869. Reordered Power of 2 LeetCode Solution
In this guide, you will get 869. Reordered Power of 2 LeetCode Solution with the best time and space complexity. The solution to Reordered Power of problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Reordered Power of solution in C++
- Reordered Power of solution in Java
- Reordered Power of solution in Python
- Additional Resources
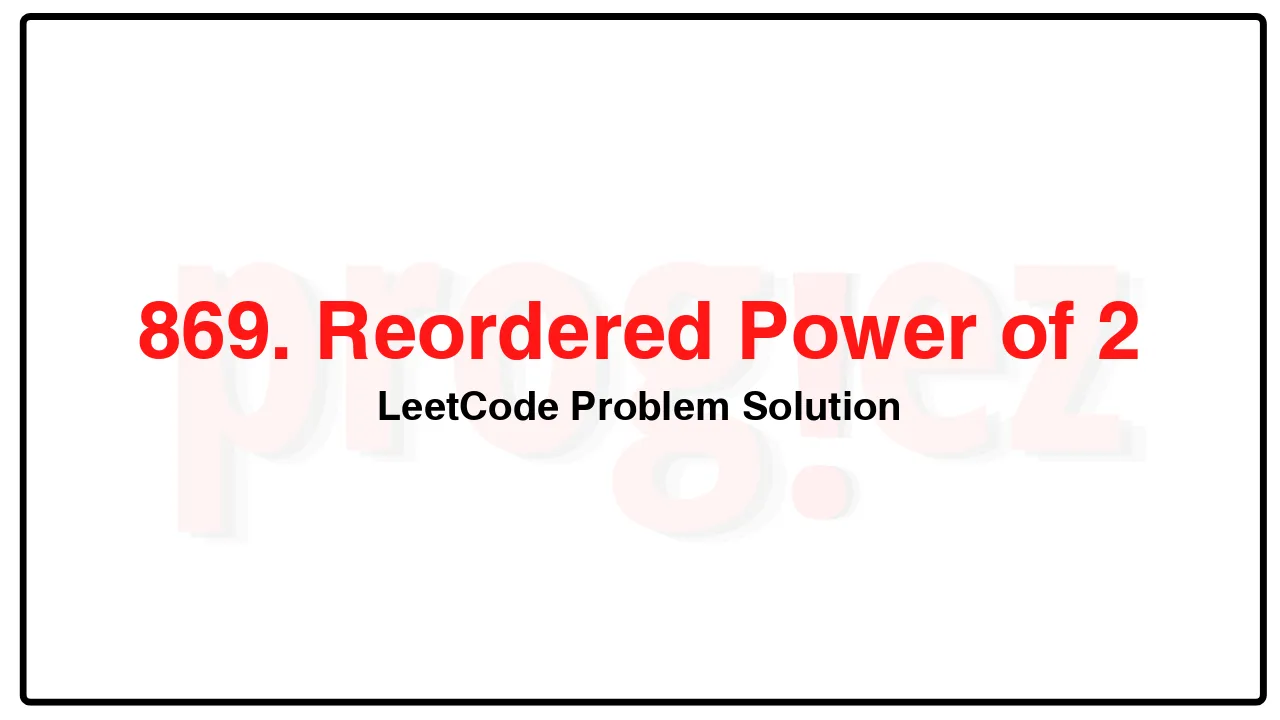
Problem Statement of Reordered Power of
You are given an integer n. We reorder the digits in any order (including the original order) such that the leading digit is not zero.
Return true if and only if we can do this so that the resulting number is a power of two.
Example 1:
Input: n = 1
Output: true
Example 2:
Input: n = 10
Output: false
Constraints:
1 <= n <= 109
Complexity Analysis
- Time Complexity:
- Space Complexity:
869. Reordered Power of 2 LeetCode Solution in C++
class Solution {
public:
bool reorderedPowerOf2(int n) {
int count = counter(n);
for (int i = 0; i < 30; ++i)
if (counter(1 << i) == count)
return true;
return false;
}
private:
int counter(int n) {
int count = 0;
for (; n > 0; n /= 10)
count += pow(10, n % 10);
return count;
}
};
/* code provided by PROGIEZ */
869. Reordered Power of 2 LeetCode Solution in Java
class Solution {
public boolean reorderedPowerOf2(int n) {
int count = counter(n);
for (int i = 0; i < 30; ++i)
if (counter(1 << i) == count)
return true;
return false;
}
private int counter(int n) {
int count = 0;
for (; n > 0; n /= 10)
count += Math.pow(10, n % 10);
return count;
}
}
// code provided by PROGIEZ
869. Reordered Power of 2 LeetCode Solution in Python
class Solution:
def reorderedPowerOf2(self, n: int) -> bool:
count = collections.Counter(str(n))
return any(Counter(str(1 << i)) == count for i in range(30))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.