442. Find All Duplicates in an Array LeetCode Solution
In this guide, you will get 442. Find All Duplicates in an Array LeetCode Solution with the best time and space complexity. The solution to Find All Duplicates in an Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Find All Duplicates in an Array solution in C++
- Find All Duplicates in an Array solution in Java
- Find All Duplicates in an Array solution in Python
- Additional Resources
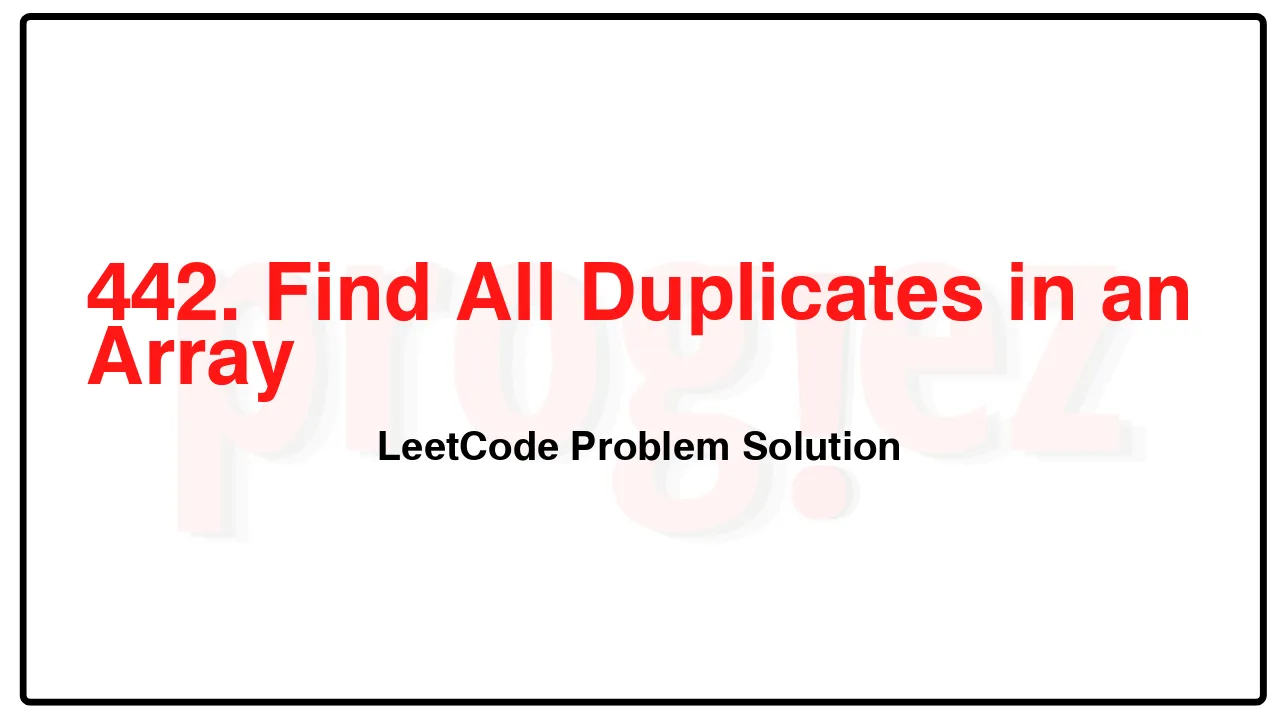
Problem Statement of Find All Duplicates in an Array
Given an integer array nums of length n where all the integers of nums are in the range [1, n] and each integer appears at most twice, return an array of all the integers that appears twice.
You must write an algorithm that runs in O(n) time and uses only constant auxiliary space, excluding the space needed to store the output
Example 1:
Input: nums = [4,3,2,7,8,2,3,1]
Output: [2,3]
Example 2:
Input: nums = [1,1,2]
Output: [1]
Example 3:
Input: nums = [1]
Output: []
Constraints:
n == nums.length
1 <= n <= 105
1 <= nums[i] <= n
Each element in nums appears once or twice.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
442. Find All Duplicates in an Array LeetCode Solution in C++
class Solution {
public:
vector<int> findDuplicates(vector<int>& nums) {
vector<int> ans;
for (const int num : nums) {
nums[abs(num) - 1] *= -1;
if (nums[abs(num) - 1] > 0)
ans.push_back(abs(num));
}
return ans;
}
};
/* code provided by PROGIEZ */
442. Find All Duplicates in an Array LeetCode Solution in Java
class Solution {
public List<Integer> findDuplicates(int[] nums) {
List<Integer> ans = new ArrayList<>();
for (final int num : nums) {
nums[Math.abs(num) - 1] *= -1;
if (nums[Math.abs(num) - 1] > 0)
ans.add(Math.abs(num));
}
return ans;
}
}
// code provided by PROGIEZ
442. Find All Duplicates in an Array LeetCode Solution in Python
class Solution:
def findDuplicates(self, nums: list[int]) -> list[int]:
ans = []
for num in nums:
nums[abs(num) - 1] *= -1
if nums[abs(num) - 1] > 0:
ans.append(abs(num))
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.