71. Simplify Path LeetCode Solution
In this guide, you will get 71. Simplify Path LeetCode Solution with the best time and space complexity. The solution to Simplify Path problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Simplify Path solution in C++
- Simplify Path solution in Java
- Simplify Path solution in Python
- Additional Resources
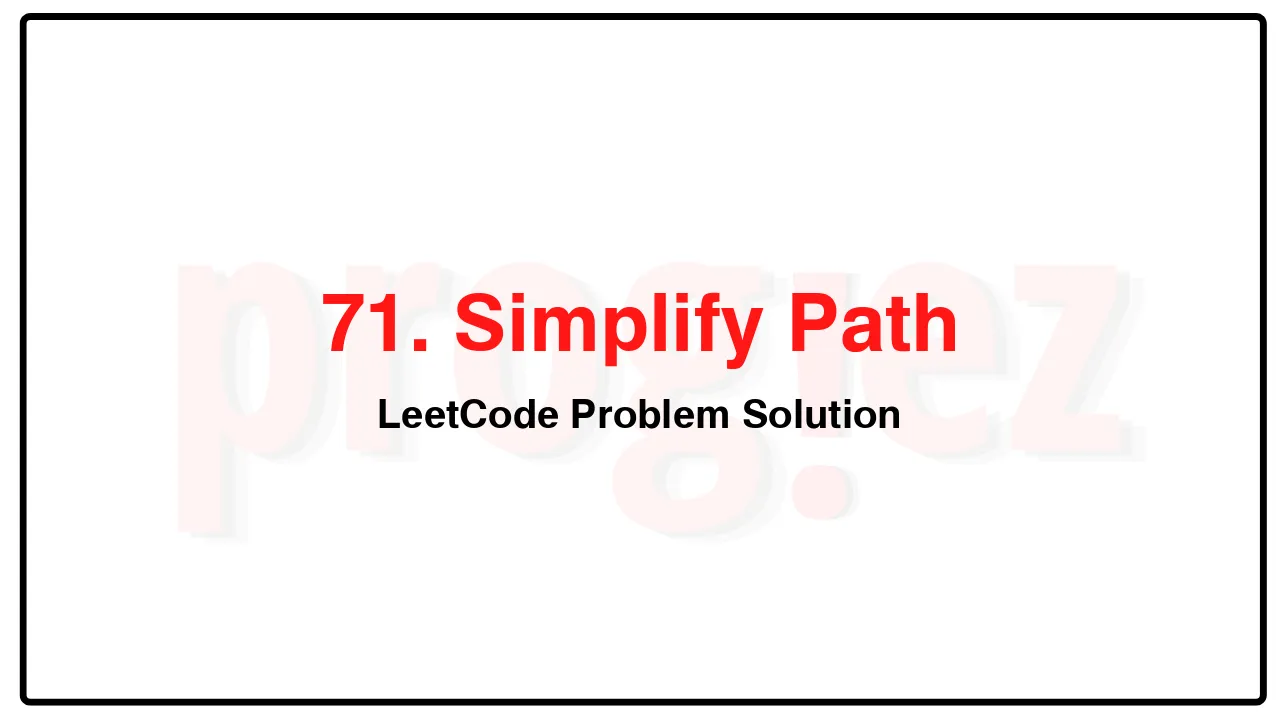
Problem Statement of Simplify Path
You are given an absolute path for a Unix-style file system, which always begins with a slash ‘/’. Your task is to transform this absolute path into its simplified canonical path.
The rules of a Unix-style file system are as follows:
A single period ‘.’ represents the current directory.
A double period ‘..’ represents the previous/parent directory.
Multiple consecutive slashes such as ‘//’ and ‘///’ are treated as a single slash ‘/’.
Any sequence of periods that does not match the rules above should be treated as a valid directory or file name. For example, ‘…’ and ‘….’ are valid directory or file names.
The simplified canonical path should follow these rules:
The path must start with a single slash ‘/’.
Directories within the path must be separated by exactly one slash ‘/’.
The path must not end with a slash ‘/’, unless it is the root directory.
The path must not have any single or double periods (‘.’ and ‘..’) used to denote current or parent directories.
Return the simplified canonical path.
Example 1:
Input: path = “/home/”
Output: “/home”
Explanation:
The trailing slash should be removed.
Example 2:
Input: path = “/home//foo/”
Output: “/home/foo”
Explanation:
Multiple consecutive slashes are replaced by a single one.
Example 3:
Input: path = “/home/user/Documents/../Pictures”
Output: “/home/user/Pictures”
Explanation:
A double period “..” refers to the directory up a level (the parent directory).
Example 4:
Input: path = “/../”
Output: “/”
Explanation:
Going one level up from the root directory is not possible.
Example 5:
Input: path = “/…/a/../b/c/../d/./”
Output: “/…/b/d”
Explanation:
“…” is a valid name for a directory in this problem.
Constraints:
1 <= path.length <= 3000
path consists of English letters, digits, period '.', slash '/' or '_'.
path is a valid absolute Unix path.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
71. Simplify Path LeetCode Solution in C++
class Solution {
public:
string simplifyPath(string path) {
string ans;
istringstream iss(path);
vector<string> stack;
for (string dir; getline(iss, dir, '/');) {
if (dir.empty() || dir == ".")
continue;
if (dir == "..") {
if (!stack.empty())
stack.pop_back();
} else {
stack.push_back(dir);
}
}
for (const string& s : stack)
ans += "/" + s;
return ans.empty() ? "/" : ans;
}
};
/* code provided by PROGIEZ */
71. Simplify Path LeetCode Solution in Java
class Solution {
public String simplifyPath(String path) {
final String[] dirs = path.split("/");
Stack<String> stack = new Stack<>();
for (final String dir : dirs) {
if (dir.isEmpty() || dir.equals("."))
continue;
if (dir.equals("..")) {
if (!stack.isEmpty())
stack.pop();
} else {
stack.push(dir);
}
}
return "/" + String.join("/", stack);
}
}
// code provided by PROGIEZ
71. Simplify Path LeetCode Solution in Python
class Solution:
def simplifyPath(self, path: str) -> str:
stack = []
for str in path.split('/'):
if str in ('', '.'):
continue
if str == '..':
if stack:
stack.pop()
else:
stack.append(str)
return '/' + '/'.join(stack)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.