405. Convert a Number to Hexadecimal LeetCode Solution
In this guide, you will get 405. Convert a Number to Hexadecimal LeetCode Solution with the best time and space complexity. The solution to Convert a Number to Hexadecimal problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Convert a Number to Hexadecimal solution in C++
- Convert a Number to Hexadecimal solution in Java
- Convert a Number to Hexadecimal solution in Python
- Additional Resources
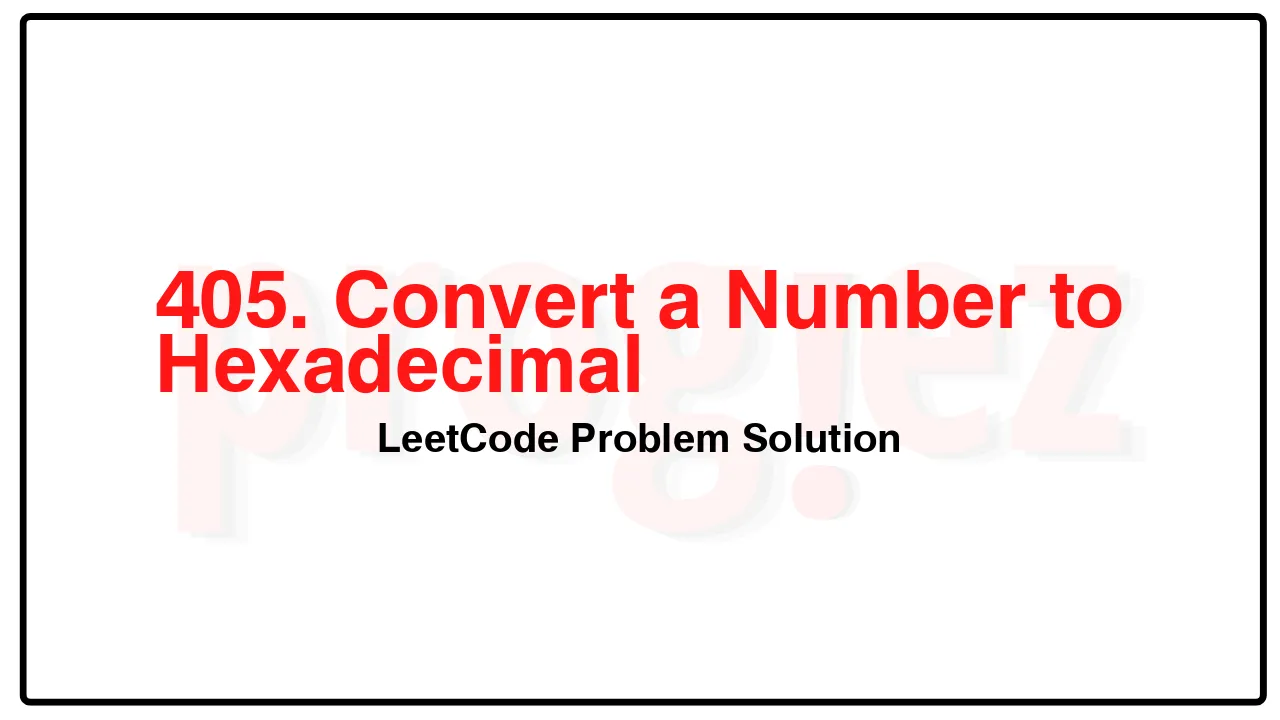
Problem Statement of Convert a Number to Hexadecimal
Given a 32-bit integer num, return a string representing its hexadecimal representation. For negative integers, two’s complement method is used.
All the letters in the answer string should be lowercase characters, and there should not be any leading zeros in the answer except for the zero itself.
Note: You are not allowed to use any built-in library method to directly solve this problem.
Example 1:
Input: num = 26
Output: “1a”
Example 2:
Input: num = -1
Output: “ffffffff”
Constraints:
-231 <= num <= 231 – 1
Complexity Analysis
- Time Complexity: O(\log_{16} n)
- Space Complexity: O(1)
405. Convert a Number to Hexadecimal LeetCode Solution in C++
class Solution {
public:
string toHex(unsigned num) {
if (num == 0)
return "0";
constexpr char hex[] = {'0', '1', '2', '3', '4', '5', '6', '7',
'8', '9', 'a', 'b', 'c', 'd', 'e', 'f'};
string ans;
while (num != 0) {
ans += hex[num & 0xf];
num >>= 4;
}
ranges::reverse(ans);
return ans;
}
};
/* code provided by PROGIEZ */
405. Convert a Number to Hexadecimal LeetCode Solution in Java
class Solution {
public String toHex(int num) {
if (num == 0)
return "0";
final char[] hex = {'0', '1', '2', '3', '4', '5', '6', '7',
'8', '9', 'a', 'b', 'c', 'd', 'e', 'f'};
StringBuilder sb = new StringBuilder();
while (num != 0) {
sb.append(hex[num & 0xf]);
num >>>= 4;
}
return sb.reverse().toString();
}
}
// code provided by PROGIEZ
405. Convert a Number to Hexadecimal LeetCode Solution in Python
class Solution:
def toHex(self, num: int) -> str:
if num == 0:
return '0'
hex = '0123456789abcdef'
ans = []
# Handling negative numbers by using 32-bit unsigned representation Python's
# bitwise operation works on signed numbers, so we convert to 32-bit
# unsigned for negative numbers.
if num < 0:
num += 2**32
while num > 0:
ans.append(hex[num & 0xF])
num >>= 4
return ''.join(reversed(ans))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.