31. Next PermutationLeetCode Solution
In this guide we will provide 31. Next PermutationLeetCode Solution with best time and space complexity. The solution to Next Permutation problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Next Permutation solution in C++
- Next Permutation soution in Java
- Next Permutation solution Python
- Additional Resources
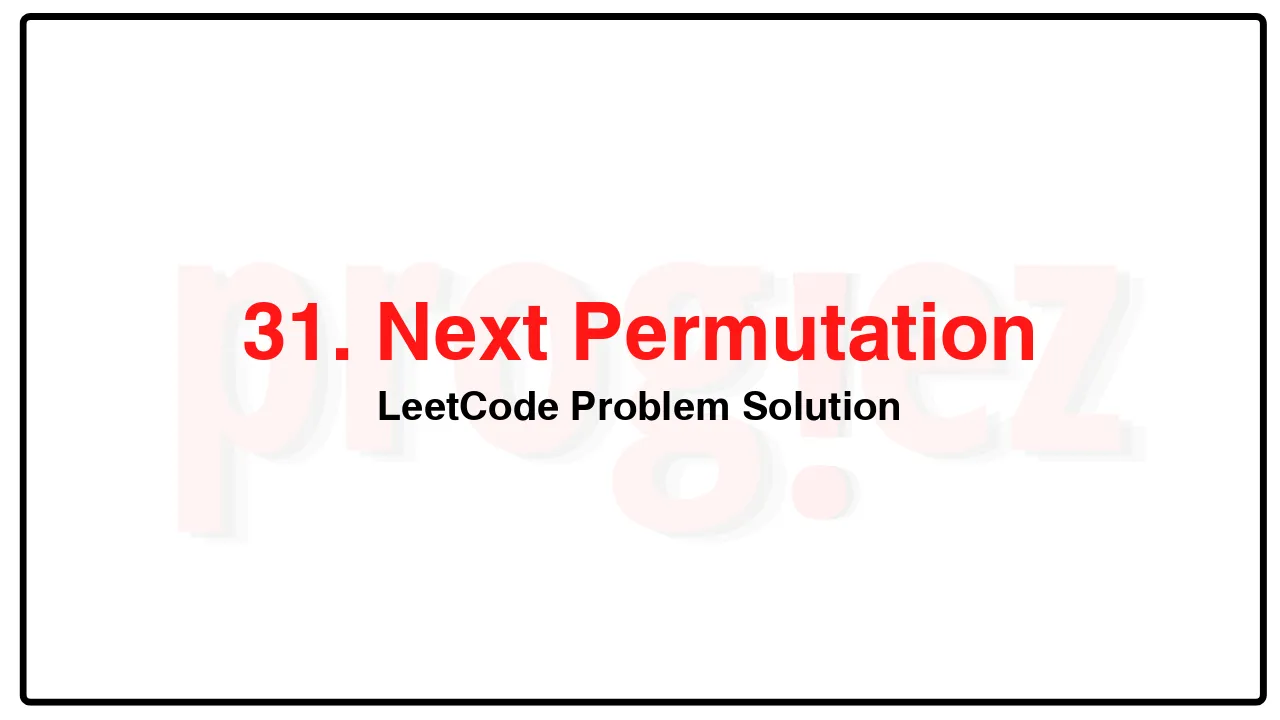
Problem Statement of Next Permutation
A permutation of an array of integers is an arrangement of its members into a sequence or linear order.
For example, for arr = [1,2,3], the following are all the permutations of arr: [1,2,3], [1,3,2], [2, 1, 3], [2, 3, 1], [3,1,2], [3,2,1].
The next permutation of an array of integers is the next lexicographically greater permutation of its integer. More formally, if all the permutations of the array are sorted in one container according to their lexicographical order, then the next permutation of that array is the permutation that follows it in the sorted container. If such arrangement is not possible, the array must be rearranged as the lowest possible order (i.e., sorted in ascending order).
For example, the next permutation of arr = [1,2,3] is [1,3,2].
Similarly, the next permutation of arr = [2,3,1] is [3,1,2].
While the next permutation of arr = [3,2,1] is [1,2,3] because [3,2,1] does not have a lexicographical larger rearrangement.
Given an array of integers nums, find the next permutation of nums.
The replacement must be in place and use only constant extra memory.
Example 1:
Input: nums = [1,2,3]
Output: [1,3,2]
Example 2:
Input: nums = [3,2,1]
Output: [1,2,3]
Example 3:
Input: nums = [1,1,5]
Output: [1,5,1]
Constraints:
1 <= nums.length <= 100
0 <= nums[i] <= 100
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
31. Next PermutationLeetCode Solution in C++
class Solution {
public:
void nextPermutation(vector<int>& nums) {
const int n = nums.size();
// From back to front, find the first number < nums[i + 1].
int i;
for (i = n - 2; i >= 0; --i)
if (nums[i] < nums[i + 1])
break;
// From back to front, find the first number > nums[i], swap it with
// nums[i].
if (i >= 0)
for (int j = n - 1; j > i; --j)
if (nums[j] > nums[i]) {
swap(nums[i], nums[j]);
break;
}
// Reverse nums[i + 1..n - 1].
reverse(nums, i + 1, n - 1);
}
private:
void reverse(vector<int>& nums, int l, int r) {
while (l < r)
swap(nums[l++], nums[r--]);
}
};
/* code provided by PROGIEZ */
31. Next PermutationLeetCode Solution in Java
class Solution {
public void nextPermutation(int[] nums) {
final int n = nums.length;
// From back to front, find the first number < nums[i + 1].
int i;
for (i = n - 2; i >= 0; --i)
if (nums[i] < nums[i + 1])
break;
// From back to front, find the first number > nums[i], swap it with
// nums[i].
if (i >= 0)
for (int j = n - 1; j > i; --j)
if (nums[j] > nums[i]) {
swap(nums, i, j);
break;
}
// Reverse nums[i + 1..n - 1].
reverse(nums, i + 1, n - 1);
}
private void reverse(int[] nums, int l, int r) {
while (l < r)
swap(nums, l++, r--);
}
private void swap(int[] nums, int i, int j) {
final int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
}
// code provided by PROGIEZ
31. Next PermutationLeetCode Solution in Python
class Solution:
def nextPermutation(self, nums: list[int]) -> None:
n = len(nums)
# From back to front, find the first number < nums[i + 1].
i = n - 2
while i >= 0:
if nums[i] < nums[i + 1]:
break
i -= 1
# From back to front, find the first number > nums[i], swap it with nums[i].
if i >= 0:
for j in range(n - 1, i, -1):
if nums[j] > nums[i]:
nums[i], nums[j] = nums[j], nums[i]
break
def reverse(nums: list[int], l: int, r: int) -> None:
while l < r:
nums[l], nums[r] = nums[r], nums[l]
l += 1
r -= 1
# Reverse nums[i + 1..n - 1].
reverse(nums, i + 1, len(nums) - 1)
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us