520. Detect Capital LeetCode Solution
In this guide, you will get 520. Detect Capital LeetCode Solution with the best time and space complexity. The solution to Detect Capital problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Detect Capital solution in C++
- Detect Capital solution in Java
- Detect Capital solution in Python
- Additional Resources
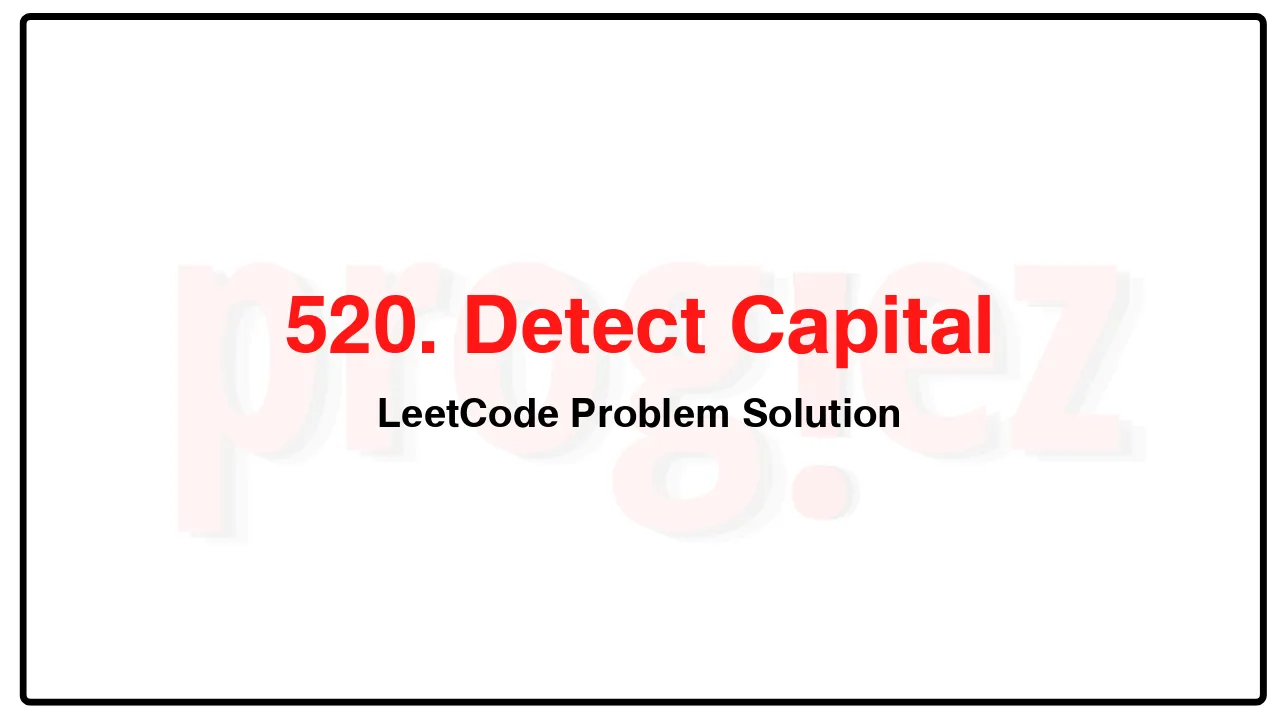
Problem Statement of Detect Capital
We define the usage of capitals in a word to be right when one of the following cases holds:
All letters in this word are capitals, like “USA”.
All letters in this word are not capitals, like “leetcode”.
Only the first letter in this word is capital, like “Google”.
Given a string word, return true if the usage of capitals in it is right.
Example 1:
Input: word = “USA”
Output: true
Example 2:
Input: word = “FlaG”
Output: false
Constraints:
1 <= word.length <= 100
word consists of lowercase and uppercase English letters.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
520. Detect Capital LeetCode Solution in C++
class Solution {
public:
bool detectCapitalUse(string word) {
for (int i = 1; i < word.length(); ++i)
if (isupper(word[1]) != isupper(word[i]) ||
islower(word[0]) && isupper(word[i]))
return false;
return true;
}
};
/* code provided by PROGIEZ */
520. Detect Capital LeetCode Solution in Java
class Solution {
public boolean detectCapitalUse(String word) {
return word.equals(word.toUpperCase()) ||
word.substring(1).equals(word.substring(1).toLowerCase());
}
}
// code provided by PROGIEZ
520. Detect Capital LeetCode Solution in Python
class Solution:
def detectCapitalUse(self, word: str) -> bool:
return word.isupper() or word.islower() or word.istitle()
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.