925. Long Pressed Name LeetCode Solution
In this guide, you will get 925. Long Pressed Name LeetCode Solution with the best time and space complexity. The solution to Long Pressed Name problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Long Pressed Name solution in C++
- Long Pressed Name solution in Java
- Long Pressed Name solution in Python
- Additional Resources
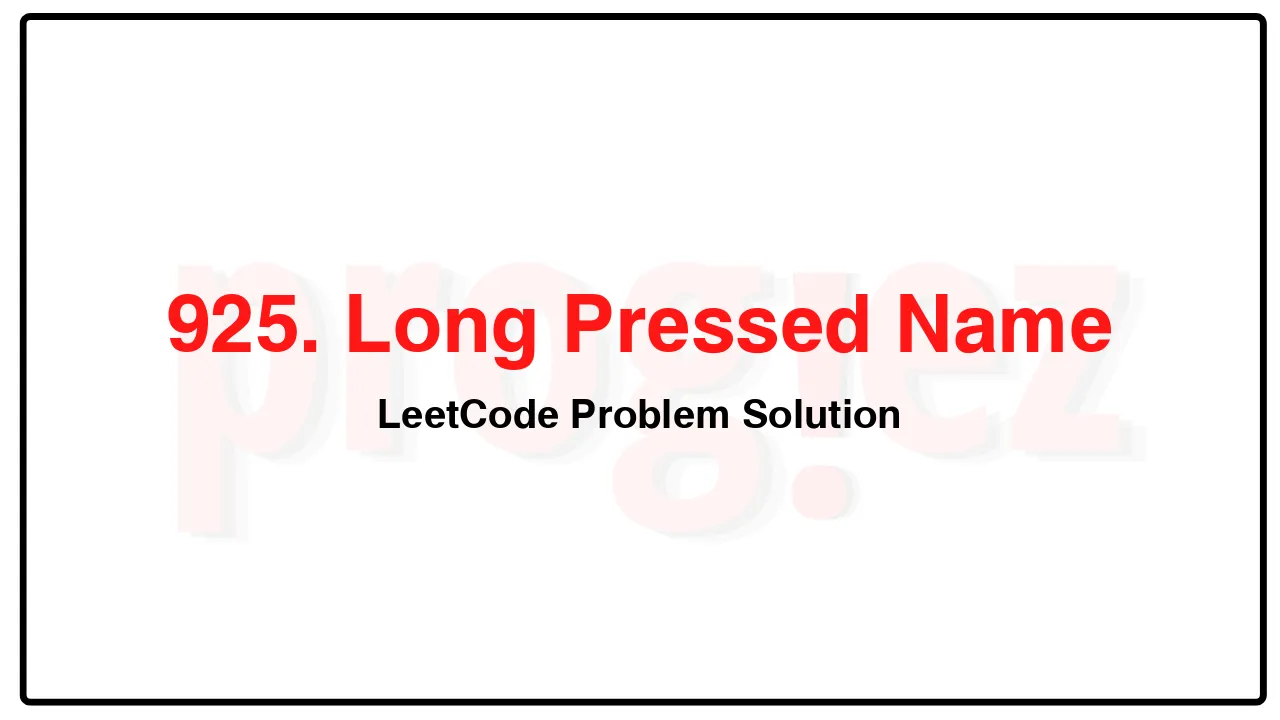
Problem Statement of Long Pressed Name
Your friend is typing his name into a keyboard. Sometimes, when typing a character c, the key might get long pressed, and the character will be typed 1 or more times.
You examine the typed characters of the keyboard. Return True if it is possible that it was your friends name, with some characters (possibly none) being long pressed.
Example 1:
Input: name = “alex”, typed = “aaleex”
Output: true
Explanation: ‘a’ and ‘e’ in ‘alex’ were long pressed.
Example 2:
Input: name = “saeed”, typed = “ssaaedd”
Output: false
Explanation: ‘e’ must have been pressed twice, but it was not in the typed output.
Constraints:
1 <= name.length, typed.length <= 1000
name and typed consist of only lowercase English letters.
Complexity Analysis
- Time Complexity:
- Space Complexity:
925. Long Pressed Name LeetCode Solution in C++
class Solution {
public:
bool isLongPressedName(string name, string typed) {
int i = 0;
for (int j = 0; j < typed.length(); ++j)
if (i < name.length() && name[i] == typed[j])
++i;
else if (j == 0 || typed[j] != typed[j - 1])
return false;
return i == name.length();
}
};
/* code provided by PROGIEZ */
925. Long Pressed Name LeetCode Solution in Java
class Solution {
public boolean isLongPressedName(String name, String typed) {
int i = 0;
for (int j = 0; j < typed.length(); ++j)
if (i < name.length() && name.charAt(i) == typed.charAt(j))
++i;
else if (j == 0 || typed.charAt(j) != typed.charAt(j - 1))
return false;
return i == name.length();
}
}
// code provided by PROGIEZ
925. Long Pressed Name LeetCode Solution in Python
class Solution:
def isLongPressedName(self, name: str, typed: str) -> bool:
i = 0
for j, t in enumerate(typed):
if i < len(name) and name[i] == t:
i += 1
elif j == 0 or t != typed[j - 1]:
return False
return i == len(name)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.