64. Minimum Path Sum LeetCode Solution
In this guide, you will get 64. Minimum Path Sum LeetCode Solution with the best time and space complexity. The solution to Minimum Path Sum problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Path Sum solution in C++
- Minimum Path Sum solution in Java
- Minimum Path Sum solution in Python
- Additional Resources
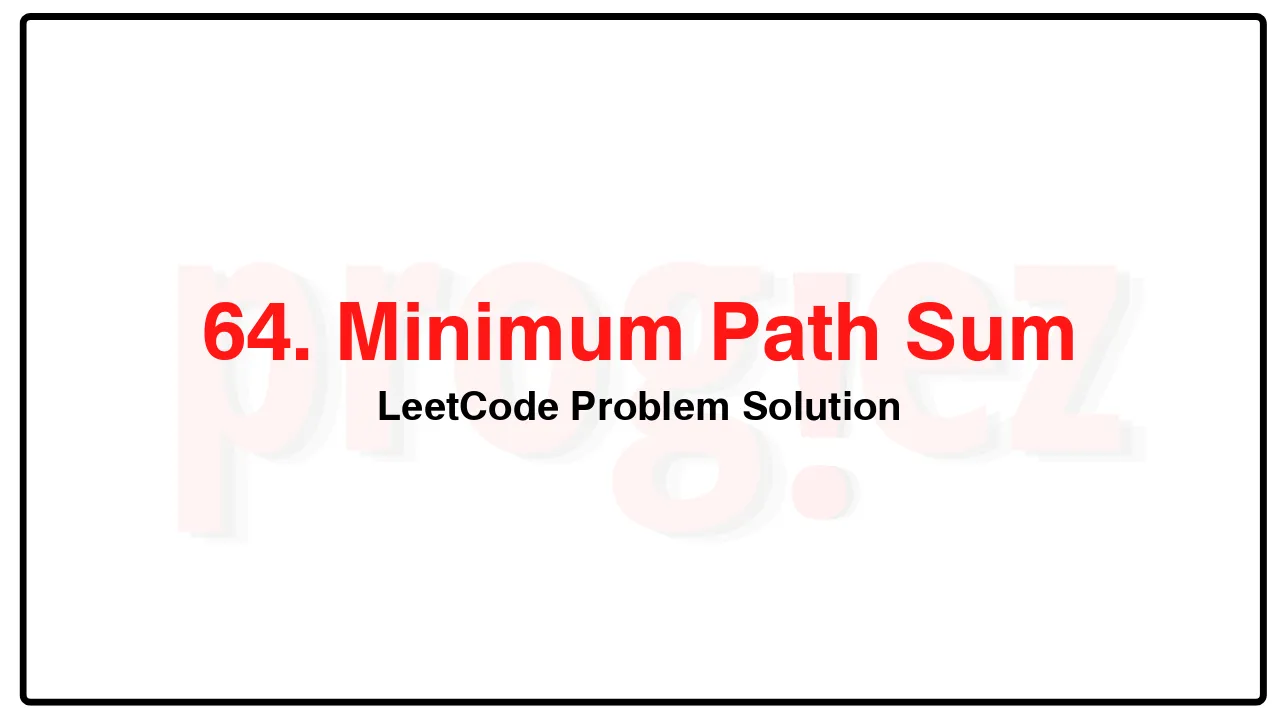
Problem Statement of Minimum Path Sum
Given a m x n grid filled with non-negative numbers, find a path from top left to bottom right, which minimizes the sum of all numbers along its path.
Note: You can only move either down or right at any point in time.
Example 1:
Input: grid = [[1,3,1],[1,5,1],[4,2,1]]
Output: 7
Explanation: Because the path 1 → 3 → 1 → 1 → 1 minimizes the sum.
Example 2:
Input: grid = [[1,2,3],[4,5,6]]
Output: 12
Constraints:
m == grid.length
n == grid[i].length
1 <= m, n <= 200
0 <= grid[i][j] <= 200
Complexity Analysis
- Time Complexity: O(mn)
- Space Complexity: O(1)
64. Minimum Path Sum LeetCode Solution in C++
class Solution {
public:
int minPathSum(vector<vector<int>>& grid) {
const int m = grid.size();
const int n = grid[0].size();
for (int i = 0; i < m; ++i)
for (int j = 0; j < n; ++j)
if (i > 0 && j > 0)
grid[i][j] += min(grid[i - 1][j], grid[i][j - 1]);
else if (i > 0)
grid[i][0] += grid[i - 1][0];
else if (j > 0)
grid[0][j] += grid[0][j - 1];
return grid[m - 1][n - 1];
}
};
/* code provided by PROGIEZ */
64. Minimum Path Sum LeetCode Solution in Java
class Solution {
public int minPathSum(int[][] grid) {
final int m = grid.length;
final int n = grid[0].length;
for (int i = 0; i < m; ++i)
for (int j = 0; j < n; ++j)
if (i > 0 && j > 0)
grid[i][j] += Math.min(grid[i - 1][j], grid[i][j - 1]);
else if (i > 0)
grid[i][0] += grid[i - 1][0];
else if (j > 0)
grid[0][j] += grid[0][j - 1];
return grid[m - 1][n - 1];
}
}
// code provided by PROGIEZ
64. Minimum Path Sum LeetCode Solution in Python
class Solution:
def minPathSum(self, grid: list[list[int]]) -> int:
m = len(grid)
n = len(grid[0])
for i in range(m):
for j in range(n):
if i > 0 and j > 0:
grid[i][j] += min(grid[i - 1][j], grid[i][j - 1])
elif i > 0:
grid[i][0] += grid[i - 1][0]
elif j > 0:
grid[0][j] += grid[0][j - 1]
return grid[m - 1][n - 1]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.