441. Arranging Coins LeetCode Solution
In this guide, you will get 441. Arranging Coins LeetCode Solution with the best time and space complexity. The solution to Arranging Coins problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Arranging Coins solution in C++
- Arranging Coins solution in Java
- Arranging Coins solution in Python
- Additional Resources
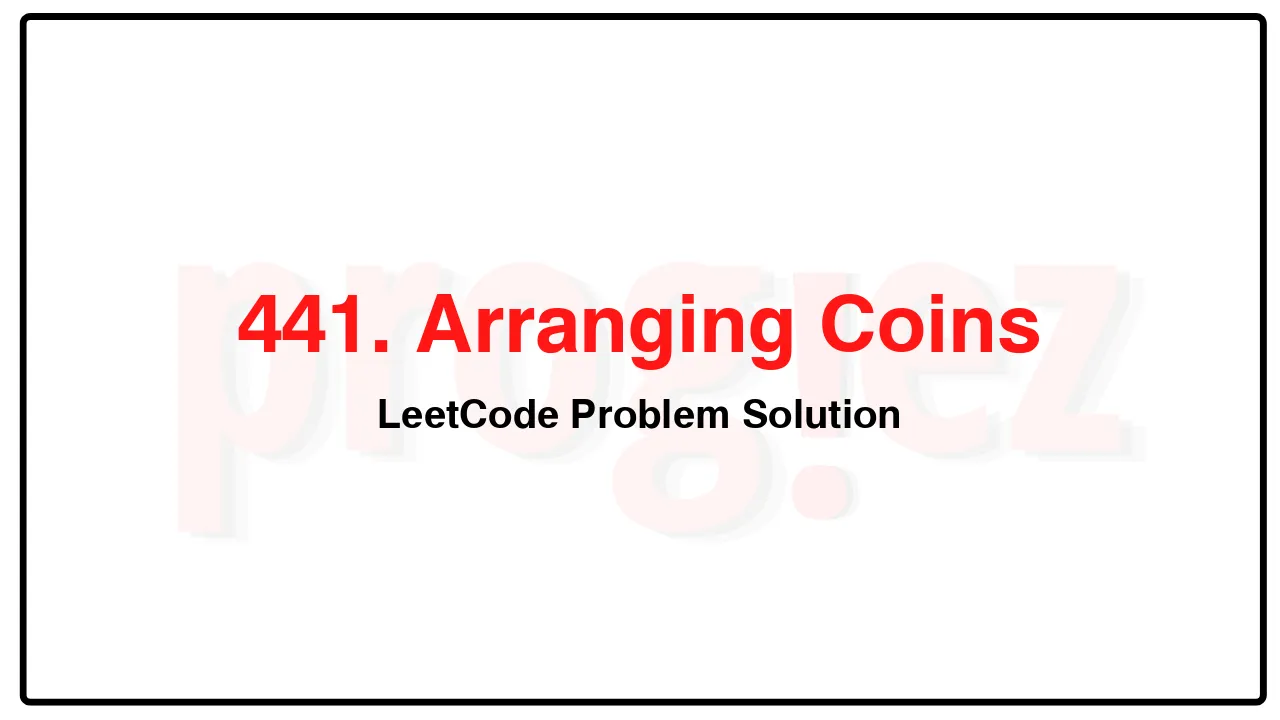
Problem Statement of Arranging Coins
You have n coins and you want to build a staircase with these coins. The staircase consists of k rows where the ith row has exactly i coins. The last row of the staircase may be incomplete.
Given the integer n, return the number of complete rows of the staircase you will build.
Example 1:
Input: n = 5
Output: 2
Explanation: Because the 3rd row is incomplete, we return 2.
Example 2:
Input: n = 8
Output: 3
Explanation: Because the 4th row is incomplete, we return 3.
Constraints:
1 <= n <= 231 – 1
Complexity Analysis
- Time Complexity: O(1)
- Space Complexity: O(1)
441. Arranging Coins LeetCode Solution in C++
class Solution {
public:
int arrangeCoins(long n) {
return (-1 + sqrt(8 * n + 1)) / 2;
}
};
/* code provided by PROGIEZ */
441. Arranging Coins LeetCode Solution in Java
class Solution {
public int arrangeCoins(long n) {
return (int) (-1 + Math.sqrt(8 * n + 1)) / 2;
}
}
// code provided by PROGIEZ
441. Arranging Coins LeetCode Solution in Python
class Solution:
def arrangeCoins(self, n: int) -> int:
return int((-1 + math.sqrt(8 * n + 1)) // 2)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.