = i; x < i + 3; ++x)
for (int y = j; y < j + 3; ++y)
ans[i][j] = Math.max(ans[i][j], grid[x][y]);
return ans;
}
}
// code provided by PROGIEZ
2373. Largest Local Values in a Matrix LeetCode Solution in Python
class Solution:
def largestLocal(self, grid: list[list[int]]) -> list[list[int]]:
n = len(grid)
ans = [[0] * (n - 2) for _ in range(n - 2)]
for i in range(n - 2):
for j in range(n - 2):
for x in range(i, i + 3):
for y in range(j, j + 3):
ans[i][j] = max(ans[i][j], grid[x][y])
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.