238. Product of Array Except Self LeetCode Solution
In this guide, you will get 238. Product of Array Except Self LeetCode Solution with the best time and space complexity. The solution to Product of Array Except Self problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Product of Array Except Self solution in C++
- Product of Array Except Self solution in Java
- Product of Array Except Self solution in Python
- Additional Resources
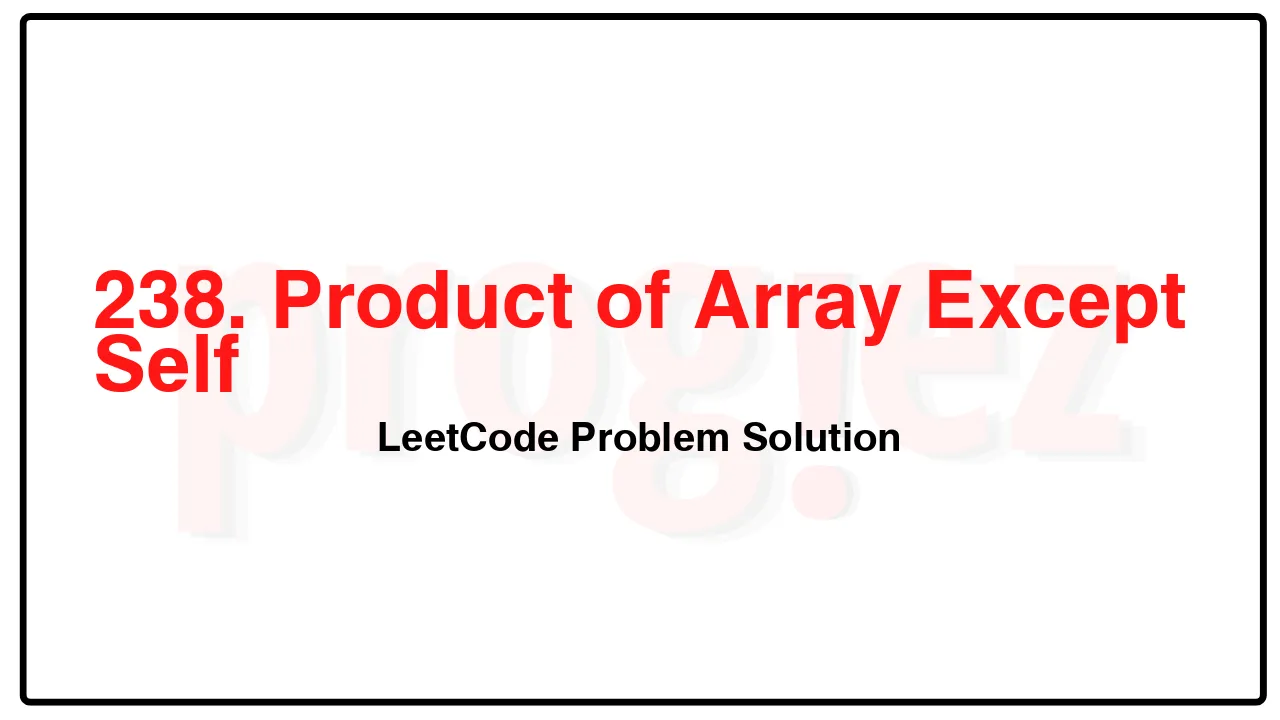
Problem Statement of Product of Array Except Self
Given an integer array nums, return an array answer such that answer[i] is equal to the product of all the elements of nums except nums[i].
The product of any prefix or suffix of nums is guaranteed to fit in a 32-bit integer.
You must write an algorithm that runs in O(n) time and without using the division operation.
Example 1:
Input: nums = [1,2,3,4]
Output: [24,12,8,6]
Example 2:
Input: nums = [-1,1,0,-3,3]
Output: [0,0,9,0,0]
Constraints:
2 <= nums.length <= 105
-30 <= nums[i] <= 30
The input is generated such that answer[i] is guaranteed to fit in a 32-bit integer.
Follow up: Can you solve the problem in O(1) extra space complexity? (The output array does not count as extra space for space complexity analysis.)
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
238. Product of Array Except Self LeetCode Solution in C++
class Solution {
public:
vector<int> productExceptSelf(vector<int>& nums) {
const int n = nums.size();
vector<int> ans(n); // Can also use `nums` as the ans array.
vector<int> prefix(n, 1); // prefix product
vector<int> suffix(n, 1); // suffix product
for (int i = 1; i < n; ++i)
prefix[i] = prefix[i - 1] * nums[i - 1];
for (int i = n - 2; i >= 0; --i)
suffix[i] = suffix[i + 1] * nums[i + 1];
for (int i = 0; i < n; ++i)
ans[i] = prefix[i] * suffix[i];
return ans;
}
};
/* code provided by PROGIEZ */
238. Product of Array Except Self LeetCode Solution in Java
class Solution {
public int[] productExceptSelf(int[] nums) {
final int n = nums.length;
int[] ans = new int[n]; // Can also use `nums` as the ans array.
int[] prefix = new int[n]; // prefix product
int[] suffix = new int[n]; // suffix product
prefix[0] = 1;
for (int i = 1; i < n; ++i)
prefix[i] = prefix[i - 1] * nums[i - 1];
suffix[n - 1] = 1;
for (int i = n - 2; i >= 0; --i)
suffix[i] = suffix[i + 1] * nums[i + 1];
for (int i = 0; i < n; ++i)
ans[i] = prefix[i] * suffix[i];
return ans;
}
}
// code provided by PROGIEZ
238. Product of Array Except Self LeetCode Solution in Python
class Solution:
def productExceptSelf(self, nums: list[int]) -> list[int]:
n = len(nums)
prefix = [1] * n # prefix product
suffix = [1] * n # suffix product
for i in range(1, n):
prefix[i] = prefix[i - 1] * nums[i - 1]
for i in reversed(range(n - 1)):
suffix[i] = suffix[i + 1] * nums[i + 1]
return [prefix[i] * suffix[i] for i in range(n)]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.