784. Letter Case Permutation LeetCode Solution
In this guide, you will get 784. Letter Case Permutation LeetCode Solution with the best time and space complexity. The solution to Letter Case Permutation problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Letter Case Permutation solution in C++
- Letter Case Permutation solution in Java
- Letter Case Permutation solution in Python
- Additional Resources
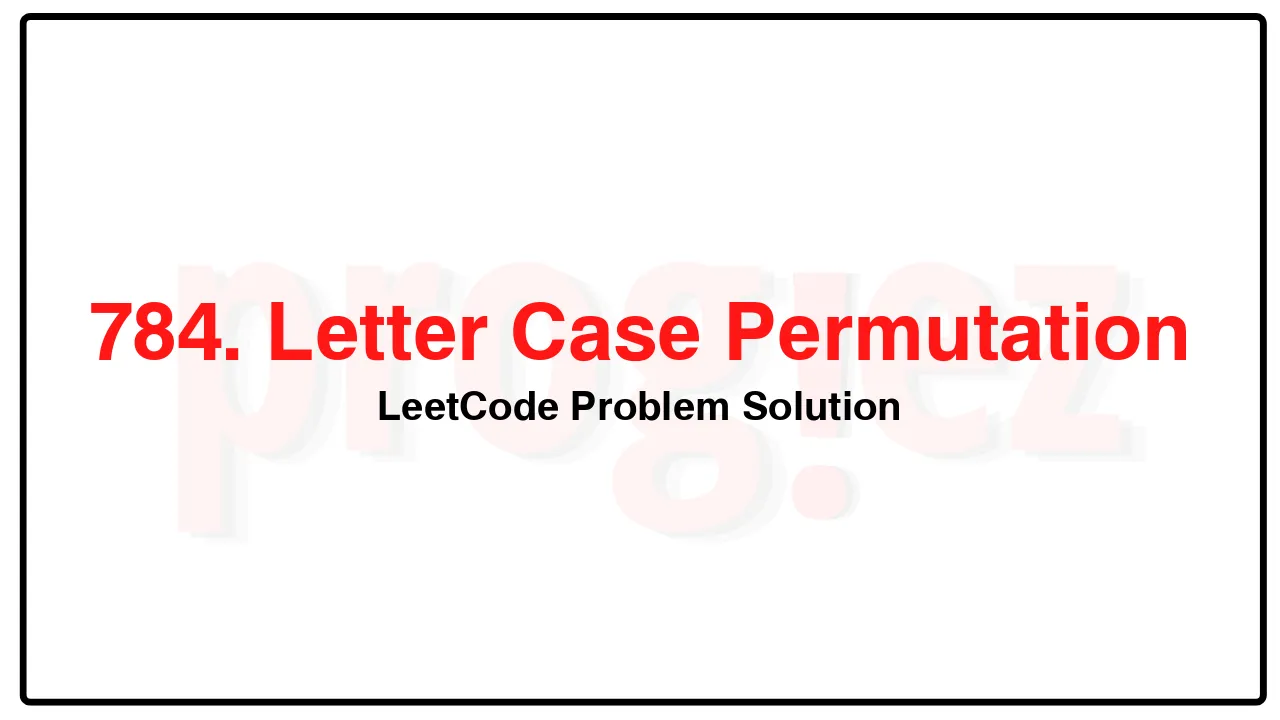
Problem Statement of Letter Case Permutation
Given a string s, you can transform every letter individually to be lowercase or uppercase to create another string.
Return a list of all possible strings we could create. Return the output in any order.
Example 1:
Input: s = “a1b2”
Output: [“a1b2″,”a1B2″,”A1b2″,”A1B2”]
Example 2:
Input: s = “3z4”
Output: [“3z4″,”3Z4”]
Constraints:
1 <= s.length <= 12
s consists of lowercase English letters, uppercase English letters, and digits.
Complexity Analysis
- Time Complexity: O(2^n)
- Space Complexity: O(2^n)
784. Letter Case Permutation LeetCode Solution in C++
class Solution {
public:
vector<string> letterCasePermutation(string s) {
vector<string> ans;
dfs(s, 0, ans);
return ans;
}
private:
void dfs(string& s, int i, vector<string>& ans) {
if (i == s.length()) {
ans.push_back(s);
return;
}
if (isdigit(s[i])) {
dfs(s, i + 1, ans);
return;
}
s[i] = tolower(s[i]);
dfs(s, i + 1, ans);
s[i] = toupper(s[i]);
dfs(s, i + 1, ans);
}
};
/* code provided by PROGIEZ */
784. Letter Case Permutation LeetCode Solution in Java
class Solution {
public List<String> letterCasePermutation(String s) {
List<String> ans = new ArrayList<>();
dfs(new StringBuilder(s), 0, ans);
return ans;
}
private void dfs(StringBuilder sb, int i, List<String> ans) {
if (i == sb.length()) {
ans.add(sb.toString());
return;
}
if (Character.isDigit(sb.charAt(i))) {
dfs(sb, i + 1, ans);
return;
}
sb.setCharAt(i, Character.toLowerCase(sb.charAt(i)));
dfs(sb, i + 1, ans);
sb.setCharAt(i, Character.toUpperCase(sb.charAt(i)));
dfs(sb, i + 1, ans);
}
}
// code provided by PROGIEZ
784. Letter Case Permutation LeetCode Solution in Python
N/A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.