18. 4Sum LeetCode Solution
In this guide we will provide 18. 4Sum LeetCode Solution with best time and space complexity. The solution to Sum problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
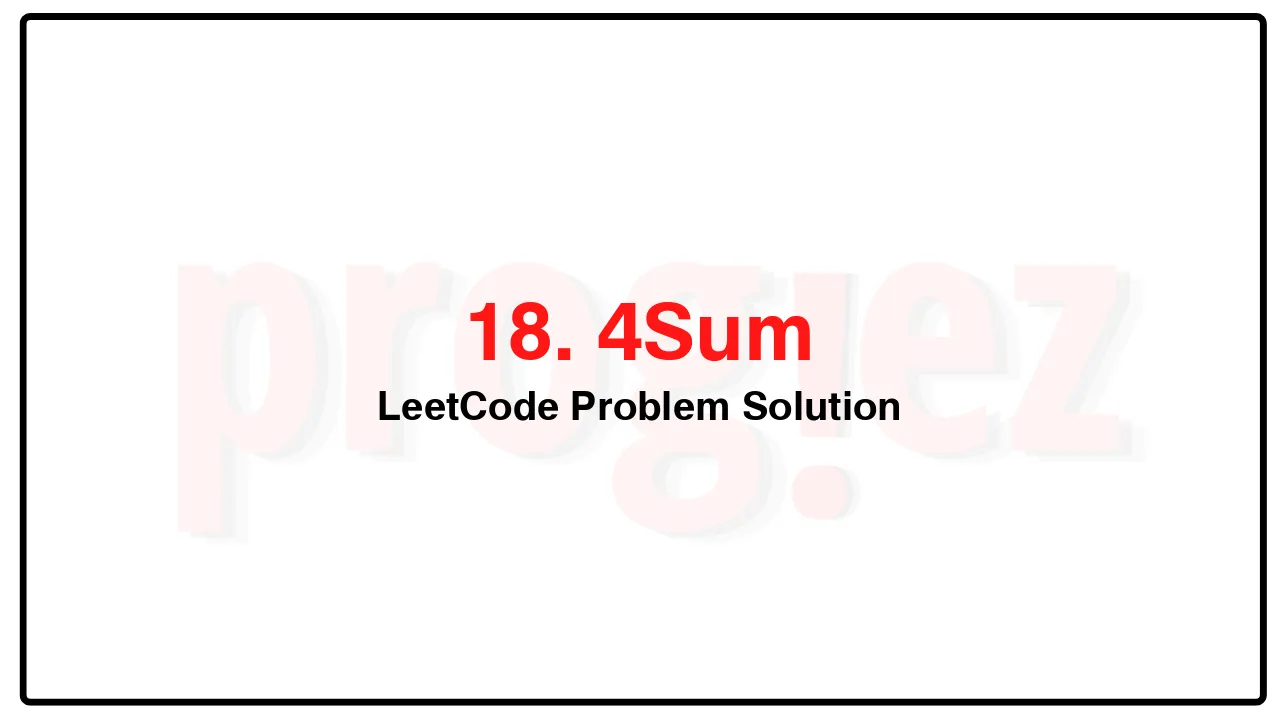
Problem Statement of Sum
Given an array nums of n integers, return an array of all the unique quadruplets [nums[a], nums[b], nums[c], nums[d]] such that:
0 <= a, b, c, d < n
a, b, c, and d are distinct.
nums[a] + nums[b] + nums[c] + nums[d] == target
You may return the answer in any order.
Example 1:
Input: nums = [1,0,-1,0,-2,2], target = 0
Output: [[-2,-1,1,2],[-2,0,0,2],[-1,0,0,1]]
Example 2:
Input: nums = [2,2,2,2,2], target = 8
Output: [[2,2,2,2]]
Constraints:
1 <= nums.length <= 200
-109 <= nums[i] <= 109
-109 <= target <= 109
Complexity Analysis
- Time Complexity: O(n^3)
- Space Complexity: O(1)
18. 4Sum LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> fourSum(vector<int>& nums, int target) {
vector<vector<int>> ans;
vector<int> path;
ranges::sort(nums);
nSum(nums, 4, target, 0, nums.size() - 1, path, ans);
return ans;
}
private:
// Finds n numbers that add up to the target in [l, r].
void nSum(const vector<int>& nums, long n, long target, int l, int r,
vector<int>& path, vector<vector<int>>& ans) {
if (r - l + 1 < n || target < nums[l] * n || target > nums[r] * n)
return;
if (n == 2) {
// Similar to the sub procedure in 15. 3Sum
while (l < r) {
const int sum = nums[l] + nums[r];
if (sum == target) {
path.push_back(nums[l]);
path.push_back(nums[r]);
ans.push_back(path);
path.pop_back();
path.pop_back();
++l;
--r;
while (l < r && nums[l] == nums[l - 1])
++l;
while (l < r && nums[r] == nums[r + 1])
--r;
} else if (sum < target) {
++l;
} else {
--r;
}
}
return;
}
for (int i = l; i <= r; ++i) {
if (i > l && nums[i] == nums[i - 1])
continue;
path.push_back(nums[i]);
nSum(nums, n - 1, target - nums[i], i + 1, r, path, ans);
path.pop_back();
}
}
};
/* code provided by PROGIEZ */
18. 4Sum LeetCode Solution in Java
class Solution {
public List<List<Integer>> fourSum(int[] nums, int target) {
List<List<Integer>> ans = new ArrayList<>();
Arrays.sort(nums);
nSum(nums, 4, target, 0, nums.length - 1, new ArrayList<>(), ans);
return ans;
}
// Finds n numbers that add up to the target in [l, r].
private void nSum(int[] nums, long n, long target, int l, int r, List<Integer> path,
List<List<Integer>> ans) {
if (r - l + 1 < n || target < nums[l] * n || target > nums[r] * n)
return;
if (n == 2) {
// Similar to the sub procedure in 15. 3Sum
while (l < r) {
final int sum = nums[l] + nums[r];
if (sum == target) {
path.add(nums[l]);
path.add(nums[r]);
ans.add(new ArrayList<>(path));
path.remove(path.size() - 1);
path.remove(path.size() - 1);
++l;
--r;
while (l < r && nums[l] == nums[l - 1])
++l;
while (l < r && nums[r] == nums[r + 1])
--r;
} else if (sum < target) {
++l;
} else {
--r;
}
}
return;
}
for (int i = l; i <= r; ++i) {
if (i > l && nums[i] == nums[i - 1])
continue;
path.add(nums[i]);
nSum(nums, n - 1, target - nums[i], i + 1, r, path, ans);
path.remove(path.size() - 1);
}
}
}
// code provided by PROGIEZ
18. 4Sum LeetCode Solution in Python
class Solution:
def fourSum(self, nums: list[int], target: int):
ans = []
def nSum(
l: int, r: int, target: int, n: int, path: list[int],
ans: list[list[int]]) -> None:
"""Finds n numbers that add up to the target in [l, r]."""
if r - l + 1 < n or n < 2 or target < nums[l] * n or target > nums[r] * n:
return
if n == 2:
while l < r:
summ = nums[l] + nums[r]
if summ == target:
ans.append(path + [nums[l], nums[r]])
l += 1
while nums[l] == nums[l - 1] and l < r:
l += 1
elif summ < target:
l += 1
else:
r -= 1
return
for i in range(l, r + 1):
if i > l and nums[i] == nums[i - 1]:
continue
nSum(i + 1, r, target - nums[i], n - 1, path + [nums[i]], ans)
nums.sort()
nSum(0, len(nums) - 1, target, 4, [], ans)
return ans
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us