46. Permutations LeetCode Solution
In this guide we will provide 46. Permutations LeetCode Solution with best time and space complexity. The solution to Permutations problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Permutations solution in C++
- Permutations soution in Java
- Permutations solution Python
- Additional Resources
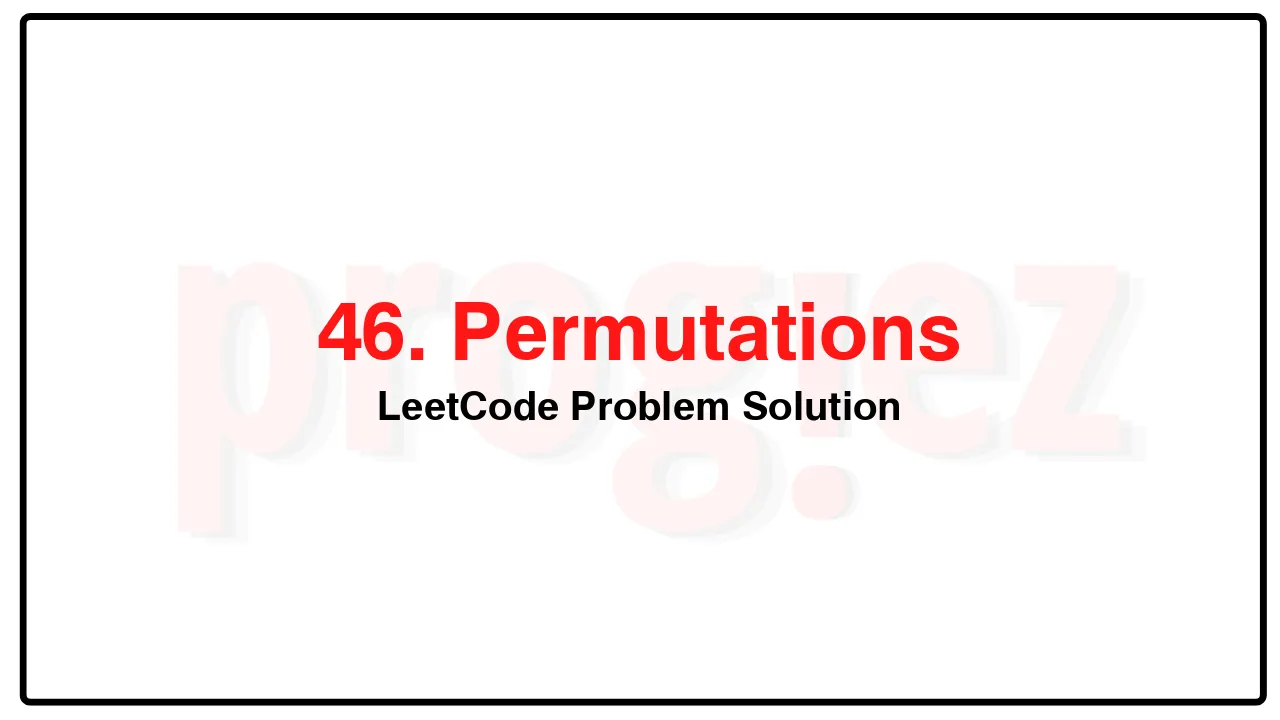
Problem Statement of Permutations
Given an array nums of distinct integers, return all the possible permutations. You can return the answer in any order.
Example 1:
Input: nums = [1,2,3]
Output: [[1,2,3],[1,3,2],[2,1,3],[2,3,1],[3,1,2],[3,2,1]]
Example 2:
Input: nums = [0,1]
Output: [[0,1],[1,0]]
Example 3:
Input: nums = [1]
Output: [[1]]
Constraints:
1 <= nums.length <= 6
-10 <= nums[i] <= 10
All the integers of nums are unique.
Complexity Analysis
- Time Complexity: O(n \cdot n!)
- Space Complexity: O(n \cdot n!)
46. Permutations LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> permute(vector<int>& nums) {
vector<vector<int>> ans;
dfs(nums, vector<bool>(nums.size()), {}, ans);
return ans;
}
private:
void dfs(const vector<int>& nums, vector<bool>&& used, vector<int>&& path,
vector<vector<int>>& ans) {
if (path.size() == nums.size()) {
ans.push_back(path);
return;
}
for (int i = 0; i < nums.size(); ++i) {
if (used[i])
continue;
used[i] = true;
path.push_back(nums[i]);
dfs(nums, std::move(used), std::move(path), ans);
path.pop_back();
used[i] = false;
}
}
};
/* code provided by PROGIEZ */
46. Permutations LeetCode Solution in Java
class Solution {
public List<List<Integer>> permute(int[] nums) {
List<List<Integer>> ans = new ArrayList<>();
dfs(nums, new boolean[nums.length], new ArrayList<>(), ans);
return ans;
}
private void dfs(int[] nums, boolean[] used, List<Integer> path, List<List<Integer>> ans) {
if (path.size() == nums.length) {
ans.add(new ArrayList<>(path));
return;
}
for (int i = 0; i < nums.length; ++i) {
if (used[i])
continue;
used[i] = true;
path.add(nums[i]);
dfs(nums, used, path, ans);
path.remove(path.size() - 1);
used[i] = false;
}
}
}
// code provided by PROGIEZ
46. Permutations LeetCode Solution in Python
class Solution:
def permute(self, nums: list[int]) -> list[list[int]]:
ans = []
used = [False] * len(nums)
def dfs(path: list[int]) -> None:
if len(path) == len(nums):
ans.append(path.copy())
return
for i, num in enumerate(nums):
if used[i]:
continue
used[i] = True
path.append(num)
dfs(path)
path.pop()
used[i] = False
dfs([])
return ans
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us